
A retro gaming programme, designed for use on a portable embedded system. Incorporates power saving techniques.
Dependencies: ConfigFile N5110 PowerControl beep mbed
main.cpp
00001 /** 00002 @file main.cpp 00003 @brief Program implementation 00004 @brief "Example code of how to read a joystick" courtesy of Craig A. Evans. Created: 7th March 2015. 00005 */ 00006 00007 #include "PowerControl/PowerControl.h"//for sleep modes 00008 #include "PowerControl/EthernetPowerControl.h"//for Ethernet Power Down 00009 #include "mbed.h" 00010 #include "N5110.h" 00011 #include "beep.h" 00012 #include "tower.h" 00013 #include <ctime> 00014 00015 ///writes to CFG file. 00016 void write() 00017 { 00018 ///Sets a Key 1 and Value 1. 00019 if (!cfg.setValue("Open1", player1initials )) {//key/value 00020 error("Failure to set a value.\n"); 00021 } 00022 ///Sets a Key 2 and Value 2. 00023 if (!cfg.setValue("Open2", player2initials )) {//key/value 00024 error("Failure to set a value.\n"); 00025 } 00026 ///Sets a Key 3 and Value 3. 00027 if (!cfg.setValue("Open3", player3initials )) {//key/value 00028 error("Failure to set a value.\n"); 00029 } 00030 ///If a file does not already exist, create one. 00031 ///If one already exits, append the file. 00032 if (!cfg.write("/local/towerMemory.cfg")) {//Write key and value to CFG file 00033 error("Failure to write a configuration file.\n"); 00034 } 00035 } 00036 00037 ///Reads CFG file. 00038 void read() 00039 { 00040 //Checks if key matches. 00041 char *key1 = "Open1"; 00042 char *key2 = "Open2"; 00043 char *key3 = "Open3"; 00044 00045 int count=0; 00046 00047 ///Checks Key for match, then reads Value - if read is unsuccessful it prints error message. 00048 if (!cfg.read("/local/towerMemory.cfg")) { 00049 error("Failure to read a configuration file.\n"); 00050 } 00051 ///check file size - if size acceptable, prints buffer to location. 00052 if (cfg.getValue(key1, &player1initials [0], sizeof(player1initials ))) { 00053 serial.printf("%s\n",player1initials ); 00054 00055 count=10;//first possible character of score 00056 highScore1 =0;//make sure that multiplacation works correctly 00057 while(1){ 00058 //if first iteration/not an empty score 00059 if(highScore1 !=0){ 00060 highScore1 *=10; 00061 } 00062 //convert ASCII to int value 00063 highScore1 +=player1initials [count]-48; 00064 //increment 00065 count++; 00066 //check to see if at end of string array 00067 if(player1initials [count]==NULL){ 00068 break; 00069 } 00070 } 00071 serial.printf("%i\n",highScore1 ); 00072 } 00073 //check file size - if size acceptable, prints buffer to location. 00074 if (cfg.getValue(key2, &player2initials [0], sizeof(player2initials ))) { 00075 serial.printf("%s\n",player2initials ); 00076 count=10; 00077 highScore2 =0; 00078 while(1){ 00079 if(highScore2 !=0){ 00080 highScore2 *=10; 00081 } 00082 highScore2 +=player2initials [count]-48; 00083 count++; 00084 if(player2initials [count]==NULL){ 00085 break; 00086 } 00087 } 00088 serial.printf("%i\n",highScore2 ); 00089 } 00090 //check file size - if size acceptable, prints buffer to location. 00091 if (cfg.getValue(key3, &player3initials [0], sizeof(player3initials ))) { 00092 serial.printf("%s\n",player3initials ); 00093 count=10; 00094 highScore3 =0; 00095 while(1){ 00096 if(highScore3 !=0){ 00097 highScore3 *=10; 00098 } 00099 highScore3 +=player3initials [count]-48; 00100 count++; 00101 if(player3initials [count]==NULL){ 00102 break; 00103 } 00104 } 00105 serial.printf("%i\n",highScore3 ); 00106 } 00107 } 00108 00109 ///Struct declaration for storing alphabet arrays. 00110 struct State { 00111 char output1; 00112 char output2; 00113 char output3; 00114 int nextState[2]; 00115 }; 00116 00117 ///Assigns new identifier to Struct. 00118 typedef const struct State STYP; 00119 00120 ///Finite State Machine for Struct data members. 00121 STYP fsm[27] = { 00122 00123 ///Outputs 1,2 and 3 for Initial Arrays. 00124 {'A','A','A',{1,26}}, 00125 {'B','B','B',{2,0}}, 00126 {'C','C','C',{3,1}}, 00127 {'D','D','D',{4,2}}, 00128 {'E','E','E',{5,3}}, 00129 {'F','F','F',{6,4}}, 00130 {'G','G','G',{7,5}}, 00131 {'H','H','H',{8,6}}, 00132 {'I','I','I',{9,7}}, 00133 {'J','J','J',{10,8}}, 00134 {'K','K','K',{11,9}}, 00135 {'L','L','L',{12,10}}, 00136 {'M','M','M',{13,11}}, 00137 {'N','N','N',{14,12}}, 00138 {'O','O','O',{15,13}}, 00139 {'P','P','P',{16,14}}, 00140 {'Q','Q','Q',{17,15}}, 00141 {'R','R','R',{18,16}}, 00142 {'S','S','S',{19,17}}, 00143 {'T','T','T',{20,18}}, 00144 {'U','U','U',{21,19}}, 00145 {'V','V','V',{22,20}}, 00146 {'W','W','W',{23,21}}, 00147 {'X','X','X',{24,22}}, 00148 {'Y','Y','Y',{25,23}}, 00149 {'Z','Z','Z',{26,24}}, 00150 {'.','.','.',{0,25}}, 00151 }; 00152 00153 ///Creates enumerated type (0,1,2,3 etc. for direction). 00154 enum DirectionName { 00155 UP, 00156 DOWN, 00157 LEFT, 00158 RIGHT, 00159 CENTRE, 00160 UNKNOWN 00161 }; 00162 00163 ///Struct for Joystick. 