A project to implement a console using the Mbed using VGA for video output and a PS/2 keyboard for the input. The eventual goal is to also include tools for managing SD cards, and a semi-self-hosting programming environment.
Dependencies: PS2_MbedConsole fastlib SDFileSystem vga640x480g_mbedconsole lightvm mbed
main.cpp
00001 /* 00002 <Copyright Header> 00003 Copyright (c) 2012 Jordan "Earlz" Earls <http://lastyearswishes.com> 00004 All rights reserved. 00005 00006 Redistribution and use in source and binary forms, with or without 00007 modification, are permitted provided that the following conditions 00008 are met: 00009 00010 1. Redistributions of source code must retain the above copyright 00011 notice, this list of conditions and the following disclaimer. 00012 2. Redistributions in binary form must reproduce the above copyright 00013 notice, this list of conditions and the following disclaimer in the 00014 documentation and/or other materials provided with the distribution. 00015 3. The name of the author may not be used to endorse or promote products 00016 derived from this software without specific prior written permission. 00017 00018 THIS SOFTWARE IS PROVIDED ``AS IS'' AND ANY EXPRESS OR IMPLIED WARRANTIES, 00019 INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY 00020 AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL 00021 THE AUTHOR BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, 00022 EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, 00023 PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; 00024 OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 00025 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR 00026 OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF 00027 ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00028 00029 This file is part of the MbedConsole project 00030 */ 00031 00032 #include "mbedconsole.h" 00033 #include "clock.h" 00034 #include "i2s.h" 00035 #include "nvic.h" 00036 #include "SDFileSystem.h" 00037 00038 #define DEVICE_STDIO_MESSAGES 00039 #include "mbed_debug.h" 00040 00041 PS2Keyboard *ps2kb; 00042 DigitalOut myled(LED1); 00043 Serial serial(USBTX, USBRX); 00044 ConsoleStream console("console"); 00045 SDFileSystem *sdcard; 00046 00047 00048 void keyboard_init(); 00049 00050 int main() { 00051 serial.baud(9600); 00052 serial.puts("Resetting interrupt priorities..\n"); 00053 //Set all priorities to 100 so we are able to make VGA a priority 00054 //By default, all priorities are at their highest 0 00055 NVIC_SetPriority( NonMaskableInt_IRQn, 100 ); 00056 NVIC_SetPriority(MemoryManagement_IRQn, 100); 00057 00058 NVIC_SetPriority(BusFault_IRQn, 100); 00059 NVIC_SetPriority(UsageFault_IRQn, 100); 00060 NVIC_SetPriority(SVCall_IRQn, 100); 00061 NVIC_SetPriority(DebugMonitor_IRQn, 100); 00062 NVIC_SetPriority(PendSV_IRQn, 100); 00063 NVIC_SetPriority(SysTick_IRQn, 50); 00064 NVIC_SetPriority(WDT_IRQn, 100); 00065 NVIC_SetPriority(TIMER0_IRQn, 85); 00066 NVIC_SetPriority(TIMER1_IRQn, 85); 00067 NVIC_SetPriority(TIMER2_IRQn, 85); 00068 NVIC_SetPriority(TIMER3_IRQn, 85); 00069 NVIC_SetPriority(UART0_IRQn, 75); 00070 NVIC_SetPriority(UART1_IRQn, 100); 00071 NVIC_SetPriority(UART2_IRQn, 100); 00072 NVIC_SetPriority(UART3_IRQn, 100); 00073 00074 NVIC_SetPriority(PWM1_IRQn, 100); 00075 NVIC_SetPriority(I2C0_IRQn, 100); 00076 NVIC_SetPriority(I2C1_IRQn, 100); 00077 NVIC_SetPriority(I2C2_IRQn, 100); 00078 NVIC_SetPriority(SPI_IRQn, 1); 00079 NVIC_SetPriority(SSP0_IRQn, 100); 00080 NVIC_SetPriority(SSP1_IRQn, 100); 00081 NVIC_SetPriority(PLL0_IRQn, 100); 00082 NVIC_SetPriority(RTC_IRQn, 100); 00083 NVIC_SetPriority(EINT0_IRQn, 100); 00084 NVIC_SetPriority(EINT1_IRQn, 100); 00085 00086 NVIC_SetPriority(EINT2_IRQn, 100); 00087 NVIC_SetPriority(EINT3_IRQn, 100); 00088 NVIC_SetPriority(ADC_IRQn, 100); 00089 NVIC_SetPriority(BOD_IRQn, 100); 00090 NVIC_SetPriority(USB_IRQn, 100); 00091 NVIC_SetPriority(CAN_IRQn, 100); 00092 NVIC_SetPriority(DMA_IRQn, 100); 00093 00094 NVIC_SetPriority(I2S_IRQn, 100); 00095 NVIC_SetPriority(ENET_IRQn, 100); 00096 NVIC_SetPriority(RIT_IRQn, 100); 00097 NVIC_SetPriority(MCPWM_IRQn, 100); 00098 NVIC_SetPriority(QEI_IRQn, 100); 00099 NVIC_SetPriority(PLL1_IRQn, 100); 00100 init_vga(); 00101 vga_cls(); 00102 keyboard_init(); 00103 fl_select_clock_i2s(FL_CLOCK_DIV1); // assume 100MHz 00104 fl_i2s_set_tx_rate(1,4); // set 25 MHz pixel clock 00105 00106 00107 NVIC_SetPriority( EINT3_IRQn, 90 ); 00108 00109 //initialize way down here AFTER overclocking has already taken place 00110 //Otherwise we end up with sdcard assume a different clockrate or something 00111 wait(0.5); //MAGIC do not remove this wait statement. SD card won't work otherwise. 00112 sdcard=new SDFileSystem(p11, p12, p13, p20, "sd"); 00113 00114 freopen("/console", "w", stdout); 00115 setvbuf(stdout, NULL, _IONBF, 0); 00116 freopen("/console", "r", stdin); 00117 setvbuf(stdout, NULL, _IONBF, 0); 00118 //freopen("/console", "w", stderr); 00119 setvbuf(stderr, NULL, _IONBF, 0); 00120 //serial.baud(9216);//9600); 00121 00122 serial.puts("Entering main shell\n"); 00123 debug("testing... "); 00124 while(1) 00125 { 00126 vputs("mbedConsole by Jordan Earls\n"); 00127 shell_begin(); 00128 } 00129 } 00130 00131 00132 00133
Generated on Sun Jul 17 2022 09:10:09 by
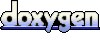