
Pong for Gamepad2
Embed:
(wiki syntax)
Show/hide line numbers
N5110.h
00001 #ifndef N5110_H 00002 #define N5110_H 00003 00004 #include "mbed.h" 00005 00006 // number of pixels on display 00007 #define WIDTH 84 00008 #define HEIGHT 48 00009 #define BANKS 6 00010 00011 /// Fill types for 2D shapes 00012 enum FillType { 00013 FILL_TRANSPARENT, ///< Transparent with outline 00014 FILL_BLACK, ///< Filled black 00015 FILL_WHITE, ///< Filled white (no outline) 00016 }; 00017 00018 /** N5110 Class 00019 @brief Library for interfacing with Nokia 5110 LCD display (https://www.sparkfun.com/products/10168) using the hardware SPI on the mbed. 00020 @brief The display is powered from a GPIO pin meaning it can be controlled via software. The LED backlight is also software-controllable (via PWM pin). 00021 @brief Can print characters and strings to the display using the included 5x7 font. 00022 @brief The library also implements a screen buffer so that individual pixels on the display (84 x 48) can be set, cleared and read. 00023 @brief The library can print primitive shapes (lines, circles, rectangles) 00024 @brief Acknowledgements to Chris Yan's Nokia_5110 Library. 00025 00026 @brief Revision 1.3 00027 00028 @author Craig A. Evans 00029 @date 7th February 2017 00030 00031 @code 00032 00033 #include "mbed.h" 00034 #include "N5110.h" 00035 00036 // rows,cols 00037 int sprite[8][5] = { 00038 { 0,0,1,0,0 }, 00039 { 0,1,1,1,0 }, 00040 { 0,0,1,0,0 }, 00041 { 0,1,1,1,0 }, 00042 { 1,1,1,1,1 }, 00043 { 1,1,1,1,1 }, 00044 { 1,1,0,1,1 }, 00045 { 1,1,0,1,1 }, 00046 }; 00047 00048 // VCC,SCE,RST,D/C,MOSI,SCLK,LED 00049 //N5110 lcd(p7,p8,p9,p10,p11,p13,p21); // LPC1768 - pwr from GPIO 00050 N5110 lcd(p8,p9,p10,p11,p13,p21); // LPC1768 - powered from +3V3 - JP1 in 2/3 position 00051 //N5110 lcd(PTC9,PTC0,PTC7,PTD2,PTD1,PTC11); // K64F - pwr from 3V3 00052 00053 int main() 00054 { 00055 // first need to initialise display 00056 lcd.init(); 00057 00058 // change set contrast in range 0.0 to 1.0 00059 // 0.4 appears to be a good starting point 00060 lcd.setContrast(0.4); 00061 00062 while(1) { 00063 00064 // these are default settings so not strictly needed 00065 lcd.normalMode(); // normal colour mode 00066 00067 lcd.clear(); 00068 // x origin, y origin, rows, cols, sprite 00069 lcd.drawSprite(20,6,8,5,(int *)sprite); 00070 lcd.refresh(); 00071 wait(5.0); 00072 00073 lcd.clear(); // clear buffer at start of every loop 00074 // can directly print strings at specified co-ordinates (must be less than 84 pixels to fit on display) 00075 lcd.printString("Hello, World!",0,0); 00076 00077 char buffer[14]; // each character is 6 pixels wide, screen is 84 pixels (84/6 = 14) 00078 // so can display a string of a maximum 14 characters in length 00079 // or create formatted strings - ensure they aren't more than 14 characters long 00080 int temperature = 27; 00081 int length = sprintf(buffer,"T = %2d C",temperature); // print formatted data to buffer 00082 // it is important the format specifier ensures the length will fit in the buffer 00083 if (length <= 14) // if string will fit on display (assuming printing at x=0) 00084 lcd.printString(buffer,0,1); // display on screen 00085 00086 float pressure = 1012.3; // same idea with floats 00087 length = sprintf(buffer,"P = %.2f mb",pressure); 00088 if (length <= 14) 00089 lcd.printString(buffer,0,2); 00090 00091 // can also print individual characters at specified place 00092 lcd.printChar('X',5,3); 00093 00094 // draw a line across the display at y = 40 pixels (origin top-left) 00095 for (int i = 0; i < WIDTH; i++) { 00096 lcd.setPixel(i,40,true); 00097 } 00098 // need to refresh display after setting pixels or writing strings 00099 lcd.refresh(); 00100 wait(5.0); 00101 00102 // can check status of pixel using getPixel(x,y); 00103 lcd.clear(); // clear buffer 00104 lcd.setPixel(2,2,true); // set random pixel in buffer 00105 lcd.refresh(); 00106 wait(1.0); 00107 00108 int pixel_to_test = lcd.getPixel(2,2); 00109 00110 if ( pixel_to_test ) { 00111 lcd.printString("2,2 is set",0,4); 00112 } 00113 00114 // this one shouldn't be set 00115 lcd.setPixel(3,3,false); // clear random pixel in buffer 00116 lcd.refresh(); 00117 pixel_to_test = lcd.getPixel(3,3); 00118 00119 if ( pixel_to_test == 0 ) { 00120 lcd.printString("3,3 is clear",0,5); 00121 } 00122 00123 lcd.refresh(); 00124 wait(4.0); 00125 00126 lcd.clear(); // clear buffer 00127 lcd.inverseMode(); // invert colours 00128 lcd.setBrightness(1.0); // put LED backlight on full 00129 00130 float array[84]; 00131 00132 for (int i = 0; i < 84; i++) { 00133 array[i] = 0.5 + 0.5*sin(i*2*3.14/84); 00134 } 00135 00136 // can also plot graphs - 84 elements only 00137 // values must be in range 0.0 - 1.0 00138 lcd.plotArray(array); 00139 lcd.refresh(); 00140 wait(5.0); 00141 00142 lcd.clear(); 00143 lcd.normalMode(); // normal colour mode back 00144 lcd.setBrightness(0.5); // put LED backlight on 50% 00145 00146 // example of drawing lines 00147 for (int x = 0; x < WIDTH ; x+=10) { 00148 // x0,y0,x1,y1,type 0-white,1-black,2-dotted 00149 lcd.drawLine(0,0,x,HEIGHT,2); 00150 } 00151 lcd.refresh(); // refresh after drawing shapes 00152 wait(5.0); 00153 00154 00155 lcd.clear(); 00156 // example of how to draw circles 00157 lcd.drawCircle(WIDTH/2,HEIGHT/2,20,FILL_BLACK); // x,y,radius,black fill 00158 lcd.drawCircle(WIDTH/2,HEIGHT/2,10,FILL_WHITE); // x,y,radius,white fill 00159 lcd.drawCircle(WIDTH/2,HEIGHT/2,30,FILL_TRANSPARENT); // x,y,radius,transparent with outline 00160 lcd.refresh(); // refresh after drawing shapes 00161 wait(5.0); 00162 00163 lcd.clear(); 00164 // example of how to draw rectangles 00165 // origin x,y,width,height,type 00166 lcd.drawRect(10,10,50,30,FILL_BLACK); // filled black rectangle 00167 lcd.drawRect(15,15,20,10,FILL_WHITE); // filled white rectange (no outline) 00168 lcd.drawRect(2,2,70,40,FILL_TRANSPARENT); // transparent, just outline 00169 lcd.refresh(); // refresh after drawing shapes 00170 wait(5.0); 00171 00172 } 00173 } 00174 00175 00176 @endcode 00177 */ 00178 class N5110 00179 { 00180 private: 00181 // objects 00182 SPI *_spi; 00183 DigitalOut *_led; 00184 DigitalOut *_pwr; 00185 DigitalOut *_sce; 00186 DigitalOut *_rst; 