00164 typedef struct JoyStick Joystick; 00165 struct JoyStick { 00166 float x;//current x value 00167 float x0;//'centred' x value 00168 float y;//current y value 00169 float y0;//'centred' y value 00170 int button;//button state (assume pull-down used, so 1 = pressed, 0 = unpressed) 00171 DirectionName direction;//current direction 00172 }; 00173 00174 ///create struct variable 00175 Joystick joystick; 00176 00177 ///Called when timerA expires. If buttonA is pressed - set flag A. 00178 void timerExpiredA() 00179 { 00180 ///Includes backlight reset for user input. 00181 if((deBounce1.read_ms()>=95)&&(buttonA == 1)) { 00182 buttonFlagA = 1; 00183 lcd.setBrightness(1.0); 00184 serial.printf("flagA set\n"); 00185 deBounce1.reset(); 00186 ///Reset value for debounce timer 00187 } 00188 } 00189 00190 ///Called when timer expires. If buttonB is pressed - Set flag B. 00191 void timerExpiredB() 00192 { 00193 ///Includes backlight reset for user input. 00194 if((deBounce2.read_ms()>=95)&&(buttonB == 1)) { 00195 buttonFlagB = 1; 00196 lcd.setBrightness(1.0); 00197 serial.printf("flagB set\n"); 00198 deBounce2.reset();//reset timer 00199 ///Resets value for debounce timer 00200 } 00201 } 00202 00203 ///Randomises initial co-ordinates for falling hazards. 00204 void randomise() 00205 { 00206 ///Creates initial seed for randomisation. 00207 srand (time(NULL));//initial seed for randomisation 00208 00209 //initial random x co-ordinates 00210 //for falling hazards 00211 //(values between 3 and 76) 00212 randX1 = rand() % 74 + 5; 00213 randX2 = rand() % 74 + 5; 00214 randX3 = rand() % 74 + 5; 00215 randX4 = rand() % 74 + 5; 00216 randX5 = rand() % 74 + 5; 00217 randX6 = rand() % 74 + 5; 00218 } 00219 00220 ///Draws the games static background. 00221 void drawBackground() 00222 { 00223 //x, y, w, h, fill - draw ground 00224 lcd.drawRect(0,47,84,0,1); 00225 //x, y, w, h, fill - draw left wall 00226 lcd.drawRect(2,0,0,47,1); 00227 //left wall - brick line 1 00228 lcd.drawLine(1,1,1,48,2); 00229 //left wall - brick line 2 00230 lcd.drawLine(0,0,0,48,2); 00231 00232 //x, y, w, h, fill - draw right wall 00233 lcd.drawRect(81,0,0,47,1); 00234 //right wall - brick line 1 00235 lcd.drawLine(82,0,82,48,2); 00236 //right wall - brick line 2 00237 lcd.drawLine(83,1,83,48,2); 00238 00239 lcd.refresh(); 00240 } 00241 00242 ///Draws the Introduction screen. 00243 void drawWelcome() 00244 { 00245 //bottom border 00246 lcd.drawRect(0,44,84,2,1); 00247 //top border 00248 lcd.drawRect(0,1,84,2,1); 00249 lcd.refresh(); 00250 00251 //print initials 'DRT' 00252 lcd.printChar('D',30,2); 00253 wait(0.6); 00254 lcd.printChar('R',37,2); 00255 wait(0.6); 00256 lcd.printChar('T',44,2); 00257 wait(0.6); 00258 00259 //print 'presents...' 00260 lcd.printString("presents...",8,3); 00261 wait(1.0); 00262 00263 //dramatic flashing 00264 buzzer.beep(5000,0.3); 00265 lcd.inverseMode(); 00266 wait(0.2); 00267 lcd.normalMode(); 00268 wait(0.2); 00269 buzzer.beep(5000,0.3); 00270 lcd.inverseMode(); 00271 wait(0.2); 00272 lcd.normalMode(); 00273 wait(1.0); 00274 00275 //more dramatic flashing 00276 buzzer.beep(5000,0.3); 00277 lcd.inverseMode(); 00278 wait(0.2); 00279 lcd.normalMode(); 00280 wait(0.2); 00281 buzzer.beep(5000,0.3); 00282 lcd.inverseMode(); 00283 wait(0.2); 00284 lcd.normalMode(); 00285 wait(0.6); 00286 } 00287 00288 ///Draws Pixel Ninja. 00289 void drawNinja() 00290 { 00291 //x, y, w, h, fill - left leg 00292 lcd.drawRect(a1,39,0,7,1); 00293 //right leg 00294 lcd.drawRect(a2,39,0,7,1); 00295 //centre stick 00296 lcd.drawRect(a3,37,0,7,1); 00297 //back of the head 00298 lcd.drawRect(a4,33,0,4,1); 00299 //top of the head 00300 lcd.drawRect(a5,33,4,0,1); 00301 //jaw 00302 lcd.drawRect(a6,38,2,0,1); 00303 //right shoulder 00304 lcd.drawRect(a7,40,1,0,1); 00305 //left shoulder 00306 lcd.drawRect(a8,40,1,0,1); 00307 //left arm 00308 lcd.drawRect(a9,41,0,1,1); 00309 //right arm 00310 lcd.drawRect(a10,41,0,1,1); 00311 //right eye 00312 lcd.drawRect(a11,35,0,0,1); 00313 //mouth piece 00314 lcd.drawRect(a12,37,0,0,1); 00315 //left eye 00316 lcd.drawRect(a13,35,0,0,1); 00317 //sword handle 00318 lcd.drawRect(a14,36,0,0,1); 00319 lcd.drawRect(a15,37,0,0,1); 00320 lcd.drawRect(a16,38,0,0,1); 00321 00322 lcd.refresh(); 00323 } 00324 00325 ///Implements Pixel Ninja's boundary conditions. 00326 void ninjaBoundaries() 00327 { 00328 ///If ninja exceeds max boundary, Ninja equals max boundary. 