
Pong for Gamepad2
Embed:
(wiki syntax)
Show/hide line numbers
Paddle.cpp
00001 #include "Paddle.h" 00002 00003 // nothing doing in the constructor and destructor 00004 Paddle::Paddle() 00005 { 00006 00007 } 00008 00009 Paddle::~Paddle() 00010 { 00011 00012 } 00013 00014 void Paddle::init(int x,int height,int width) 00015 { 00016 _x = x; // x value on screen is fixed 00017 _y = HEIGHT/2 - height/2; // y depends on height of screen and height of paddle 00018 _height = height; 00019 _width = width; 00020 _speed = 1; // default speed 00021 _score = 0; // start score from zero 00022 00023 } 00024 00025 void Paddle::draw(N5110 &lcd) 00026 { 00027 // draw paddle in screen buffer. 00028 lcd.drawRect(_x,_y,_width,_height,FILL_BLACK); 00029 } 00030 00031 void Paddle::update(Direction d,float mag) 00032 { 00033 _speed = int(mag*10.0f); // scale is arbitrary, could be changed in future 00034 00035 // update y value depending on direction of movement 00036 // North is decrement as origin is at the top-left so decreasing moves up 00037 if (d == N) { 00038 _y-=_speed; 00039 } else if (d == S) { 00040 _y+=_speed; 00041 } 00042 00043 // check the y origin to ensure that the paddle doesn't go off screen 00044 if (_y < 1) { 00045 _y = 1; 00046 } 00047 if (_y > HEIGHT - _height - 1) { 00048 _y = HEIGHT - _height - 1; 00049 } 00050 } 00051 00052 void Paddle::add_score() 00053 { 00054 _score++; 00055 } 00056 int Paddle::get_score() 00057 { 00058 return _score; 00059 } 00060 00061 Vector2D Paddle::get_pos() { 00062 Vector2D p = {_x,_y}; 00063 return p; 00064 }
Generated on Sat Jul 16 2022 18:01:16 by
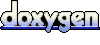