00187 DigitalOut *_dc; 00188 00189 // variables 00190 unsigned char buffer[84][6]; // screen buffer - the 6 is for the banks - each one is 8 bits; 00191 00192 public: 00193 /** Create a N5110 object connected to the specified pins 00194 * 00195 * @param pwr Pin connected to Vcc on the LCD display (pin 1) 00196 * @param sce Pin connected to chip enable (pin 3) 00197 * @param rst Pin connected to reset (pin 4) 00198 * @param dc Pin connected to data/command select (pin 5) 00199 * @param mosi Pin connected to data input (MOSI) (pin 6) 00200 * @param sclk Pin connected to serial clock (SCLK) (pin 7) 00201 * @param led Pin connected to LED backlight (must be PWM) (pin 8) 00202 * 00203 */ 00204 N5110(PinName const pwrPin, 00205 PinName const scePin, 00206 PinName const rstPin, 00207 PinName const dcPin, 00208 PinName const mosiPin, 00209 PinName const sclkPin, 00210 PinName const ledPin); 00211 00212 /** Create a N5110 object connected to the specified pins (Vcc to +3V3) 00213 * 00214 * @param sce Pin connected to chip enable (pin 3) 00215 * @param rst Pin connected to reset (pin 4) 00216 * @param dc Pin connected to data/command select (pin 5) 00217 * @param mosi Pin connected to data input (MOSI) (pin 6) 00218 * @param sclk Pin connected to serial clock (SCLK) (pin 7) 00219 * @param led Pin connected to LED backlight (must be PWM) (pin 8) 00220 * 00221 */ 00222 N5110(PinName const scePin, 00223 PinName const rstPin, 00224 PinName const dcPin, 00225 PinName const mosiPin, 00226 PinName const sclkPin, 00227 PinName const ledPin); 00228 00229 00230 /** Creates a N5110 object with the New Gamepad (Rev 2.1) pin mapping 00231 */ 00232 N5110(); 00233 00234 /** 00235 * Free allocated memory when object goes out of scope 00236 */ 00237 ~N5110(); 00238 00239 /** Initialise display 00240 * 00241 * Powers up the display and turns on backlight (50% brightness default). 00242 * Sets the display up in horizontal addressing mode and with normal video mode. 00243 */ 00244 void init(); 00245 00246 /** Turn off 00247 * 00248 * Powers down the display and turns of the backlight. 00249 * Needs to be reinitialised before being re-used. 00250 */ 00251 void turnOff(); 00252 00253 /** Clear 00254 * 00255 * Clears the screen buffer. 00256 */ 00257 void clear(); 00258 00259 /** Set screen constrast 00260 * @param constrast - float in range 0.0 to 1.0 (0.40 to 0.60 is usually a good value) 00261 */ 00262 void setContrast(float contrast); 00263 00264 /** Turn on normal video mode (default) 00265 * Black on white 00266 */ 00267 void normalMode(); 00268 00269 /** Turn on inverse video mode (default) 00270 * White on black 00271 */ 00272 void inverseMode(); 00273 00274 /** Backlight On 00275 * 00276 * Turns backlight on 00277 */ 00278 void backLightOn(); 00279 00280 /** Set Brightness 00281 * 00282 * Turns backlight off 00283 */ 00284 void backLightOff(); 00285 00286 /** Print String 00287 * 00288 * Prints a string of characters to the screen buffer. String is cut-off after the 83rd pixel. 