00329 //right eye 00330 if(a11 > 80 ) 00331 a11 = 80; 00332 //mouth piece 00333 if(a12 > 80) 00334 a12 = 80; 00335 //right arm 00336 if(a10> 80) 00337 a10 = 80; 00338 //right shoulder 00339 if(a7 > 79) 00340 a7 = 79; 00341 //right leg 00342 if(a2 > 78) 00343 a2 = 78; 00344 //jaw 00345 if(a6 > 78) 00346 a6 = 78; 00347 //left eye 00348 if(a13 > 78) 00349 a13 = 78; 00350 //centre 00351 if(a3 > 77) 00352 a3 = 77; 00353 //back of the head 00354 if(a4 > 76 ) 00355 a4= 76; 00356 //topf of the head 00357 if(a5 > 76) 00358 a5 = 76; 00359 //left leg 00360 if(a1>76) 00361 a1 = 76; 00362 //sword 00363 if(a16>75) 00364 a16 = 75; 00365 //left shoulder 00366 if(a8 >74) 00367 a8 = 74; 00368 //left arm 00369 if(a9>74) 00370 a9 = 74; 00371 //sword 00372 if(a15>74) 00373 a15 = 74; 00374 //sword 00375 if(a14 > 73) 00376 a14 = 73; 00377 00378 //right eye 00379 if(a11 < 5 ) 00380 a11 = 5; 00381 //mouth piece 00382 if(a12 < 3) 00383 a12 = 3; 00384 //right arm 00385 if(a10< 9) 00386 a10 = 9; 00387 //right shoulder 00388 if(a7 < 8) 00389 a7 = 8; 00390 //right leg 00391 if(a2 < 7) 00392 a2 = 7; 00393 //jaw 00394 if(a6 < 3) 00395 a6 = 3; 00396 //left eye 00397 if(a13 < 3) 00398 a13 = 3; 00399 //centre 00400 if(a3 < 6) 00401 a3 = 6; 00402 //back of the head 00403 if(a4 < 7) 00404 a4= 7; 00405 //top of the head 00406 if(a5 < 3) 00407 a5 = 3; 00408 //left leg 00409 if(a1 < 5) 00410 a1 = 5; 00411 //sword 00412 if(a16 < 8) 00413 a16 = 8; 00414 //left shoulder 00415 if(a8 < 3) 00416 a8 = 3; 00417 //left arm 00418 if(a9 < 3) 00419 a9 = 3; 00420 //sword 00421 if(a15 < 9) 00422 a15 = 9; 00423 //sword 00424 if(a14 < 10) 00425 a14 = 10; 00426 ///If ninja falls below min boundary, Ninja equals min boundary. 00427 } 00428 00429 ///Resets configuration values for the game. 00430 void resetGame() 00431 { 00432 ///Resets Global variables for pixelNinja movement. 00433 a1 = 22; 00434 a2 = 24; 00435 a3 = 23; 00436 a4 = 22; 00437 a5 = 22; 00438 a6 = 24; 00439 a7 = 25; 00440 a8 = 20; 00441 a9 = 20; 00442 a10 = 26; 00443 a11 = 26; 00444 a12 = 26; 00445 a13 = 24; 00446 a14 = 19; 00447 a15 = 20; 00448 a16 = 21; 00449 00450 ///Resets current score. 00451 score = 0; 00452 00453 //in this case the X values are given a 00454 //new random variable each time the player 00455 //dies, exits or starts a new game 00456 00457 ///Randomises X co-ordinates. 00458 randomise(); 00459 00460 ///Resets Y co-ordinates. 00461 randY1 = 0; 00462 randY2 = 0; 00463 randY3 = 0; 00464 randY4 = 0; 00465 randY5 = 0; 00466 randY6 = 0; 00467 00468 ///Clears screen. 00469 lcd.clear(); 00470 } 00471 00472 ///Draws falling hazards. 00473 void drawHazards() 00474 { 00475 //X, Y, radius, fill 00476 lcd.drawCircle(randX1 ,randY1 ,2,1); 00477 lcd.drawCircle(randX2 ,randY2 ,2,1); 00478 lcd.drawCircle(randX3 ,randY3 ,2,1); 00479 lcd.drawCircle(randX4 ,randY4 ,2,1); 00480 lcd.drawCircle(randX5 ,randY5 ,2,1); 00481 lcd.drawCircle(randX6 ,randY6 ,2,1); 00482 00483 lcd.refresh(); 00484 } 00485 00486 ///Increments the hazards to make them "Fall". 00487 void hazardFall() 00488 { 00489 ///Increments values by chosen difficulty. 00490 randY1 = randY1 += fall ; 00491 randY2 = randY2 += fall ; 00492 randY3 = randY3 += fall ; 00493 randY4 = randY4 += fall ; 00494 randY5 = randY5 += fall ; 00495 randY6 = randY6 += fall ; 00496 00497 ///Loops objects once they've hit the floor. 00498 if (randY1 >=48) 00499 randY1 =0; 00500 00501 if (randY2 >=48) 00502 randY2 =0; 00503 00504 if (randY3 >=48) 00505 randY3 =0; 00506 00507 if (randY4 >=48) 00508 randY4 =0; 00509 00510 if (randY5 >=48) 00511 randY5 =0; 00512 00513 //each time the objects loop, a new pseudo random value 00514 //is assigned to the global variables (randX) to 00515 //randomise their positions 00516 00517 ///Assigns new random X co-ordinate once looped. 00518 if (randY6 >=48) { 00519 randY6 =0; 00520 ///Incements current score with each wave of hazards. 00521 score = score ++;//increment score by 1 after each wave of hazards 00522 randomise();//randomise x co-ordinates again 00523 } 00524 } 00525 00526 ///Clears old pixels and keeps set pixels. 00527 void startrek() 00528 { 00529 ///Loops thorugh screen pixel by pixel. 00530 for (int i=3; i<81; i++)//loops through rows 00531 for (int j=0; j<47; j++) 00532 if (cells [i][j]) {//if there's a pixel then keep it 00533 lcd.setPixel(i,j); 00534 } else { 00535 lcd.clearPixel(i,j);//else remove the old ones 00536 } 00537 lcd.refresh(); 00538 } 00539 00540 ///Clears old cursor, sets new one. 00541 void refreshCursor1() 00542 { 00543 ///Loops through select part of the screen. 00544 for (int i=70; i<80; i++)//loops through rows 00545 for (int j=17; j<25; j++) 00546 if (cells [i][j]) {//if there's a pixel then keep it 00547 lcd.setPixel(i,j); 00548 } else { 00549 lcd.clearPixel(i,j);//else remove the old ones 00550 } 00551 lcd.refresh(); 00552 } 00553 00554 ///Clears old cursor, sets new one. 00555 void refreshCursor2() 00556 { 00557 ///Loops though select part of the screen. 00558 for (int i=70; i<80; i++)//loops through rows 00559 for (int j=25; j<32; j++) 00560 if (cells [i][j]) {//if there's a pixel then keep it 00561 lcd.setPixel(i,j); 00562 } else { 00563 lcd.clearPixel(i,j);//else remove the old ones 00564 } 00565 lcd.refresh(); 00566 } 00567 00568 ///Clears old cursor, sets new one. 00569 void refreshCursor3() 00570 { 00571 ///Loops through select part of the screen. 00572 for (int i=70; i<80; i++)//loops through rows 00573 for (int j=32; j<40; j++) 00574 if (cells [i][j]) {//if there's a pixel then keep it 00575 lcd.setPixel(i,j); 00576 } else { 00577 lcd.clearPixel(i,j);//else remove the old ones 00578 } 00579 lcd.refresh(); 00580 } 00581 00582 ///Creates buzzer audible/LED to light when either button is pressed. 