
Example to move the robot.
Fork of 3pi_Line_Follow by
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "main.h" 00002 00003 // API objects 00004 m3pi robot; 00005 00006 // LEDs on the Mbed board 00007 BusOut leds(LED4,LED3,LED2,LED1); 00008 00009 // Buttons on the 3pi shield 00010 DigitalIn button_A(p18); 00011 DigitalIn button_B(p17); 00012 DigitalIn button_X(p21); 00013 DigitalIn button_Y(p22); 00014 DigitalIn button_enter(p24); 00015 DigitalIn button_back(p23); 00016 00017 // Blue potentiometers on the 3pi shield 00018 AnalogIn pot_P(p15); 00019 AnalogIn pot_I(p16); 00020 AnalogIn pot_D(p19); 00021 AnalogIn pot_S(p20); 00022 00023 00024 // This bit is the main function and is the code that is run when the buggies are powered up 00025 int main() 00026 { 00027 init(); 00028 welcome(); 00029 //calibrate(); 00030 00031 float dt = 1.0/50.0; 00032 00033 wait_for_enter(); 00034 00035 // keep looping until back is pressed 00036 while(button_back.read() == 1) { 00037 repeat(); 00038 wait(dt); 00039 } 00040 00041 robot.stop(); 00042 robot.lcd_clear(); 00043 leds = 0b0000; 00044 } 00045 00046 // Functions 00047 void init() 00048 { 00049 robot.init(); 00050 00051 button_A.mode(PullUp); 00052 button_B.mode(PullUp); 00053 button_X.mode(PullUp); 00054 button_Y.mode(PullUp); 00055 button_enter.mode(PullUp); 00056 button_back.mode(PullUp); 00057 00058 leds = 0b0000; 00059 } 00060 00061 void welcome() 00062 { 00063 robot.lcd_clear(); 00064 const char g_song2[]= "L16 cdegreg4"; 00065 robot.play_music(g_song2,sizeof(g_song2)); 00066 robot.display_battery_voltage(0,0); 00067 robot.display_signature(0,1); 00068 wait(3.0); 00069 00070 } 00071 00072 // this makes the buggy rotate left and right 00073 // should be placed over a black line so the difference 00074 // between white and black can be determined 00075 void calibrate() 00076 { 00077 leds = 0b1111; 00078 robot.reset_calibration(); 00079 00080 wait_for_enter(); 00081 00082 00083 wait(2.0); // leave a bit of delay so hands can be moved out of the way 00084 robot.auto_calibrate(); // run calibration routine 00085 leds = 0b0000; 00086 } 00087 00088 void wait_for_enter() 00089 { 00090 // display message on LCD 00091 robot.lcd_clear(); 00092 robot.lcd_goto_xy(0,0); 00093 robot.lcd_print("Place on",8); 00094 robot.lcd_goto_xy(0,1); 00095 robot.lcd_print(" line ",8); 00096 wait(1.0); 00097 // display message on LCD 00098 robot.lcd_clear(); 00099 robot.lcd_goto_xy(0,0); 00100 robot.lcd_print(" Press ",8); 00101 robot.lcd_goto_xy(0,1); 00102 robot.lcd_print(" ENTER ",8); 00103 00104 while (button_enter.read() == 1) { // wait for button press 00105 00106 } 00107 00108 robot.lcd_clear(); 00109 00110 }
Generated on Sun Jul 17 2022 17:43:01 by
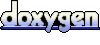