
Lab 1 Task 2 example code
Fork of 3pi_Lab1_Task2_Example1 by
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 /* 3pi Lab 1 Example 2 00002 00003 (c) Dr Craig A. Evans, University of Leeds 00004 00005 June 2017 00006 00007 */ 00008 00009 #include "mbed.h" 00010 #include "m3pi.h" 00011 00012 // API objects 00013 m3pi robot; 00014 AnalogIn pot_P(p15); 00015 00016 // Function Prototypes 00017 void init(); 00018 00019 // Main Function 00020 int main() 00021 { 00022 init(); 00023 00024 // move cursor to position (0,0) - top-left 00025 robot.lcd_goto_xy(0,0); 00026 robot.lcd_print("Lab 1",5); // 5 is number of characters in message (max 8) 00027 robot.lcd_goto_xy(0,1); 00028 robot.lcd_print("Task 2",6); 00029 00030 // we will update the motors 100 times per second 00031 float dt = 1.0/100.0; 00032 00033 // main loop - this runs forever 00034 while(1) { 00035 00036 // this returns a value in the range 0.0 to 1.0 00037 float pot_P_val = pot_P.read(); 00038 00039 // change to -1.0 to 1.0 00040 float motor_speed = 2.0*pot_P_val-1.0; 00041 // this gives full-speed backward (-1.0) to full-speed forward 00042 00043 // set the speed of the left and right motors 00044 robot.motors(motor_speed,motor_speed); 00045 00046 // wait for a short time before repeating the loop 00047 wait(dt); 00048 00049 } 00050 } 00051 00052 // Functions 00053 void init() 00054 { 00055 robot.init(); 00056 }
Generated on Fri Jul 15 2022 10:34:02 by
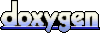