
Testing getting PJON working on mbed https://github.com/gioblu/PJON
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 #include <pjon.h> 00003 /* 00004 // network(Arduino pin used, selected device id) 00005 PJON network(p12, 1); 00006 DigitalOut myled(LED1); 00007 00008 int main() { 00009 network.send(44, "B", 1, 1000000); 00010 while(1) { 00011 network.update(); 00012 myled = 1; 00013 wait(0.2); 00014 myled = 0; 00015 wait(0.2); 00016 } 00017 } 00018 */ 00019 00020 float test; 00021 float mistakes; 00022 int busy; 00023 int fail; 00024 00025 // network(Arduino pin used, selected device id) 00026 PJON network(p21, 45); 00027 Serial serial(USBTX, USBRX); 00028 Timer timer; 00029 int packet; 00030 char content[] = "01234567890123456789"; 00031 00032 void setup() { 00033 serial.baud(19200); 00034 timer.start(); 00035 serial.printf("PJON - Network analysis\n\r"); 00036 serial.printf("Starting a 10 seconds communication test..\n\r\n\r"); 00037 } 00038 00039 void loop() { 00040 Timer t2; 00041 long time = timer.read_ms(); 00042 while(timer.read_ms() - time < 10000) { 00043 00044 /* 00045 Here send_string low level function is used to 00046 be able to catch every single sending result. 00047 */ 00048 00049 int response = network.send_string(44, content, 20); 00050 if(response == ACK) 00051 test++; 00052 if(response == NAK) 00053 mistakes++; 00054 if(response == BUSY) 00055 busy++; 00056 if(response == FAIL) 00057 fail++; 00058 wait_us(50); 00059 } 00060 00061 serial.printf("Absolute com speed: "); 00062 serial.printf("%i", (test * 24 ) / 10 ); 00063 serial.printf("B/s\n\r"); 00064 serial.printf("Practical bandwidth: "); 00065 serial.printf("%i", (test * 20 ) / 10 ); 00066 serial.printf("B/s\n\r"); 00067 serial.printf("Packets sent: "); 00068 serial.printf("%f\n\r", test); 00069 serial.printf("Mistakes (error found with CRC) "); 00070 serial.printf("%f\n\r", mistakes); 00071 serial.printf("Fail (no answer from receiver) "); 00072 serial.printf("%i\n\r", fail); 00073 serial.printf("Busy (Channel is busy or affected by interference) "); 00074 serial.printf("%i\n\r", busy); 00075 serial.printf("Accuracy: "); 00076 serial.printf("%i", 100 - (100 / (test / mistakes))); 00077 serial.printf(" %\n\r"); 00078 serial.printf(" --------------------- \n\r"); 00079 00080 test = 0; 00081 mistakes = 0; 00082 busy = 0; 00083 fail = 0; 00084 }; 00085 00086 int main(){ 00087 setup(); 00088 while(true){ 00089 loop(); 00090 } 00091 }
Generated on Sat Jul 23 2022 04:31:54 by
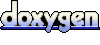