00289 * @param x - the column number (0 to 83) 00290 * @param y - the row number (0 to 5) - the display is split into 6 banks - each bank can be considered a row 00291 */ 00292 void printString(char const *str, 00293 unsigned int const x, 00294 unsigned int const y); 00295 00296 /** Print Character 00297 * 00298 * Sends a character to the screen buffer. Printed at the specified location. Character is cut-off after the 83rd pixel. 00299 * @param c - the character to print. Can print ASCII as so printChar('C'). 00300 * @param x - the column number (0 to 83) 00301 * @param y - the row number (0 to 5) - the display is split into 6 banks - each bank can be considered a row 00302 */ 00303 void printChar(char const c, 00304 unsigned int const x, 00305 unsigned int const y); 00306 00307 /** 00308 * @brief Set a Pixel 00309 * 00310 * @param x The x co-ordinate of the pixel (0 to 83) 00311 * @param y The y co-ordinate of the pixel (0 to 47) 00312 * @param state The state of the pixel [true=black (default), false=white] 00313 * 00314 * @details This function sets the state of a pixel in the screen buffer. 00315 * The third parameter can be omitted, 00316 */ 00317 void setPixel(unsigned int const x, 00318 unsigned int const y, 00319 bool const state = true); 00320 00321 /** 00322 * @brief Clear a Pixel 00323 * 00324 * @param x - the x co-ordinate of the pixel (0 to 83) 00325 * @param y - the y co-ordinate of the pixel (0 to 47) 00326 * 00327 * @details This function clears pixel in the screen buffer 00328 * 00329 * @deprecated Use setPixel(x, y, false) instead 00330 */ 00331 void clearPixel(unsigned int const x, 00332 unsigned int const y) 00333 __attribute__((deprecated("Use setPixel(x,y,false) instead"))); 00334 00335 /** Get a Pixel 00336 * 00337 * This function gets the status of a pixel in the screen buffer. 00338 * @param x - the x co-ordinate of the pixel (0 to 83) 00339 * @param y - the y co-ordinate of the pixel (0 to 47) 00340 * @returns 00341 * 0 - pixel is clear 00342 * 1 - pixel is set 00343 */ 00344 int getPixel(unsigned int const x, 00345 unsigned int const y) const; 00346 00347 /** Refresh display 00348 * 00349 * This functions sends the screen buffer to the display. 00350 */ 00351 void refresh(); 00352 00353 /** Randomise buffer 00354 * 00355 * This function fills the buffer with random data. Can be used to test the display. 00356 * A call to refresh() must be made to update the display to reflect the change in pixels. 00357 * The seed is not set and so the generated pattern will probably be the same each time. 00358 * TODO: Randomise the seed - maybe using the noise on the AnalogIn pins. 00359 */ 00360 void randomiseBuffer(); 00361 00362 /** Plot Array 00363 * 00364 * This function plots a one-dimensional array in the buffer. 00365 * @param array[] - y values of the plot. Values should be normalised in the range 0.0 to 1.0. First 84 plotted. 00366 */ 00367 void plotArray(float const array[]); 00368 00369 /** Draw Circle 00370 * 00371 * This function draws a circle at the specified origin with specified radius in the screen buffer 00372 * Uses the midpoint circle algorithm. 