Library for TEA5767 FM stereo radio module
Dependents: TEA5767_RadioFM_Test_Code
TEA5767.h
00001 /* 00002 * Author: Edoardo De Marchi 00003 * Date: 05/10/13 00004 * Notes: Library for TEA5767 radio module 00005 */ 00006 00007 #pragma once 00008 #include "mbed.h" 00009 00010 00011 /** TEA5767 class 00012 * 00013 */ 00014 class TEA5767 00015 { 00016 00017 public: 00018 /** Creates an TEA5767 object connected to the specified I2C object 00019 * 00020 * @param sda I2C data port 00021 * @param scl I2C clock port 00022 * @param addr the I2C address of the TEA5767 device 00023 */ 00024 TEA5767(PinName sda, PinName scl, int addr); 00025 00026 /** Destroys a TEA5767 object 00027 * 00028 */ 00029 ~TEA5767(); 00030 00031 /** Set the band FM 00032 * 00033 * @param valueBand set the band ('e' = EUROPE/US - 'j' = JAPANESE) 00034 */ 00035 void SetBand(char valueBand); 00036 00037 /** Set the radio frequency 00038 * 00039 * @param freq frequency 00040 * @param side side injection mode ('h' = HIGH - 'l' = LOW) 00041 */ 00042 void SetFrequency(double freq, char side = 'h'); 00043 00044 /** Auto search up frequency 00045 * 00046 * @param freq frequency 00047 */ 00048 void SearchUp(float freq); 00049 00050 /** Auto search down frequency 00051 * 00052 * @param freq frequency 00053 */ 00054 void SearchDown(float freq); 00055 00056 /** Read the current frequancy 00057 * 00058 * @returns the current frequency 00059 */ 00060 float FreqCurrent(); 00061 00062 /** Checks if the address exist on an I2C bus 00063 * 00064 * @returns 0 on success, or non-0 on failure 00065 */ 00066 int CheckDevice(); 00067 00068 /** Read the signal level of the band 00069 * 00070 * @returns the signal level 00071 */ 00072 int SignalLevel(); 00073 00074 00075 private: 00076 00077 /** Initialization of the device 00078 * 00079 */ 00080 void Init(); 00081 00082 /** calculation of the high or low side injection 00083 * 00084 * @param freq the wanted tuning frequency 00085 * @param mode I2C high ('h') or low ('l') side injection 00086 * @returns decimal value of PLL word 00087 */ 00088 unsigned int SideInjection(float freq, char mode); 00089 00090 /** Set the 5 address of the device 00091 * 00092 * @param N 14bits PLL word 00093 * @param data_1 set the bit 7 and 6 of 1st data byte (default value = 0x00) 00094 * @param data_3 set the 3th data byte (default value = 0x10) 00095 * @param data_4 set the 4th data byte (default value = 0x10) 00096 * @param data_5 set the 5th data byte (default value = 0x00) 00097 */ 00098 void SetData(unsigned int N, char data_1 = 0x00, char data_3 = 0x10, char data_4 = 0x10, char data_5 = 0x00); 00099 00100 /** Read the 5 address of the device 00101 * 00102 * @param buf buffer in which the 5 addresses are saved 00103 */ 00104 void GetData(char* buf); 00105 00106 char band; 00107 char side; 00108 unsigned int frequency; 00109 I2C i2c; 00110 int addr; 00111 00112 };
Generated on Tue Jul 12 2022 20:43:49 by
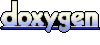