Metro SW timers as in Arduino
Embed:
(wiki syntax)
Show/hide line numbers
Metro.cpp
00001 /* 00002 The MIT License (MIT) 00003 00004 Copyright (c) 2013 thomasfredericks 00005 Copyright (c) 2015 Eduardo de Mier 00006 00007 Permission is hereby granted, free of charge, to any person obtaining a copy of 00008 this software and associated documentation files (the "Software"), to deal in 00009 the Software without restriction, including without limitation the rights to 00010 use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of 00011 the Software, and to permit persons to whom the Software is furnished to do so, 00012 subject to the following conditions: 00013 00014 The above copyright notice and this permission notice shall be included in all 00015 copies or substantial portions of the Software. 00016 00017 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00018 IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS 00019 FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR 00020 COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER 00021 IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN 00022 CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00023 */ 00024 00025 #include "Metro.h" 00026 #include "Arduino.h" 00027 00028 Metro::Metro(unsigned long interval_millis) 00029 { 00030 this->autoreset = 0; 00031 interval(interval_millis); 00032 reset(); 00033 } 00034 00035 // New creator so I can use either the original check behavior or benjamin.soelberg's 00036 // suggested one (see below). 00037 // autoreset = 0 is benjamin.soelberg's check behavior 00038 // autoreset != 0 is the original behavior 00039 00040 Metro::Metro(unsigned long interval_millis, uint8_t autoreset) 00041 { 00042 this->autoreset = autoreset; // Fix by Paul Bouchier 00043 interval(interval_millis); 00044 reset(); 00045 } 00046 00047 void Metro::interval(unsigned long interval_millis) 00048 { 00049 this->interval_millis = interval_millis; 00050 } 00051 00052 // Benjamin.soelberg's check behavior: 00053 // When a check is true, add the interval to the internal counter. 00054 // This should guarantee a better overall stability. 00055 00056 // Original check behavior: 00057 // When a check is true, add the interval to the current millis() counter. 00058 // This method can add a certain offset over time. 00059 00060 char Metro::check() 00061 { 00062 if (millis() - this->previous_millis >= this->interval_millis) { 00063 // As suggested by benjamin.soelberg@gmail.com, the following line 00064 // this->previous_millis = millis(); 00065 // was changed to 00066 // this->previous_millis += this->interval_millis; 00067 00068 // If the interval is set to 0 we revert to the original behavior 00069 if (this->interval_millis <= 0 || this->autoreset ) { 00070 this->previous_millis = millis(); 00071 } else { 00072 this->previous_millis += this->interval_millis; 00073 } 00074 00075 return 1; 00076 } 00077 00078 00079 00080 return 0; 00081 00082 } 00083 00084 void Metro::reset() 00085 { 00086 00087 this->previous_millis = millis(); 00088 00089 } 00090
Generated on Wed Aug 3 2022 13:40:14 by
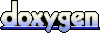