
Development and testing of ultrasonic distance measurement library for device HC-SR04.
Dependencies: TextLCD_improved mbed Distance_HC_SR04
main.cpp
00001 /* 00002 * MAIN.CPP 00003 */ 00004 #include "mbed.h" 00005 00006 #include "TextLCD.h" 00007 #include "Distance_HC_SR04.h" 00008 00009 00010 int main() { 00011 00012 TextLCD lcd(PA_8, PA_7, PA_9, PA_1, PB_5, PA_10, TextLCD::LCD16x2); 00013 00014 wait_ms(250); 00015 lcd.cls(); 00016 00017 Distance_HC_SR04 distFront(PB_9, PA_6); 00018 uint32_t ticks_us; 00019 float distance; 00020 00021 while (true) { 00022 ticks_us = distFront.measureTicks(); 00023 distance = distFront.getDistance(); 00024 00025 lcd.cls(); 00026 00027 switch (distFront.getState()) { 00028 case COMPLETED: 00029 lcd.printf("Dist.: %u", ticks_us); 00030 lcd.locate(0, 1); 00031 lcd.printf("Dist.: %.3f", distance); 00032 00033 break; 00034 00035 case TIMEOUT: 00036 lcd.printf("Dist.: ---"); 00037 lcd.locate(0, 1); 00038 lcd.printf("TIMEOUT"); 00039 00040 break; 00041 00042 case ERROR_SIG: 00043 lcd.printf("Dist.: ---"); 00044 lcd.locate(0, 1); 00045 lcd.printf("ERROR_SIG"); 00046 00047 break; 00048 00049 case OUT_OF_RANGE_MIN: 00050 lcd.printf("Dist.: ---"); 00051 lcd.locate(0, 1); 00052 lcd.printf("OUT_OF_RANGE_MIN"); 00053 00054 break; 00055 00056 case OUT_OF_RANGE_MAX: 00057 lcd.printf("Dist.: ---"); 00058 lcd.locate(0, 1); 00059 lcd.printf("OUT_OF_RANGE_MAX"); 00060 00061 break; 00062 00063 default: 00064 lcd.printf("Dist.: ---"); 00065 lcd.locate(0, 1); 00066 lcd.printf("OTHER"); 00067 00068 break; 00069 } 00070 wait_ms(100); 00071 } 00072 }
Generated on Sat Jul 16 2022 14:42:15 by
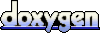