00373 * @see http://en.wikipedia.org/wiki/Midpoint_circle_algorithm 00374 * @param x0 - x-coordinate of centre 00375 * @param y0 - y-coordinate of centre 00376 * @param radius - radius of circle in pixels 00377 * @param fill - fill-type for the shape 00378 */ 00379 void drawCircle(unsigned int const x0, 00380 unsigned int const y0, 00381 unsigned int const radius, 00382 FillType const fill); 00383 00384 /** Draw Line 00385 * 00386 * This function draws a line between the specified points using linear interpolation. 00387 * @param x0 - x-coordinate of first point 00388 * @param y0 - y-coordinate of first point 00389 * @param x1 - x-coordinate of last point 00390 * @param y1 - y-coordinate of last point 00391 * @param type - 0 white,1 black,2 dotted 00392 */ 00393 void drawLine(unsigned int const x0, 00394 unsigned int const y0, 00395 unsigned int const x1, 00396 unsigned int const y1, 00397 unsigned int const type); 00398 00399 /** Draw Rectangle 00400 * 00401 * This function draws a rectangle. 00402 * @param x0 - x-coordinate of origin (top-left) 00403 * @param y0 - y-coordinate of origin (top-left) 00404 * @param width - width of rectangle 00405 * @param height - height of rectangle 00406 * @param fill - fill-type for the shape 00407 */ 00408 void drawRect(unsigned int const x0, 00409 unsigned int const y0, 00410 unsigned int const width, 00411 unsigned int const height, 00412 FillType const fill); 00413 00414 /** Draw Sprite 00415 * 00416 * This function draws a sprite as defined in a 2D array 00417 * @param x0 - x-coordinate of origin (top-left) 00418 * @param y0 - y-coordinate of origin (top-left) 00419 * @param nrows - number of rows in sprite 00420 * @param ncols - number of columns in sprite 00421 * @param sprite - 2D array representing the sprite 00422 */ 00423 void drawSprite(int x0, 00424 int y0, 00425 int nrows, 00426 int ncols, 00427 int *sprite); 00428 00429 00430 private: 00431 // methods 00432 void setXYAddress(unsigned int const x, 00433 unsigned int const y); 00434 void initSPI(); 00435 void turnOn(); 00436 void reset(); 00437 void clearRAM(); 00438 void sendCommand(unsigned char command); 00439 void sendData(unsigned char data); 00440 void setTempCoefficient(char tc); // 0 to 3 00441 void setBias(char bias); // 0 to 7 00442 }; 00443 00444 const unsigned char font5x7[480] = { 00445 0x00, 0x00, 0x00, 0x00, 0x00,// (space) 00446 0x00, 0x00, 0x5F, 0x00, 0x00,// ! 00447 0x00, 0x07, 0x00, 0x07, 0x00,// " 00448 0x14, 0x7F, 0x14, 0x7F, 0x14,// # 00449 0x24, 0x2A, 0x7F, 0x2A, 0x12,// $ 00450 0x23, 0x13, 0x08, 0x64, 0x62,// % 00451 0x36, 0x49, 0x55, 0x22, 0x50,// & 00452 0x00, 0x05, 0x03, 0x00, 0x00,// ' 00453 0x00, 0x1C, 0x22, 0x41, 0x00,// ( 00454 0x00, 0x41, 0x22, 0x1C, 0x00,// ) 00455 0x08, 0x2A, 0x1C, 0x2A, 0x08,// * 00456 0x08, 0x08, 0x3E, 0x08, 0x08,// + 00457 0x00, 0x50, 0x30, 0x00, 0x00,// , 00458 0x08, 0x08, 0x08, 0x08, 0x08,// - 00459 0x00, 0x60, 0x60, 0x00, 0x00,// . 