
This is an example to broadcast measured value of BME280 through BLE GATT service.
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 #include "BME280.h" 00003 #include "ble/BLE.h" 00004 #include "ble/services/EnvironmentalService.h" 00005 00006 #if defined(_DEBUG) 00007 Serial pc(USBTX, USBRX); 00008 #endif 00009 00010 #if defined(TARGET_TY51822R3) 00011 BME280 sensor(I2C_SDA0, I2C_SCL0); 00012 #else 00013 BME280 sensor(I2C_SDA, I2C_SCL); 00014 #endif 00015 00016 const static char DEVICE_NAME[] = "BME280"; 00017 const static uint16_t uuid16_list[] = {GattService::UUID_ENVIRONMENTAL_SERVICE}; 00018 static EnvironmentalService *service = NULL; 00019 static EventQueue eventQueue(/* event count */ 16 * EVENTS_EVENT_SIZE); 00020 00021 void disconnectionCallback(const Gap::DisconnectionCallbackParams_t *params) 00022 { 00023 BLE::Instance(BLE::DEFAULT_INSTANCE).gap().startAdvertising(); // restart advertising 00024 } 00025 00026 void bleInitComplete(BLE::InitializationCompleteCallbackContext *params) 00027 { 00028 BLE &ble = params->ble; 00029 ble_error_t error = params->error; 00030 service = new EnvironmentalService(ble); 00031 #if defined(_DEBUG) 00032 pc.printf("Inside BLE..starting payload creation..\r\n"); 00033 #endif 00034 ble.gap().onDisconnection(disconnectionCallback); 00035 00036 /* Setup advertising. */ 00037 ble.gap().accumulateAdvertisingPayload(GapAdvertisingData::BREDR_NOT_SUPPORTED | GapAdvertisingData::LE_GENERAL_DISCOVERABLE); 00038 ble.gap().accumulateAdvertisingPayload(GapAdvertisingData::COMPLETE_LIST_16BIT_SERVICE_IDS, (uint8_t *)uuid16_list, sizeof(uuid16_list)); 00039 ble.gap().accumulateAdvertisingPayload(GapAdvertisingData::COMPLETE_LOCAL_NAME, (uint8_t *)DEVICE_NAME, sizeof(DEVICE_NAME)); 00040 ble.gap().setAdvertisingType(GapAdvertisingParams::ADV_CONNECTABLE_UNDIRECTED); 00041 ble.gap().setAdvertisingInterval(1000); 00042 error = ble.gap().startAdvertising(); 00043 #if defined(_DEBUG) 00044 pc.printf("ble.gap().startAdvertising() => %u\r\n", error); 00045 #endif 00046 } 00047 00048 void readSensorCallback(void) 00049 { 00050 float tmp_t, tmp_p, tmp_h; 00051 00052 if(service != NULL) { 00053 tmp_t = sensor.getTemperature(); 00054 tmp_p = sensor.getPressure(); 00055 tmp_h = sensor.getHumidity(); 00056 service->updatePressure(tmp_p); 00057 service->updateTemperature(tmp_t); 00058 service->updateHumidity(tmp_h); 00059 #if defined(_DEBUG) 00060 pc.printf("%04.2f hPa, %2.2f degC, %2.2f %%\r\n", tmp_p, tmp_t, tmp_h ); 00061 #endif 00062 } 00063 } 00064 00065 void scheduleBleEventsProcessing(BLE::OnEventsToProcessCallbackContext* context) 00066 { 00067 BLE &ble = BLE::Instance(); 00068 eventQueue.call(Callback<void()>(&ble, &BLE::processEvents)); 00069 } 00070 00071 int main() 00072 { 00073 eventQueue.call_every(1000, readSensorCallback); 00074 00075 BLE &ble = BLE::Instance(); 00076 ble.onEventsToProcess(scheduleBleEventsProcessing); 00077 ble.init(bleInitComplete); 00078 00079 eventQueue.dispatch_forever(); 00080 00081 return 0; 00082 }
Generated on Thu Jul 14 2022 17:13:59 by
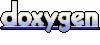