
Testing getting PJON working on mbed https://github.com/gioblu/PJON
Embed:
(wiki syntax)
Show/hide line numbers
pjon.h
00001 00002 /*-O//\ __ __ 00003 |-gfo\ |__| | | | |\ | 00004 |!y°o:\ | __| |__| | \| v1.0 00005 |y"s§+`\ Giovanni Blu Mitolo 2012 - 2015 00006 /so+:-..`\ gioscarab@gmail.com 00007 |+/:ngr-*.`\ 00008 |5/:%&-a3f.:;\ PJON is a device communications bus system that connects up to 255 00009 \+//u/+g%{osv,,\ arduino boards over one wire up to 5.29kB/s data communication speed. 00010 \=+&/osw+olds.\\ Contains acknowledge, collision detection, CRC and encpryption all done 00011 \:/+-.-°-:+oss\ with micros() and delayMicroseconds(), with no use of interrupts or timers. 00012 | | \oy\\ Pull down resistor on the bus is generally used to reduce interference. 00013 > < 00014 _____-| |-________________________________________________________________________ 00015 | BIT_WIDTH 20 | BIT_SPACER 56 | ACCEPTANCE 20 | READ_DELAY 8 | 00016 |----------------------------------------------------------------------------------| 00017 |Transfer speed: 5.29kB/s | Absolute bandwidth: 3.23 kB/s | 00018 |Baud rate: 42372 baud | Data throughput: 2.68 kB/s | 00019 |__________________________________________________________________________________| 00020 00021 Copyright (c) 2012-2015, Giovanni Blu Mitolo All rights reserved. 00022 00023 Redistribution and use in source and binary forms, with or without 00024 modification, are permitted provided that the following conditions are met: 00025 - Redistributions of source code must retain the above copyright 00026 notice, this list of conditions and the following disclaimer. 00027 00028 - Redistributions in binary form must reproduce the above copyright 00029 notice, this list of conditions and the following disclaimer in the 00030 documentation and/or other materials provided with the distribution. 00031 00032 - All advertising materials mentioning features or use of this software 00033 must display the following acknowledgement: 00034 This product includes PJON software developed by Giovanni Blu Mitolo. 00035 00036 - Neither the name of PJON, PJON_ASK nor the 00037 names of its contributors may be used to endorse or promote products 00038 derived from this software without specific prior written permission. 00039 00040 This software is provided by the copyright holders and contributors"as is" 00041 and any express or implied warranties, including, but not limited to, the 00042 implied warranties of merchantability and fitness for a particular purpose 00043 are disclaimed. In no event shall the copyright holder or contributors be 00044 liable for any direct, indirect, incidental, special, exemplary, or consequential 00045 damages (including, but not limited to, procurement of substitute goods or services; 00046 loss of use, data, or profits; or business interruption) however caused and on any 00047 theory of liability, whether in contract, strict liability, or tort (including 00048 negligence or otherwise) arising in any way out of the use of this software, even if 00049 advised of the possibility of such damage. */ 00050 00051 #ifndef PJON_h 00052 #define PJON_h 00053 00054 #include "mbed.h" 00055 00056 /* COMPATIBILITY_MODE set to true: 00057 Run the network with a cautious speed to support all architectures. 00058 8Mhz devices will be able to communicate with 16 or more Mhz devices. 00059 COMPATIBILITY_MODE set to false: 00060 Run the network at full speed with the best performances, but is 00061 indicated only for networks made by a group of devices with the 00062 same architecture / processor (for example 10 Arduino Uno) */ 00063 00064 #define COMPATIBILITY_MODE true 00065 00066 //mbed (needs tweaking) 00067 /* 00068 #define BIT_WIDTH 52 00069 #define BIT_SPACER 121 00070 #define ACCEPTANCE 51 00071 #define READ_DELAY 29 00072 */ 00073 #define BIT_WIDTH 45 00074 #define BIT_SPACER 113 00075 #define ACCEPTANCE 45 00076 #define READ_DELAY 23 00077 /* The following constants setup are quite conservative and determined