00583 void actionButtons() 00584 { 00585 ///If FX is on emit beep and LED flash, else just flash. 00586 if((FX == 0)&&(buttonA||buttonB)) { 00587 ledA = 1; 00588 buzzer.beep(1500,0.3); 00589 } 00590 if (buttonA || buttonB) { 00591 ledA = 1; 00592 } else { 00593 ledA = 0; 00594 } 00595 } 00596 00597 ///Implements Joystick navigation for main menu. 00598 void mainMenu(int& mainOption) 00599 { 00600 ///Includes actionButtons(); 00601 actionButtons();//set audible/light for button 00602 00603 if (printFlag ) {//if flag set, clear flag and print joystick values to serial port 00604 printFlag = 0; 00605 00606 //option up 00607 if (joystick.direction == UP) { 00608 serial.printf(" UP\n"); 00609 mainOption = mainOption--; 00610 if (mainOption < 0)mainOption = 0; 00611 } 00612 //option down 00613 if (joystick.direction == DOWN) { 00614 serial.printf(" DOWN\n"); 00615 mainOption = mainOption++; 00616 if (mainOption > 2)mainOption = 2; 00617 } 00618 //Centre / Unknown orientation 00619 if (joystick.direction == CENTRE) 00620 serial.printf(" CENTRE\n"); 00621 if (joystick.direction == UNKNOWN) 00622 serial.printf(" Unsupported direction\n"); 00623 00624 //'Play Game' option 1 00625 if (mainOption == 0) { 00626 lcd.printString("Play Game",3,4); 00627 } 00628 //'High Scores' option 2 00629 if (mainOption == 1) { 00630 lcd.printString(" Scores ",3,4); 00631 } 00632 //'Options' option 3 00633 if (mainOption == 2) { 00634 lcd.printString(" Options ",3,4); 00635 } 00636 } 00637 } 00638 00639 ///Draws the Main Menu. 00640 void drawMainMenu() 00641 { 00642 //bottom border 00643 lcd.drawRect(0,47,84,0,1); 00644 //top border 00645 lcd.drawRect(0,0,84,2,1); 00646 00647 //title outline 00648 lcd.drawRect(3,6,77,10,0); 00649 00650 ////castle //x, y, w, h, fill////////////////////// 00651 00652 //castle main bulk 00653 lcd.drawRect(59,32,21,8,1); 00654 00655 //left window bulk 00656 lcd.drawRect(59,22,2,10,1); 00657 //centre left window bulk 00658 lcd.drawRect(65,22,2,10,1); 00659 //centre right window bulk 00660 lcd.drawRect(72,22,2,10,1); 00661 //right window bulk 00662 lcd.drawRect(78,22,2,10,1); 00663 //central window bulk 00664 lcd.drawRect(68,25,3,7,1); 00665 00666 //central window bulk 00667 lcd.drawRect(75,28,0,0,1); 00668 lcd.drawRect(77,28,0,0,1); 00669 lcd.drawRect(64,28,0,0,1); 00670 lcd.drawRect(62,28,0,0,1); 00671 00672 //above left window bulk 00673 lcd.drawRect(62,25,3,2,1); 00674 //above right window bulk 00675 lcd.drawRect(75,25,2,2,1); 00676 00677 //lower right line 00678 lcd.drawRect(71,42,9,0,1); 00679 //upper right line 00680 lcd.drawRect(70,41,10,0,1); 00681 00682 //upper left line 00683 lcd.drawRect(59,41,10,0,1); 00684 //lower left line 00685 lcd.drawRect(59,42,9,0,1); 00686 00687 //bottom left bulk 00688 lcd.drawRect(59,43,8,3,1); 00689 //bottom right bulk 00690 lcd.drawRect(72,43,8,3,1); 00691 00692 //option arrows - lower 00693 lcd.drawRect(27,42,4,0,1); 00694 lcd.drawRect(28,43,2,0,1); 00695 lcd.drawRect(29,44,0,0,1); 00696 00697 //option arrows - higher 00698 lcd.drawRect(27,29,4,0,1); 00699 lcd.drawRect(28,28,2,0,1); 00700 lcd.drawRect(29,27,0,0,1); 00701 00702 //print 'Xtreme Tower' 00703 lcd.printString("Xtreme Tower",7,1); 00704 00705 lcd.refresh(); 00706 } 00707 00708 ///Implements Joystick navigation for Exit Menu. 00709 void exitMenu(int& exitOption) 00710 { 00711 ///Includes actionButtons(); 00712 actionButtons(); 00713 if (printFlag ) {//if flag set, clear flag and print joystick values to serial port 00714 printFlag = 0; 00715 00716 if (joystick.direction == LEFT) { 00717 serial.printf(" LEFT\n"); 00718 exitOption--; 00719 if(exitOption < 0)exitOption = 0; 00720 } 00721 if (joystick.direction == RIGHT) { 00722 serial.printf(" RIGHT\n"); 00723 exitOption++; 00724 if(exitOption > 1)exitOption = 1; 00725 } 00726 if (joystick.direction == CENTRE) 00727 serial.printf(" CENTRE\n"); 00728 if (joystick.direction == UNKNOWN) 00729 serial.printf(" Unsupported direction\n"); 00730 } 00731 //draws option cursor 00732 if(exitOption == 0) { 00733 lcd.printString("YES",33,3); 00734 } else if(exitOption == 1) { 00735 lcd.printString(" NO",33,3); 00736 } 00737 } 00738 00739 ///If the joystick is moved left, Pixel Ninja moves left. 00740 void ninjaLeft() 00741 { 00742 ///Decrements values for left movement. 00743 a1 = a1-=2; 00744 a2 = a2-=2; 00745 a3 = a3-=2; 00746 a4 = a3+1; 00747 a5 = a3-3; 00748 a6 = a3-3; 00749 a7 = a7-=2; 00750 a8 = a8-=2; 00751 a9 = a9-=2; 00752 a10 = a10-=2; 00753 a11 = a3-1; 00754 a12 = a3-3; 00755 a13 = a3-3; 00756 a14 = a3+4; 00757 a15 = a3+3; 00758 a16 = a3+2; 00759 ///Also turns head to face left. 00760 } 00761 00762 ///If the Joystick is moved right, Pixel Ninja moves right. 00763 void ninjaRight() 00764 { 00765 ///Increments for right movement. 00766 a1 = a1+=2; 00767 a2 = a2+=2; 00768 a3 = a3+=2; 00769 a4 = a3-1; 00770 a5 = a3-1; 00771 a6 = a3+1; 00772 a7 = a7+=2; 00773 a8 = a8+=2; 00774 a9 = a9+=2; 00775 a10 = a10+=2; 00776 a11 = a3+3; 00777 a12 = a3+3; 00778 a13 = a3+1; 00779 a14 = a3-4; 00780 a15 = a3-3; 00781 a16 = a3-2; 00782 ///Turns head to face right. 