
Small portion of code to verify correctly working hardware and connections
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 /* 00002 Initial prototyping to check hardware connections 00003 00004 Testing the following connections: 00005 00006 Joystick: 00007 xPot yPot button 00008 AnalogIn PTB11, AnalogIn PTB10, PTB9 00009 00010 Nokia 5110 lcd display: (Handled by library) 00011 VCC SCE RST D/C MOSI SCLK LED 00012 PTE26, PTA0, PTC4, PTD0, PTD2, PTD1, PTC3 00013 00014 buttonA buttonB g_led buzzer 00015 PTA1 PTB23 PWM PTC11 Undecided between AnalogOut DAC0_OUT or PWM PTC10 (decision will be based on sound quality) 00016 00017 01/03/16 00018 By Joel W. Webb 00019 Revision 1.0 00020 */ 00021 00022 #include "mbed.h" 00023 #include "N5110.h" 00024 00025 #define DIRECTION_TOLERANCE 0.05 00026 00027 // Nokia lcd object 00028 // VCC SCE RST D/C MOSI SCLK LED 00029 N5110 lcd(PTE26, PTA0, PTC4, PTD0, PTD2, PTD1, PTC3); 00030 00031 // Joystick 00032 AnalogIn xPot(PTB11); 00033 AnalogIn yPot(PTB10); 00034 DigitalIn button(PTB9); 00035 00036 enum DirectionName { 00037 UP, 00038 DOWN, 00039 LEFT, 00040 RIGHT, 00041 CENTRE, 00042 UNKNOWN 00043 }; 00044 00045 // struct for Joystick 00046 typedef struct JoyStick Joystick; 00047 struct JoyStick { 00048 float x; // current x value 00049 float x0; // 'centred' x value 00050 float y; // current y value 00051 float y0; // 'centred' y value 00052 int button; // button state (assume pull-down used, so 1 = pressed, 0 = unpressed) 00053 DirectionName direction; // current direction 00054 }; 00055 // create struct variable 00056 Joystick joystick; 00057 Ticker pollJoystick; // Ticker interrput for polling the joystick 00058 00059 // Other inputs/outputs 00060 InterruptIn buttonA(PTA1); 00061 InterruptIn buttonB(PTB23); 00062 PwmOut g_led(PTC11); 00063 DigitalOut r_led(LED1); // Testing led 00064 00065 //Global Flags defined here 00066 int g_joystick_flag; 00067 int g_buttonA_flag; 00068 int g_buttonB_flag; 00069 00070 // ISR defined here 00071 void buttonA_isr(){ 00072 g_buttonA_flag = 1; 00073 } 00074 void buttonB_isr(){ 00075 g_buttonB_flag = 1; 00076 } 00077 00078 // Functions defined here 00079 void initInputs(); 00080 void updateJoystick(); 00081 void calibrateJoystick(); 00082 00083 int main() 00084 { 00085 initInputs(); 00086 pollJoystick.attach(&updateJoystick,1.0/10.0); // read joystick 10 times per second 00087 00088 while (true) { 00089 00090 if (g_buttonA_flag){ 00091 g_buttonA_flag = 0; 00092 lcd.clear(); 00093 lcd.printString("Button A",0,0); 00094 } 00095 if (g_buttonB_flag){ 00096 g_buttonB_flag = 0; 00097 lcd.clear(); 00098 lcd.printString("Button B",0,1); 00099 } 00100 if (g_joystick_flag){ 00101 g_joystick_flag = 0; 00102 lcd.printString(" ",0,2); 00103 if (joystick.direction == 0){ lcd.printString("UP",0,2); } 00104 else if (joystick.direction == 1){ lcd.printString("DOWN",0,2); } 00105 else if (joystick.direction == 2){ lcd.printString("LEFT",0,2); } 00106 else if (joystick.direction == 3){ lcd.printString("RIGHT",0,2); } 00107 else if (joystick.direction == 4){ lcd.printString("CENTRE",0,2); } 00108 else if (joystick.direction == 5){ lcd.printString("UNDEFINED",0,2); } 00109 if (joystick.button){ lcd.printString("Button J",0,3); } 00110 } 00111 00112 lcd.refresh(); 00113 sleep(); 00114 00115 } 00116 } 00117 00118 00119 00120 void initInputs(){ 00121 00122 r_led = 1; 00123 // Initialising power LED 00124 g_led.period(0.001); // Frequency of 1kHz 00125 g_led = 0.25; // Duty cycle of 0.25 00126 00127 // Setting up Interrupt service routines for input buttons 00128 // Note no pull mode is specified so default pulldown resistors will be used 00129 buttonA.rise(&buttonA_isr); 00130 buttonB.rise(&buttonB_isr); 00131 buttonA.mode(PullDown); 00132 buttonB.mode(PullDown); 00133 00134 00135 //Testing LCD display works (splash screen) 00136 lcd.init(); 00137 wait(1.0); 00138 lcd.printString("Calibrating",0,0); 00139 lcd.printString("Do not move",0,1); 00140 lcd.printString("Joystick",0,2); 00141 lcd.refresh(); 00142 00143 calibrateJoystick(); 00144 00145 wait(1.0); 00146 lcd.clear(); 00147 00148 } 00149 00150 // read default positions of the joystick to calibrate later readings 00151 void calibrateJoystick() 00152 { 00153 button.mode(PullDown); 00154 // must not move during calibration 00155 joystick.x0 = xPot; // initial positions in the range 0.0 to 1.0 (0.5 if centred exactly) 00156 joystick.y0 = yPot; 00157 } 00158 void updateJoystick() 00159 { 00160 // read current joystick values relative to calibrated values (in range -0.5 to 0.5, 0.0 is centred) 00161 joystick.x = xPot - joystick.x0; 00162 joystick.y = yPot - joystick.y0; 00163 // read button state 00164 joystick.button = button; 00165 00166 // calculate direction depending on x,y values 00167 // tolerance allows a little lee-way in case joystick not exactly in the stated direction 00168 if ( fabs(joystick.y) < DIRECTION_TOLERANCE && fabs(joystick.x) < DIRECTION_TOLERANCE) { 00169 joystick.direction = CENTRE; 00170 } else if ( joystick.y > DIRECTION_TOLERANCE && fabs(joystick.x) < DIRECTION_TOLERANCE) { 00171 joystick.direction = UP; 00172 } else if ( joystick.y < DIRECTION_TOLERANCE && fabs(joystick.x) < DIRECTION_TOLERANCE) { 00173 joystick.direction = DOWN; 00174 } else if ( joystick.x > DIRECTION_TOLERANCE && fabs(joystick.y) < DIRECTION_TOLERANCE) { 00175 joystick.direction = RIGHT; 00176 } else if ( joystick.x < DIRECTION_TOLERANCE && fabs(joystick.y) < DIRECTION_TOLERANCE) { 00177 joystick.direction = LEFT; 00178 } else { 00179 joystick.direction = UNKNOWN; 00180 } 00181 00182 // set flag for printing 00183 g_joystick_flag = 1; 00184 } 00185
Generated on Thu Sep 28 2023 04:20:04 by
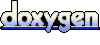