
Using DAC to play a melody
Fork of 1620_App_Board_UART_getc by
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 /* ELEC1620 Application Board Example 00002 00003 Example of the Tone library to interface with the DAC 00004 00005 (c) Dr Craig A. Evans, University of Leeds, March 2017 00006 00007 */ 00008 00009 #include "mbed.h" 00010 #include "Tone.h" 00011 00012 Tone dac(p18); 00013 00014 // Super Mario Theme Tune 00015 const int note_array[] = { 00016 NOTE_E7, NOTE_E7, 0, NOTE_E7, 00017 0, NOTE_C7, NOTE_E7, 0, 00018 NOTE_G7, 0, 0, 0, 00019 NOTE_G6, 0, 0, 0, 00020 00021 NOTE_C7, 0, 0, NOTE_G6, 00022 0, 0, NOTE_E6, 0, 00023 0, NOTE_A6, 0, NOTE_B6, 00024 0, NOTE_AS6, NOTE_A6, 0, 00025 00026 NOTE_G6, NOTE_E7, NOTE_G7, 00027 NOTE_A7, 0, NOTE_F7, NOTE_G7, 00028 0, NOTE_E7, 0,NOTE_C7, 00029 NOTE_D7, NOTE_B6, 0, 0, 00030 00031 NOTE_C7, 0, 0, NOTE_G6, 00032 0, 0, NOTE_E6, 0, 00033 0, NOTE_A6, 0, NOTE_B6, 00034 0, NOTE_AS6, NOTE_A6, 0, 00035 00036 NOTE_G6, NOTE_E7, NOTE_G7, 00037 NOTE_A7, 0, NOTE_F7, NOTE_G7, 00038 0, NOTE_E7, 0,NOTE_C7, 00039 NOTE_D7, NOTE_B6, 0, 0 00040 }; 00041 00042 // 8 corresponds to 1/8 00043 const int duration_array[] = { 00044 8,8,8,8, 00045 8,8,8,8, 00046 8,8,8,8, 00047 8,8,8,8, 00048 00049 8,8,8,8, 00050 8,8,8,8, 00051 8,8,8,8, 00052 8,8,8,8, 00053 00054 6,6,6, 00055 8,8,8,8, 00056 8,8,8,8, 00057 8,8,8,8, 00058 00059 8,8,8,8, 00060 8,8,8,8, 00061 8,8,8,8, 00062 8,8,8,8, 00063 00064 6,6,6, 00065 8,8,8,8, 00066 8,8,8,8, 00067 8,8,8,8, 00068 }; 00069 00070 int main() 00071 { 00072 dac.init(); 00073 00074 int n = sizeof(note_array)/sizeof(int); 00075 // tell it the number of notes, arrays, BPM and whether to repeat 00076 dac.play_melody(n,note_array,duration_array,120.0,true); 00077 00078 }
Generated on Sat Jul 23 2022 00:34:01 by
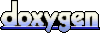