Library to control NeoPixel strings of RGB leds
Dependents: NeoPixelI2cSlave NeoPixelI2cSlave
neopixel_string.cpp
00001 #include "neopixel_string.h" 00002 00003 NeoPixelString::NeoPixelString(PinName spi_pin, unsigned int length) : PixelArray(spi_pin) { 00004 this->length = length; 00005 pixels = new neopixel::Pixel[length]; // Actual buffer of colors 00006 00007 // Clear all 00008 update(Colors::Black); 00009 } 00010 00011 unsigned int NeoPixelString::getLength(void) { 00012 return this->length; 00013 } 00014 00015 void NeoPixelString::update(void) { 00016 PixelArray::update(pixels, length); 00017 } 00018 00019 void NeoPixelString::update(neopixel::Pixel singlecolor) { 00020 for (unsigned int i = 0; i < length; i++) { 00021 pixels[i] = singlecolor; 00022 } 00023 update(); 00024 } 00025 00026 neopixel::Pixel NeoPixelString::getPixel(unsigned int i) { 00027 if (i < this->length) { 00028 return pixels[i]; 00029 } 00030 } 00031 00032 void NeoPixelString::setPixel(unsigned i, neopixel::Pixel pixel) { 00033 if (i < this->length) { 00034 pixels[i] = pixel; 00035 } 00036 } 00037 00038 void NeoPixelString::diagnose(void) { 00039 update(Colors::Black); 00040 wait(1); 00041 for (int i = 0; i < 5; i++) { 00042 update(ColorAdjuster::intensity(Colors::Red, 5)); 00043 wait(0.5); 00044 update(ColorAdjuster::intensity(Colors::Green, 5)); 00045 wait(0.5); 00046 update(ColorAdjuster::intensity(Colors::Blue, 5)); 00047 wait(0.5); 00048 } 00049 00050 update(Colors::Black); 00051 for (unsigned int i = 0; i < length; i++) { 00052 pixels[i] = ColorAdjuster::intensity(Colors::White, 5); 00053 PixelArray::update(pixels, length); 00054 wait(0.1); 00055 } 00056 }
Generated on Fri Jul 15 2022 00:27:27 by
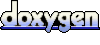