only 00078 with a huge amount of time and blind testing (without oscilloscope) 00079 tweaking values and analysing results. Theese can be changed to obtain 00080 faster speed. Probably you need experience, time and an oscilloscope. */ 00081 00082 #if defined(__AVR_ATmega88__) || defined(__AVR_ATmega168__) || defined(__AVR_ATmega328__) || defined(__AVR_ATmega328P__) 00083 #if (F_CPU == 16000000 && !COMPATIBILITY_MODE) 00084 #define BIT_WIDTH 20 00085 #define BIT_SPACER 56 00086 #define ACCEPTANCE 20 00087 #define READ_DELAY 8 00088 #endif 00089 #if (F_CPU == 8000000 || COMPATIBILITY_MODE) 00090 #define BIT_WIDTH 40 00091 #define BIT_SPACER 112 00092 #define ACCEPTANCE 40 00093 #define READ_DELAY 16 00094 #endif 00095 #endif 00096 00097 #if defined(__AVR_ATmega1280__) || defined(__AVR_ATmega2560__) 00098 #if (F_CPU == 16000000 && !COMPATIBILITY_MODE) 00099 /* Mega has shorter values than Duemianove / Uno because 00100 micros() produces longer delays on ATmega1280/2560 */ 00101 #define BIT_WIDTH 18 00102 #define BIT_SPACER 54 00103 #define ACCEPTANCE 18 00104 #define READ_DELAY 8 00105 #endif 00106 #if (F_CPU == 8000000 || COMPATIBILITY_MODE) 00107 /* Mega has shorter values than Duemianove / Uno because 00108 micros() produces longer delays on ATmega1280/2560 */ 00109 #define BIT_WIDTH 38 00110 #define BIT_SPACER 110 00111 #define ACCEPTANCE 38 00112 #define READ_DELAY 16 00113 #endif 00114 #endif 00115 00116 #if defined(__AVR_ATtiny45__) || defined(__AVR_ATtiny85__) 00117 #if (F_CPU == 8000000 || COMPATIBILITY_MODE) // Internal clock 00118 /* ATtiny has shorter values than Duemianove / Uno because 00119 micros() produces longer delays on ATtiny45/85 */ 00120 #define BIT_WIDTH 35 00121 #define BIT_SPACER 108 00122 #define ACCEPTANCE 35 00123 #define READ_DELAY 16 00124 #endif 00125 #endif 00126 00127 #endif 00128 00129 // Protocol symbols 00130 #define ACK 6 00131 #define NAK 21 00132 #define FAIL 0x100 00133 #define BUSY 666 00134 #define BROADCAST 124 00135 #define TO_BE_SENT 74 00136 00137 // Errors 00138 #define CONNECTION_LOST 101 00139 #define PACKETS_BUFFER_FULL 102 00140 #define MEMORY_FULL 103 00141 00142 // Maximum sending attempts before throwing CONNECTON_LOST error 00143 #define MAX_ATTEMPTS 250 00144 00145 // Packets buffer length, if full PACKET_BUFFER_FULL error is thrown 00146 #define MAX_PACKETS 10 00147 00148 // Max packet length, higher if necessary (affects memory) 00149 #define PACKET_MAX_LENGTH 50 00150 00151 struct packet { 00152 uint8_t attempts; 00153 uint8_t device_id; 00154 char *content; 00155 uint8_t length; 00156 uint32_t registration; 00157 int state; 00158 uint32_t timing; 00159 }; 00160 00161 typedef void (* receiver)(uint8_t length, uint8_t *payload); 00162 typedef void (* pjon_error)(uint8_t code, uint8_t data); 00163 00164 static void dummy_error_handler(uint8_t code, uint8_t data) { 00165 DigitalOut myled(LED1); 00166 myled = 1; 00167 }; 00168 00169 class PJON { 00170 00171 public: 00172 PJON(PinName input_pin); 00173 PJON(PinName input_pin, uint8_t id); 00174 00175 void initialize(); 00176 00177 void set_id(uint8_t id); 00178 void set_receiver(receiver r); 00179 void set_error(pjon_error e); 00180 00181 int receive_byte(); 00182 int receive(); 00183 int receive(unsigned long duration); 00184 00185 void send_bit(uint8_t VALUE, int duration); 00186 void send_byte(uint8_t b); 00187 int send_string(uint8_t id, char *string, uint8_t length); 00188 int send(uint8_t id, char *packet, uint8_t length, unsigned long timing = 0); 00189 00190 void update(); 00191 void remove(int id); 00192 00193 uint8_t read_byte(); 00194 bool can_start(); 00195 00196 uint8_t syncronization_bit(); 00197 00198 uint8_t data[PACKET_MAX_LENGTH]; 00199 packet packets[MAX_PACKETS]; 00200 00201 private: 00202 DigitalInOut _input_pin; 00203 uint8_t _device_id; 00204 //int _input_pin; 00205 receiver _receiver; 00206 pjon_error _error; 00207 Timer _reg_timer; 00208 };
Generated on Sat Jul 23 2022 04:31:54 by
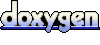