00783 } 00784 00785 ///Draws the Exit Menu. 00786 void drawExitMenu() 00787 { 00788 //set exit menu 00789 lcd.clear(); 00790 drawBackground(); 00791 lcd.printString("Exit Game?",14,1); 00792 00793 lcd.drawRect(8,3,70,30,0);//title outline 00794 //option arrow - right 00795 lcd.drawRect(55,25,0,4,1); 00796 lcd.drawRect(56,26,0,2,1); 00797 lcd.drawRect(57,27,0,0,1); 00798 00799 //option arrow - left// 00800 lcd.drawRect(27,25,0,4,1); 00801 lcd.drawRect(26,26,0,2,1); 00802 lcd.drawRect(25,27,0,0,1); 00803 00804 lcd.refresh(); 00805 } 00806 00807 ///Implements Joystick navigation for Options Menu. 00808 void optionsMenu(int& option) 00809 { 00810 //joystick selection 00811 if (printFlag ) { //if flag set, clear flag and print joystick values to serial port 00812 printFlag = 0; 00813 00814 //option up 00815 if (joystick.direction == UP) { 00816 serial.printf(" UP\n"); 00817 option = option--; 00818 if (option < 0)option = 0; 00819 } 00820 //option down 00821 if (joystick.direction == DOWN) { 00822 serial.printf(" DOWN\n"); 00823 option = option++; 00824 if (option > 1)option = 1; 00825 } 00826 //Centre / Unknown orientation 00827 if (joystick.direction == CENTRE) 00828 serial.printf(" CENTRE\n"); 00829 if (joystick.direction == UNKNOWN) 00830 serial.printf(" Unsupported direction\n"); 00831 00832 //'Difficulty' option 1 00833 if (option == 0) { 00834 lcd.drawCircle(72,27,2,1); 00835 refreshCursor3(); 00836 } 00837 //'Sound FX' option 2 00838 if (option == 1) { 00839 lcd.drawCircle(72,35,2,1); 00840 refreshCursor2(); 00841 } 00842 } 00843 lcd.refresh(); 00844 } 00845 00846 ///Draws the Options Menu. 00847 void drawOptionsMenu() 00848 { 00849 lcd.clear();//clear screen 00850 drawBackground(); 00851 lcd.drawRect(3,6,77,10,0);//title outline 00852 lcd.drawRect(0,47,84,0,1);//bottom border 00853 lcd.drawRect(0,0,84,2,1);//top border 00854 lcd.printString("Options",20,1);//title 00855 lcd.printString("Difficulty",5,3); 00856 lcd.printString("Sound FX",5,4); 00857 00858 lcd.refresh(); 00859 } 00860 00861 ///Implements Joystick navigation for Difficulty Options. 00862 void difficultyMenu(int& subOption) 00863 { 00864 ///Includes actionButtons(); 00865 actionButtons(); 00866 00867 //joystick selection 00868 if (printFlag ) {//if flag set, clear flag,print joystick values 00869 printFlag = 0; 00870 00871 //option up 00872 if (joystick.direction == UP) { 00873 serial.printf(" UP\n"); 00874 subOption = subOption--; 00875 if (subOption < 1)subOption = 1; 00876 } 00877 //option down 00878 if (joystick.direction == DOWN) { 00879 serial.printf(" DOWN\n"); 00880 subOption = subOption++; 00881 if (subOption > 3)subOption = 3; 00882 } 00883 //Centre / Unknown orientation 00884 if (joystick.direction == CENTRE) 00885 serial.printf(" CENTRE\n"); 00886 if (joystick.direction == UNKNOWN) 00887 serial.printf(" Unsupported direction\n"); 00888 00889 //'Easy' option 1 00890 if (subOption == 1) { 00891 lcd.drawCircle(72,19,2,1); 00892 refreshCursor2(); 00893 refreshCursor3(); 00894 if(buttonFlagA ) { //select easy 00895 //rightness(1.0); 00896 buttonFlagA = 0; 00897 fall = 1; 00898 } 00899 } 00900 //'Normal' option 2 00901 if (subOption == 2) { 00902 lcd.drawCircle(72,27,2,1); 00903 refreshCursor1(); 00904 refreshCursor3(); 00905 00906 if(buttonFlagA ) { //select normal 00907 //rightness(1.0); 00908 buttonFlagA = 0; 00909 fall = 2; 00910 } 00911 } 00912 //'Forget It' option 3 00913 if (subOption == 3) { 00914 lcd.drawCircle(72,35,2,1); 00915 refreshCursor1(); 00916 refreshCursor2(); 00917 00918 if(buttonFlagA ) { //select difficult 00919 //rightness(1.0); 00920 buttonFlagA = 0; 00921 fall = 3; 00922 } 00923 } 00924 } 00925 lcd.refresh(); 00926 } 00927 00928 ///Draws Difficulty Menu. 00929 void drawDifficultyMenu() 00930 { 00931 lcd.clear(); 00932 drawBackground(); 00933 lcd.drawRect(0,47,84,0,1);//bottom border 00934 lcd.drawRect(0,0,84,2,1);//top border 00935 lcd.refresh(); 00936 lcd.printString("*Difficulty*",5,1);//title 00937 lcd.printString("Easy",5,2);//title 00938 lcd.printString("Normal",5,3);//title 00939 lcd.printString("Forget It",5,4);//title 00940 } 00941 00942 ///Implements Joystick navigation for FX Menu. 00943 void soundFXMenu(int& fxOption) 00944 { 00945 ///Inlcudes actionButtons(); 00946 actionButtons(); 00947 00948 //joystick selection 00949 if (printFlag ) {//if flag set, clear flag,print joystick values 00950 printFlag = 0; 00951 00952 //option up 00953 if (joystick.direction == UP) { 00954 serial.printf(" UP\n"); 00955 fxOption = fxOption--; 00956 if (fxOption < 0)fxOption = 0; 00957 } 00958 //option down 00959 if (joystick.direction == DOWN) { 00960 serial.printf(" DOWN\n"); 00961 fxOption = fxOption++; 00962 if (fxOption > 1)fxOption = 1; 00963 } 00964 //Centre / Unknown orientation 00965 if (joystick.direction == CENTRE) 00966 serial.printf(" CENTRE\n"); 00967 if (joystick.direction == UNKNOWN) 00968 serial.printf(" Unsupported direction\n"); 00969 } 00970 //'ON' option 1 00971 if (fxOption == 0) { 00972 lcd.drawCircle(72,27,2,1);//draw cursor 'ON' 00973 refreshCursor1(); 00974 refreshCursor3(); 00975 00976 if(buttonFlagA ) { 00977 buttonFlagA =0; 00978 FX = 0; 00979 serial.printf("FX = %d\n",FX ); 00980 } 00981 } 00982 //'OFF' option 2 00983 if (fxOption == 1) { 00984 lcd.drawCircle(72,35,2,1);//draw cursor 'OFF' 00985 refreshCursor1(); 00986 refreshCursor2(); 00987 00988 if(buttonFlagA ) { 00989 buttonFlagA =0; 00990 FX = 1; 00991 serial.printf("FX = %d\n",FX ); 00992 } 00993 } 00994 lcd.refresh(); 00995 } 00996 00997 ///Draws the FX Menu. 00998 void drawSoundFXMenu() 00999 { 01000 lcd.clear(); 01001 drawBackground(); 01002 lcd.drawRect(0,47,84,0,1);//bottom border 01003 lcd.drawRect(0,0,84,2,1);//top border 01004 lcd.printString("*Sound FX*",10,1);//title 01005 lcd.printString("ON",35,3);//title 01006 lcd.printString("OFF",33,4);//title 01007 lcd.refresh(); 01008 } 01009 01010 01011 ///Game flag for main game. 01012 void gameLoop() 01013 { 01014 ///Sets gameFlag when game Timer expires. 01015 gameFlag = 1; 01016 } 01017 01018 ///Shifts and stores the new scores accordingly. 01019 void newScore() 01020 { 01021 ///Entry conditions mean function is only used if user equals or beats High Score 3. 01022 if(score >= highScore3 ) {//entry condition 01023 buttonFlagA = 0;//reset flags 01024 buttonFlagB = 0; 01025 lcd.clear();//clears screen 01026 drawBackground();//draws background 01027 lcd.printString("High Score!!",7,0);//title 01028 lcd.printString("Enter ID",19,4);//title 01029 01030 int n;//local variable used for storing temporary global variable 01031 int initial = 0;//used for isolating which initial is being selected 01032 char x,y,z; 01033 01034 //print initial characters 01035 x=fsm[state1 ].output1; 01036 lcd.printChar(x,28,2); 01037 y=fsm[state2 ].output2; 01038 lcd.printChar(y,40,2); 01039 z=fsm[state3 ].output3; 01040 lcd.printChar(z,52,2); 01041 01042 while(1) { 01043 01044 //joystick selection 01045 if (printFlag ) {//if flag set, clear flag,print joystick values 01046 printFlag = 0; 01047 01048 if(joystick.direction==CENTRE) { 01049 preDirection =0; 01050 } 01051 if (joystick.direction == UP ) { 01052 serial.printf(" UP\n"); 01053 state1 = state1 --; 01054 if (state1 < 0)state1 = 0; 01055 state2 = state2 --; 01056 if (state2 < 0)state2 = 0; 01057 state3 = state3 --; 01058 if (state3 < 0)state3 = 0; 01059 } 01060 //option down 01061 if (joystick.direction == DOWN ) { 01062 serial.printf(" DOWN\n"); 01063 state1 = state1 ++; 01064 if (state1 > 26)state1 = 26; 01065 state2 = state2 ++; 01066 if (state2 > 26)state2 = 26; 01067 state3 = state3 ++; 01068 if (state3 > 26)state3 = 26; 01069 } 01070 if (joystick.direction == LEFT && preDirection ==0) { 01071 serial.printf(" LEFT\n"); 01072 initial = initial--; 01073 if (initial < 0)initial = 0; 01074 preDirection =1; 01075 } 01076 if (joystick.direction == RIGHT && preDirection ==0) { 01077 serial.printf(" RIGHT\n"); 01078 initial = initial++; 01079 if (initial > 2)initial = 2; 01080 preDirection =1; 01081 } 01082 //Centre / Unknown orientation 01083 if (joystick.direction == CENTRE) 01084 serial.printf(" CENTRE\n"); 01085 if (joystick.direction == UNKNOWN) 01086 serial.printf(" Unsupported direction\n"); 01087 } 01088 //if initial 3 display selected character 01089 if (initial == 0) { 01090 x=fsm[state1 ].output1; 01091 lcd.printChar(x,28,2); 01092 } 01093 //if initial 1 display selected character 01094 if(initial == 1) { 01095 y=fsm[state2 ].output2; 01096 lcd.printChar(y,40,2); 01097 } 01098 //if initial 2 display selected character 01099 if(initial == 2) { 01100 z=fsm[state3 ].output3; 01101 lcd.printChar(z,52,2); 01102 } 01103 if(buttonFlagA ) { 01104 actionButtons(); 01105 buttonFlagA = 0; 01106 buttonFlagB = 0; 01107 break; 01108 } 01109 } 01110 //if player beats High Score 3, replace it with new score 01111 if(score >= highScore3 && score <highScore2 ) { 01112 highScore3 = score ; 01113 sprintf (player3initials , "3.%c%c%c.....%i",x,y,z,highScore3 ); 01114 } 01115 //if player beats High Score 3 and 2, replace HighScore2 with new score 01116 if(score >= highScore2 && score < highScore1 ) { 01117 highScore3 = highScore2 ; 01118 n = score ; 01119 highScore2 = n; 01120 sprintf (player3initials , "3.%c%c%c.....%i",player2initials [2],player2initials [3],player2initials [4],highScore3 ); 01121 sprintf (player2initials , "2.%c%c%c.....%i",x,y,z,highScore2 ); 01122 } 01123 //if player beats High Score 1, 2 and 3, replace highScore1 with new score 01124 if(score >= highScore1 ) { 01125 highScore3 = highScore2 ; 01126 highScore2 = highScore1 ; 01127 n = score ; 01128 highScore1 = n; 01129 sprintf (player3initials , "3.%c%c%c.....%i",player2initials [2],player2initials [3],player2initials [4],highScore3 ); 01130 sprintf (player2initials , "2.%c%c%c.....%i",player1initials [2],player1initials [3],player1initials [4],highScore2 ); 01131 sprintf (player1initials , "1.%c%c%c.....