00460 0x20, 0x10, 0x08, 0x04, 0x02,// / 00461 0x3E, 0x51, 0x49, 0x45, 0x3E,// 0 00462 0x00, 0x42, 0x7F, 0x40, 0x00,// 1 00463 0x42, 0x61, 0x51, 0x49, 0x46,// 2 00464 0x21, 0x41, 0x45, 0x4B, 0x31,// 3 00465 0x18, 0x14, 0x12, 0x7F, 0x10,// 4 00466 0x27, 0x45, 0x45, 0x45, 0x39,// 5 00467 0x3C, 0x4A, 0x49, 0x49, 0x30,// 6 00468 0x01, 0x71, 0x09, 0x05, 0x03,// 7 00469 0x36, 0x49, 0x49, 0x49, 0x36,// 8 00470 0x06, 0x49, 0x49, 0x29, 0x1E,// 9 00471 0x00, 0x36, 0x36, 0x00, 0x00,// : 00472 0x00, 0x56, 0x36, 0x00, 0x00,// ; 00473 0x00, 0x08, 0x14, 0x22, 0x41,// < 00474 0x14, 0x14, 0x14, 0x14, 0x14,// = 00475 0x41, 0x22, 0x14, 0x08, 0x00,// > 00476 0x02, 0x01, 0x51, 0x09, 0x06,// ? 00477 0x32, 0x49, 0x79, 0x41, 0x3E,// @ 00478 0x7E, 0x11, 0x11, 0x11, 0x7E,// A 00479 0x7F, 0x49, 0x49, 0x49, 0x36,// B 00480 0x3E, 0x41, 0x41, 0x41, 0x22,// C 00481 0x7F, 0x41, 0x41, 0x22, 0x1C,// D 00482 0x7F, 0x49, 0x49, 0x49, 0x41,// E 00483 0x7F, 0x09, 0x09, 0x01, 0x01,// F 00484 0x3E, 0x41, 0x41, 0x51, 0x32,// G 00485 0x7F, 0x08, 0x08, 0x08, 0x7F,// H 00486 0x00, 0x41, 0x7F, 0x41, 0x00,// I 00487 0x20, 0x40, 0x41, 0x3F, 0x01,// J 00488 0x7F, 0x08, 0x14, 0x22, 0x41,// K 00489 0x7F, 0x40, 0x40, 0x40, 0x40,// L 00490 0x7F, 0x02, 0x04, 0x02, 0x7F,// M 00491 0x7F, 0x04, 0x08, 0x10, 0x7F,// N 00492 0x3E, 0x41, 0x41, 0x41, 0x3E,// O 00493 0x7F, 0x09, 0x09, 0x09, 0x06,// P 00494 0x3E, 0x41, 0x51, 0x21, 0x5E,// Q 00495 0x7F, 0x09, 0x19, 0x29, 0x46,// R 00496 0x46, 0x49, 0x49, 0x49, 0x31,// S 00497 0x01, 0x01, 0x7F, 0x01, 0x01,// T 00498 0x3F, 0x40, 0x40, 0x40, 0x3F,// U 00499 0x1F, 0x20, 0x40, 0x20, 0x1F,// V 00500 0x7F, 0x20, 0x18, 0x20, 0x7F,// W 00501 0x63, 0x14, 0x08, 0x14, 0x63,// X 00502 0x03, 0x04, 0x78, 0x04, 0x03,// Y 00503 0x61, 0x51, 0x49, 0x45, 0x43,// Z 00504 0x00, 0x00, 0x7F, 0x41, 0x41,// [ 00505 0x02, 0x04, 0x08, 0x10, 0x20,// "\" 00506 0x41, 0x41, 0x7F, 0x00, 0x00,// ] 00507 0x04, 0x02, 0x01, 0x02, 0x04,// ^ 00508 0x40, 0x40, 0x40, 0x40, 0x40,// _ 00509 0x00, 0x01, 0x02, 0x04, 0x00,// ` 00510 0x20, 0x54, 0x54, 0x54, 0x78,// a 00511 0x7F, 0x48, 0x44, 0x44, 0x38,// b 00512 0x38, 0x44, 0x44, 0x44, 0x20,// c 00513 0x38, 0x44, 0x44, 0x48, 0x7F,// d 00514 0x38, 0x54, 0x54, 0x54, 0x18,// e 00515 0x08, 0x7E, 0x09, 0x01, 0x02,// f 00516 0x08, 0x14, 0x54, 0x54, 0x3C,// g 00517 0x7F, 0x08, 0x04, 0x04, 0x78,// h 00518 0x00, 0x44, 0x7D, 0x40, 0x00,// i 00519 0x20, 0x40, 0x44, 0x3D, 0x00,// j 00520 0x00, 0x7F, 0x10, 0x28, 0x44,// k 00521 0x00, 0x41, 0x7F, 0x40, 0x00,// l 00522 0x7C, 0x04, 0x18, 0x04, 0x78,// m 00523 0x7C, 0x08, 0x04, 0x04, 0x78,// n 00524 0x38, 0x44, 0x44, 0x44, 0x38,// o 00525 0x7C, 0x14, 0x14, 0x14, 0x08,// p 00526 0x08, 0x14, 0x14, 0x18, 0x7C,// q 00527 0x7C, 0x08, 0x04, 0x04, 0x08,// r 00528 0x48, 0x54, 0x54, 0x54, 0x20,// s 00529 0x04, 0x3F, 0x44, 0x40, 0x20,// t 00530 0x3C, 0x40, 0x40, 0x20, 0x7C,// u 00531 0x1C, 0x20, 0x40, 0x20, 0x1C,// v 00532 0x3C, 0x40, 0x30, 0x40, 0x3C,// w 00533 0x44, 0x28, 0x10, 0x28, 0x44,// x 00534 0x0C, 0x50, 0x50, 0x50, 0x3C,// y 00535 0x44, 0x64, 0x54, 0x4C, 0x44,// z 00536 0x00, 0x08, 0x36, 0x41, 0x00,// { 00537 0x00, 0x00, 0x7F, 0x00, 0x00,// | 00538 0x00, 0x41, 0x36, 0x08, 0x00,// } 00539 0x08, 0x08, 0x2A, 0x1C, 0x08,// -> 00540 0x08, 0x1C, 0x2A, 0x08, 0x08 // <- 00541 }; 00542 00543 #endif
Generated on Sat Jul 16 2022 18:01:16 by
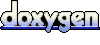