
Demo program for LCD and Joystick
Dependents: ELEC2645_Race_Collision
Joystick.h
00001 #ifndef JOYSTICK_H 00002 #define JOYSTICK_H 00003 00004 #include "mbed.h" 00005 00006 // this value can be tuned to alter tolerance of joystick movement 00007 #define TOL 0.1f 00008 #define RAD2DEG 57.2957795131f 00009 00010 enum Direction { 00011 CENTRE, // 0 00012 N, // 1 00013 NE, // 2 00014 E, // 3 00015 SE, // 4 00016 S, // 5 00017 SW, // 6 00018 W, // 7 00019 NW // 8 00020 }; 00021 00022 struct Vector2D { 00023 float x; 00024 float y; 00025 }; 00026 00027 struct Polar { 00028 float mag; 00029 float angle; 00030 }; 00031 00032 /** Joystick Class 00033 @author Dr Craig A. Evans, University of Leeds 00034 @brief Library for interfacing with analogue joystick 00035 00036 Example: 00037 00038 @code 00039 00040 #include "mbed.h" 00041 #include "Joystick.h" 00042 00043 // y x button 00044 Joystick joystick(PTB11,PTB10); 00045 00046 int main() { 00047 00048 joystick.init(); 00049 00050 while(1) { 00051 00052 Vector2D coord = joystick.get_coord(); 00053 printf("Coord = %f,%f\n",coord.x,coord.y); 00054 00055 Vector2D mapped_coord = joystick.get_mapped_coord(); 00056 printf("Mapped coord = %f,%f\n",mapped_coord.x,mapped_coord.y); 00057 00058 float mag = joystick.get_mag(); 00059 float angle = joystick.get_angle(); 00060 printf("Mag = %f Angle = %f\n",mag,angle); 00061 00062 Direction d = joystick.get_direction(); 00063 printf("Direction = %i\n",d); 00064 00065 00066 wait(0.5); 00067 } 00068 00069 00070 } 00071 00072 * @endcode 00073 */ 00074 class Joystick 00075 { 00076 public: 00077 00078 // y-pot x-pot 00079 Joystick(PinName vertPin,PinName horizPin); 00080 00081 void init(); // needs to be called at start with joystick centred 00082 float get_mag(); // polar 00083 float get_angle(); // polar 00084 Vector2D get_coord(); // cartesian co-ordinates x,y 00085 Vector2D get_mapped_coord(); // x,y mapped to circle 00086 Direction get_direction(); // N,NE,E,SE etc. 00087 Polar get_polar(); // mag and angle in struct form 00088 00089 private: 00090 00091 AnalogIn *vert; 00092 AnalogIn *horiz; 00093 00094 // centred x,y values 00095 float _x0; 00096 float _y0; 00097 00098 }; 00099 00100 #endif
Generated on Thu Jul 14 2022 03:32:24 by
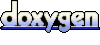