
Code for testing the ELEC1620 Application Board.
Dependencies: N5110 ShiftReg Tone mbed
main.cpp
00001 #include "main.h" 00002 00003 // objects defined here with pin numbers 00004 DigitalIn button_a(p29); 00005 DigitalIn button_b(p28); 00006 DigitalIn button_c(p27); 00007 DigitalIn button_d(p26); 00008 00009 AnalogIn ldr(p15); 00010 BusOut leds(LED4,LED3,LED2,LED1); 00011 Tone speaker(p18); 00012 00013 BusOut rgb_led(p24,p23,p22); 00014 00015 AnalogIn tmp36(p16); 00016 00017 AnalogIn pot_0(p20); 00018 AnalogIn pot_1(p19); 00019 AnalogIn pot_2(p17); 00020 00021 N5110 lcd(p8,p9,p10,p11,p13,p21); 00022 ShiftReg shift; 00023 00024 int seven_seg_array[] = { 00025 0x3F,0x06,0x5B,0x4F,0x66,0x6D,0x7D,0x07,0x7F,0x67 00026 }; 00027 // array that stores the colors R, G and B 00028 int rgd_led_array[] = { 00029 0b011,0b101,0b110 00030 }; 00031 00032 int led_val = 0; 00033 00034 void init(); 00035 void test_7seg(); 00036 void test_buttons(); 00037 void test_leds(); 00038 void test_ldr(); 00039 void test_tmp(); 00040 void test_pots(); 00041 00042 int main() 00043 { 00044 // initialise peripherals 00045 init(); 00046 00047 // test the constant outputs 00048 test_7seg(); 00049 leds = 0x0F; // on-board LEDs 00050 speaker.play(1000.0,2.0); 00051 00052 // loop forever 00053 while(1) { 00054 00055 // clear LCD at beginning of each 'frame' 00056 lcd.clear(); 00057 lcd.printString("Test",0,0); 00058 00059 // test inputs and outputs that can change 00060 00061 test_buttons(); 00062 test_leds(); 00063 test_ldr(); 00064 test_tmp(); 00065 test_pots(); 00066 00067 // print everything on the screen 00068 lcd.refresh(); 00069 // small delay before next frame is drawn 00070 wait(1.0/4); 00071 } 00072 00073 } 00074 00075 void init() 00076 { 00077 shift.write(0x00); 00078 00079 lcd.init(); 00080 00081 speaker.init(); 00082 00083 // PCB has external pull-down resistors so turn the internal ones off 00084 // (default for DigitalIn) 00085 button_a.mode(PullNone); 00086 button_b.mode(PullNone); 00087 button_c.mode(PullNone); 00088 button_d.mode(PullNone); 00089 } 00090 00091 void test_7seg() 00092 { 00093 shift.write(0xFF); 00094 } 00095 00096 void test_buttons() 00097 { 00098 // check each button, and print character on screen 00099 if ( button_a.read() == 1) { 00100 lcd.printChar('A',0,1); 00101 } 00102 if ( button_b.read() == 1) { 00103 lcd.printChar('B',8,1); 00104 } 00105 if ( button_c.read() == 1) { 00106 lcd.printChar('C',16,1); 00107 } 00108 if ( button_d.read() == 1) { 00109 lcd.printChar('D',24,1); 00110 } 00111 } 00112 00113 void test_leds() 00114 { 00115 00116 // set RGB to current val 00117 rgb_led = rgd_led_array[led_val]; 00118 00119 // increment the val (i.e. change colour combination) 00120 led_val++; 00121 00122 // once we've got to end of array, go back to beginning 00123 if (led_val == 3) { 00124 led_val = 0; 00125 } 00126 00127 } 00128 00129 void test_ldr() 00130 { 00131 float value = ldr.read(); // read in the LDR value in range 0.0 to 1.0 00132 char buffer[14]; 00133 00134 sprintf(buffer,"LDR=%.2f",value); // print formatted data to buffer 00135 lcd.printString(buffer,0,2); // display on screen 00136 } 00137 00138 void test_tmp() 00139 { 00140 float value = 3.3*tmp36.read(); // read in the TMP36 value in range 0.0 to 1.0 00141 float temperature = 100.0f*value - 50.0f; 00142 char buffer[14]; 00143 00144 sprintf(buffer,"T=%.4f C",temperature); // print formatted data to buffer 00145 lcd.printString(buffer,0,3); // display on screen 00146 } 00147 00148 void test_pots() 00149 { 00150 00151 char buffer[14]; 00152 00153 float val = pot_0.read(); 00154 sprintf(buffer,"%.2f",val); // print formatted data to buffer 00155 lcd.printString(buffer,0,5); // display on screen 00156 lcd.setContrast(val); // tune LCD contrast 00157 00158 val = pot_1.read(); 00159 sprintf(buffer,"%.2f",val); // print formatted data to buffer 00160 lcd.printString(buffer,28,5); // display on screen 00161 00162 val = pot_2.read(); 00163 sprintf(buffer,"%.2f",val); // print formatted data to buffer 00164 lcd.printString(buffer,56,5); // display on screen 00165 }
Generated on Thu Jul 14 2022 19:45:43 by
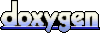