
Reading sensors
Fork of 3pi_Lab1_Task2 by
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 /* 3pi Lab 1 Example 2 00002 00003 (c) Dr Craig A. Evans, University of Leeds 00004 00005 June 2017 00006 00007 */ 00008 00009 #include "mbed.h" 00010 #include "m3pi.h" 00011 00012 // API objects 00013 m3pi robot; 00014 00015 // Function Prototypes 00016 void init(); 00017 00018 // Main Function 00019 int main() 00020 { 00021 init(); 00022 00023 // move cursor to position (0,0) - top-left 00024 robot.lcd_goto_xy(0,0); 00025 robot.lcd_print("Lab 1",5); // 5 is number of characters in message (max 8) 00026 robot.lcd_goto_xy(0,1); 00027 robot.lcd_print("Task 3",6); 00028 00029 wait(2.0); 00030 00031 // reset previous calibration values and run auto-calibrate routine 00032 robot.reset_calibration(); 00033 // robot must be placed over a black line 00034 robot.auto_calibrate(); 00035 00036 // an array to store each of the sensor values 00037 unsigned int values[5]= {0}; 00038 00039 float dt = 1.0/10.0; // we'll read the sensors 10 times a second 00040 00041 // main loop - this runs forever 00042 while(1) { 00043 00044 // clear the LCD so we can draw new information on every loop 00045 robot.lcd_clear(); 00046 00047 // read the sensor values and store in array 00048 // these values are 0 to 1000 (0 is white/1000 is black) 00049 robot.get_calibrated_values(values); 00050 00051 // Can see the values using CoolTerm - select correct COM port 00052 for (int i=0; i< 5 ; i++) { 00053 printf("[%i] = %i | ",i,values[i]); 00054 } 00055 printf("\n"); 00056 00057 // display a bar graph on the LCD 00058 robot.display_sensor_values(values,1); 00059 00060 // in range -1 to 1, no error when line is at 0.0 00061 // number is negative when the line is to the left 00062 // number is positive when the line is to the right 00063 float line_position = robot.calc_line_position(values); 00064 00065 // empty buffer to store values 00066 char buffer[6]; 00067 sprintf(buffer,"%+.3f",line_position); // create message and store in array .3 means three decimal places 00068 // print line position on LCD 00069 robot.lcd_goto_xy(1,0); 00070 robot.lcd_print(buffer,6); 00071 00072 // wait for a short time before repeating the loop 00073 wait(dt); 00074 00075 } 00076 } 00077 00078 // Functions 00079 void init() 00080 { 00081 robot.init(); 00082 }
Generated on Fri Jul 22 2022 03:06:55 by
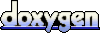