Works with interrupts
Dependents: Nucleo_UltrasonicHelloWorld Proj Nucleo_UltrasonicHelloWorld mbedTurtleCar ... more
ultrasonic.h
00001 #ifndef MBED_ULTRASONIC_H 00002 #define MBED_ULTRASONIC_H 00003 00004 #include "mbed.h" 00005 00006 class ultrasonic 00007 { 00008 public: 00009 /**iniates the class with the specified trigger pin, echo pin, update speed and timeout**/ 00010 ultrasonic(PinName trigPin, PinName echoPin, float updateSpeed, float timeout); 00011 /**iniates the class with the specified trigger pin, echo pin, update speed, timeout and method to call when the distance changes**/ 00012 ultrasonic(PinName trigPin, PinName echoPin, float updateSpeed, float timeout, void onUpdate(int)); 00013 /** returns the last measured distance**/ 00014 int getCurrentDistance(void); 00015 /**pauses measuring the distance**/ 00016 void pauseUpdates(void); 00017 /**starts mesuring the distance**/ 00018 void startUpdates(void); 00019 /**attachs the method to be called when the distances changes**/ 00020 void attachOnUpdate(void method(int)); 00021 /**changes the speed at which updates are made**/ 00022 void changeUpdateSpeed(float updateSpeed); 00023 /**gets whether the distance has been changed since the last call of isUpdated() or checkDistance()**/ 00024 int isUpdated(void); 00025 /**gets the speed at which updates are made**/ 00026 float getUpdateSpeed(void); 00027 /**call this as often as possible in your code, eg. at the end of a while(1) loop, 00028 and it will check whether the method you have attached needs to be called**/ 00029 void checkDistance(void); 00030 private: 00031 DigitalOut _trig; 00032 InterruptIn _echo; 00033 Timer _t; 00034 Timeout _tout; 00035 int _distance; 00036 float _updateSpeed; 00037 int start; 00038 int end; 00039 volatile int done; 00040 void (*_onUpdateMethod)(int); 00041 void _startT(void); 00042 void _updateDist(void); 00043 void _startTrig(void); 00044 float _timeout; 00045 int d; 00046 }; 00047 #endif
Generated on Tue Jul 12 2022 12:36:21 by
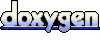