Library for TEA5767 FM stereo radio module
Dependents: TEA5767_RadioFM_Test_Code
TEA5767.cpp
00001 #include "TEA5767.h" 00002 00003 00004 TEA5767::TEA5767(PinName sda, PinName scl, int addr) : i2c(sda, scl), addr(addr) 00005 { 00006 SetBand('e'); 00007 Init(); 00008 } 00009 00010 00011 TEA5767::~TEA5767() 00012 { 00013 } 00014 00015 00016 void TEA5767::Init() 00017 { 00018 if(band == 'e') 00019 { 00020 SetFrequency(87.5, 'h'); // starting frequency 00021 }else 00022 { 00023 SetFrequency(76, 'h'); // starting frequency 00024 } 00025 } 00026 00027 00028 void TEA5767::SetBand(char valueBand) 00029 { 00030 if(valueBand == 'e' | valueBand == 'j') 00031 band = valueBand; 00032 } 00033 00034 00035 void TEA5767::SetFrequency(double freq, char side) 00036 { 00037 frequency = SideInjection(freq, side); 00038 SetData(frequency); 00039 } 00040 00041 00042 unsigned int TEA5767::SideInjection(float freq, char mode) 00043 { 00044 side = mode; 00045 if(side == 'h') 00046 { 00047 // IF = 225KHz 00048 unsigned int N_h = (4 * (freq * 1000000 + 225000)) / 32768; // formula for high side injection 00049 return N_h; // return PLL word 00050 }else 00051 { 00052 unsigned int N_l = (4 * (freq * 1000000 - 225000)) / 32768; // formula for low side injection 00053 return N_l; // return PLL word 00054 } 00055 } 00056 00057 00058 void TEA5767::SearchUp(float freq) 00059 { 00060 SetData(SideInjection(freq, 'h'), 0x40, 0xd0, 0x10, 0x00); 00061 } 00062 00063 00064 void TEA5767::SearchDown(float freq) 00065 { 00066 SetData(SideInjection(freq, 'h'), 0x40, 0x50, 0x10, 0x00); 00067 } 00068 00069 00070 float TEA5767::FreqCurrent() 00071 { 00072 char buf_temp[5]; 00073 GetData(buf_temp); 00074 frequency = ((buf_temp[0]&0x3f)<<8) | buf_temp[1]; 00075 00076 if(side == 'h') 00077 { 00078 return (((((float)frequency*32768)/4)-225000)/1000000); 00079 }else 00080 { 00081 return (((((float)frequency*32768)/4)+225000)/1000000); 00082 } 00083 } 00084 00085 00086 void TEA5767::SetData(unsigned int N, char data_1, char data_3, char data_4, char data_5) 00087 { 00088 00089 00090 char buf[5]; 00091 char freqMSB = N >> 8; // 1st 6bit 00092 char freqLSB = N & 0xff; // 2nd 8bit 00093 00094 buf[0] = ((data_1 & 0xc0) | freqMSB); 00095 buf[1] = freqLSB; 00096 buf[2] = data_3; 00097 if(band == 'e') 00098 { 00099 buf[3] = data_4 & 0xdf; 00100 }else 00101 { 00102 buf[3] = data_4 | 0x10; 00103 } 00104 buf[4] = data_5; 00105 00106 i2c.write(addr, buf, 5); 00107 } 00108 00109 00110 00111 void TEA5767::GetData(char* reg) 00112 { 00113 memset(reg, 0, sizeof(reg)); 00114 i2c.read(addr, reg, 5); 00115 } 00116 00117 00118 int TEA5767::CheckDevice() 00119 { 00120 return i2c.write(addr, NULL, 0); 00121 } 00122 00123 00124 int TEA5767::SignalLevel() 00125 { 00126 char reg[5]; 00127 GetData(reg); 00128 return ((reg[3]&0xf0)>>4); 00129 } 00130
Generated on Tue Jul 12 2022 20:43:49 by
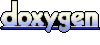