%i",x,y,z,highScore1 ); 01132 } 01133 state1 = 0; 01134 state2 = 0; 01135 state3 = 0; 01136 write(); 01137 } 01138 } 01139 01140 ///Begins Game. 01141 void game(int& exitFlag, int& exitOption) 01142 { 01143 ///Includes action buttons. 01144 actionButtons(); 01145 lcd.clear();//clears screen 01146 drawBackground();//draw background 01147 01148 while(1) { 01149 01150 //print score - top left of display 01151 char buffer [14];//create buffer for string 01152 int length = sprintf(buffer,"Level:%d",score );//insert buffer 01153 lcd.printString(buffer,3,0);//display 01154 01155 actionButtons(); 01156 drawNinja();//set character 01157 drawHazards();//initiates hazards 01158 01159 if(gameFlag ) { 01160 gameFlag = 0; 01161 hazardFall();//increments hazards towards floor 01162 if (printFlag ) { //if flag set, clear flag and print joystick values to serial port 01163 printFlag = 0; 01164 if (joystick.direction == RIGHT) { 01165 serial.printf(" RIGHT\n"); 01166 ninjaRight();//move right 01167 ninjaBoundaries(); 01168 } 01169 //check joystick direction 01170 if (joystick.direction == LEFT) { 01171 serial.printf(" LEFT\n"); 01172 ninjaLeft();//move left 01173 ninjaBoundaries(); 01174 } 01175 if (joystick.direction == CENTRE) 01176 serial.printf(" CENTRE\n"); 01177 if (joystick.direction == UNKNOWN) 01178 serial.printf(" Unsupported direction\n"); 01179 01180 //integer to represent character being 01181 //struck by falling object 01182 int contactPoint = 0; 01183 01184 //contact points 01185 if(lcd.getPixel((a3),32)) 01186 contactPoint++; 01187 if(lcd.getPixel((a3+3),32)) 01188 contactPoint++; 01189 if(lcd.getPixel((a3-3),32)) 01190 contactPoint++; 01191 01192 //if contact point is not zero 01193 //character has been hit 01194 //and the game ends 01195 if ( contactPoint !=0) { 01196 lcd.printString("Game Over",17,2); 01197 lcd.inverseMode(); 01198 buzzer.beep(2000,0.2);//frequeny/duration 01199 wait(0.5); 01200 lcd.normalMode(); 01201 wait(0.5); 01202 lcd.inverseMode(); 01203 buzzer.beep(2000,0.2); 01204 wait(0.5); 01205 lcd.normalMode(); 01206 wait(0.5); 01207 lcd.inverseMode(); 01208 buzzer.beep(2000,0.2); 01209 wait(0.5); 01210 lcd.normalMode(); 01211 newScore();//enter initial screen if previous scores are beaten 01212 //write(); 01213 resetGame();//reset values 01214 break; 01215 } 01216 lcd.refresh();//refresh screen 01217 startrek();//clears unset pixels, keeps set pixels 01218 01219 //Exit Menu (Back button pressed)// 01220 if(buttonFlagB ) { 01221 buttonFlagB = 0;//reset flags 01222 buttonFlagA = 0; 01223 actionButtons(); 01224 drawExitMenu();//draws the exit menu 01225 01226 while(1) { 01227 exitMenu(exitOption);//presents exit options 01228 01229 //'exit' option YES 01230 if((buttonFlagA )&&(exitOption == 0)) { //returns to menu 01231 //rightness(1.0); 01232 exitOption = 1;//reset intial option value 01233 buttonFlagA = 0;//reset flags 01234 buttonFlagB = 0; 01235 actionButtons(); 01236 lcd.clear();//clears screen 01237 resetGame();//resets scores/objects 01238 exitFlag = 1;//sets exit flag 01239 break; 01240 } 01241 //'exit' option NO - returns to game 01242 if((buttonFlagA )&&(exitOption == 1)) { 01243 buttonFlagA = 0;//resets flags 01244 buttonFlagB = 0; 01245 break; 01246 } 01247 sleep();//put while to sleep 01248 } 01249 //if 'exit' option YES, resets 01250 //game values returns to main menu 01251 if (exitFlag!=0) { //if exit flag set 01252 exitFlag = 0;//reset flag 01253 break;//break to main menu 01254 } 01255 } 01256 serial.printf("Score: %i \n",score );//print Score for debug 01257 01258 } 01259 } 01260 } 01261 } 01262 01263 ///Draws Scores Menu. 01264 void scores() 01265 { 01266 ///Include actionButtons(); 01267 actionButtons(); 01268 lcd.clear();//clear screen 01269 drawBackground();//set background 01270 lcd.printString("High Scores",10,0);//title 01271 01272 //prints scores with names 01273 lcd.printString(player1initials ,5,2);//display 01274 lcd.printString(player2initials ,5,3);//display 01275 lcd.printString(player3initials ,5,4);//display 01276 01277 while(1) { 01278 actionButtons();//select 01279 01280 //back to menu 01281 if(buttonFlagB ) { 01282 buttonFlagA = 0;//reset flags 01283 buttonFlagB = 0; 01284 lcd.clear(); 01285 break; 01286 } 01287 sleep();//put while to sleep 01288 } 01289 } 01290 01291 ///Implements selection for Options Menu. 01292 void optionsMenu() 01293 { 01294 ///Includes actionButtons(); 01295 int option = 0; 01296 int subOption = fall ;//keeps cursor on selected option, even after exiting screen 01297 int fxOption = 0; 01298 actionButtons(); 01299 drawOptionsMenu();//draws options menu 01300 //counters for navigation 01301 01302 while(1) { 01303 actionButtons(); 01304 optionsMenu(option);//presents options 01305 01306 //================ Difficulty menu ======================= 01307 if ((option == 0)&&(buttonFlagA )) { 01308 //rightness(1.0); 01309 buttonFlagA = 0;//reset flag 01310 actionButtons(); 01311 drawDifficultyMenu();//draws difficulty menu 01312 01313 while(1) { 01314 actionButtons(); 01315 difficultyMenu(subOption);//presents difficulty options 01316 01317 if(buttonFlagB ) { 01318 buttonFlagB = 0;//reset flags 01319 buttonFlagA = 0; 01320 lcd.clear();//clear screen 01321 break;//return back 01322 } 01323 sleep();//put while to sleep 01324 } 01325 drawOptionsMenu(); 01326 } 01327 //================sound FX menu================================== 01328 01329 if((option ==1)&&(buttonFlagA )) { 01330 buttonFlagA = 0;//reset flags 01331 buttonFlagB = 0; 01332 actionButtons(); 01333 drawSoundFXMenu();//draw menu 01334 01335 while(1) { 01336 actionButtons(); 01337 soundFXMenu(fxOption);//presents options 01338 01339 //back to options menu 01340 if(buttonFlagB ) { 01341 buttonFlagB = 0;//reset flags 01342 buttonFlagA = 0; 01343 lcd.clear();//clear screen 01344 break;//return back 01345 } 01346 sleep();//put while to sleep 01347 } 01348 drawOptionsMenu(); 01349 } 01350 //back to mainmenu 01351 if(buttonFlagB ) { 01352 buttonFlagB = 0;//reset flags 01353 buttonFlagA = 0; 01354 lcd.clear();//clear 01355 break;//return back 01356 } 01357 sleep();//put while to sleep 01358 } 01359 } 01360 01361 ///Reads default position of Joystick for calibration. 01362 void calibrateJoystick() 01363 { 01364 joyButton.mode(PullDown); 01365 //must not move during calibration 01366 joystick.x0 = xPot; //initial positions in the range 0.0 to 1.0 (0.5 if centred exactly) 01367 joystick.y0 = yPot; 01368 } 01369 01370 ///Updates Joytick position according to voltage readings. 01371 void updateJoystick() 01372 { 01373 //read current joystick values relative to calibrated values (in range -0.5 to 0.5, 0.0 is centred) 01374 joystick.x = xPot - joystick.x0; 01375 joystick.y = yPot - joystick.y0; 01376 //read button state 01377 joystick.button = joyButton; 01378 01379 //calculate direction depending on x,y values 01380 //tolerance allows a little lee-way in case joystick not exactly in the stated direction 01381 if ( fabs(joystick.y) < DIRECTION_TOLERANCE && fabs(joystick.x) < DIRECTION_TOLERANCE) { 01382 joystick.direction = CENTRE; 01383 } else if ( joystick.y > DIRECTION_TOLERANCE && fabs(joystick.x) < DIRECTION_TOLERANCE) { 01384 joystick.direction = DOWN; 01385 lcd.setBrightness(1.0); 01386 } else if ( joystick.y < DIRECTION_TOLERANCE && fabs(joystick.x) < DIRECTION_TOLERANCE) { 01387 joystick.direction = UP; 01388 lcd.setBrightness(1.0); 01389 } else if ( joystick.x > DIRECTION_TOLERANCE && fabs(joystick.y) < DIRECTION_TOLERANCE) { 01390 joystick.direction = RIGHT; 01391 lcd.setBrightness(1.0); 01392 } else if ( joystick.x < DIRECTION_TOLERANCE && fabs(joystick.y) < DIRECTION_TOLERANCE) { 01393 joystick.direction = LEFT; 01394 lcd.setBrightness(1.0); 01395 } else { 01396 joystick.direction = UNKNOWN; 01397 } 01398 //set flag for printing 01399 printFlag = 1; 01400 } 01401 01402 ///Turns off screen backlighting. 01403 void screenOff() 01404 { 01405 ///Sets lcd birghtness to 0.0. 01406 lcd.setBrightness(0.0); 01407 } 01408 01409 ///When idle, calls the funtion screenOff(); 01410 void idleMode() 01411 { 01412 ///If user input detected, reset timer. 01413 if((buttonFlagA )||(buttonFlagB )||(joystick.direction)) { //call standby mode if idle 01414 int time = 60; 01415 standby.attach(&screenOff,time); 01416 } 01417 } 01418 01419 int main() 01420 { 01421 PHY_PowerDown();//powers down the Ethernet 01422 ledP = 1;//power LED on 01423 randomise();//randomises falling hazards (initial values only) 01424 calibrateJoystick();//get centred values of joystick 01425 pollJoystick.attach(&updateJoystick,1.0/10.0);//read joystick 10 times per second 01426 01427 lcd.init();//initialise screen 01428 drawWelcome();//welcome screen 01429 lcd.clear();//clear screen 01430 01431 buttonA.mode(PullDown);//pull down buttonA 01432 buttonB.mode(PullDown);//pull down buttonB 01433 01434 int exitFlag = 0;//exit flag 01435 int mainOption = 0;//counter for main menu 01436 int exitOption = 1;//counter for exit menu 01437 01438 read();//set high scores using flash memory 01439 deBounce1.start();//timer buttonA debounce 01440 deBounce2.start();//timer buttonB debounce 01441 timerGame.attach(&gameLoop,0.1);//timer game delay 01442 timerA.attach(&timerExpiredA, 0.1);//checks state of buttonA 01443 timerB.attach(&timerExpiredB, 0.1);//checks state of buttonB 01444 idleMode();//turns off screen if idle. 01445 01446 while(1) { 01447 drawMainMenu();//draws main menu 01448 mainMenu(mainOption);//presents main menu options 01449 actionButtons();//sound light when buttons pressed 01450 01451 // if 'Play Game' selected 01452 if ((mainOption == 0)&&(buttonFlagA )) { 01453 buttonFlagA = 0; 01454 buttonFlagB = 0; 01455 game(exitFlag, exitOption);//actual game 01456 } 01457 // if 'Scores' selected 01458 if((mainOption == 1)&&(buttonFlagA )) { 01459 buttonFlagA = 0; 01460 buttonFlagB = 0; 01461 scores(); 01462 } 01463 // if 'option' selected 01464 if((mainOption == 2)&&(buttonFlagA )) { 01465 buttonFlagA = 0; 01466 buttonFlagB = 0; 01467 optionsMenu(); 01468 } 01469 sleep(); 01470 } 01471 }
Generated on Tue Jul 19 2022 02:31:56 by
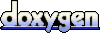