Serial output logger based on the LoggerInterface
Fork of LogIt by
serial_logger.cpp
00001 #include "serial_logger.h" 00002 #include <stdarg.h> 00003 #include <stdio.h> 00004 00005 namespace LogIt { 00006 00007 SerialLogger::SerialLogger(Serial* serial) 00008 { 00009 this->serial = serial; 00010 } 00011 00012 void SerialLogger::emergency(const char * message, ...) 00013 { 00014 va_list args; 00015 va_start (args, message); 00016 log(EMERGENCY, message, args); 00017 va_end (args); 00018 } 00019 00020 void SerialLogger::alert(const char * message, ...) 00021 { 00022 va_list args; 00023 va_start (args, message); 00024 log(ALERT, message, args); 00025 va_end (args); 00026 } 00027 00028 void SerialLogger::critical(const char * message, ...) 00029 { 00030 va_list args; 00031 va_start (args, message); 00032 log(CRITICAL, message, args); 00033 va_end (args); 00034 } 00035 00036 void SerialLogger::error(const char * message, ...) 00037 { 00038 va_list args; 00039 va_start (args, message); 00040 log(ERROR, message, args); 00041 va_end (args); 00042 } 00043 00044 void SerialLogger::warning(const char * message, ...) 00045 { 00046 va_list args; 00047 va_start (args, message); 00048 log(WARNING, message, args); 00049 va_end (args); 00050 } 00051 00052 void SerialLogger::notice(const char * message, ...) 00053 { 00054 va_list args; 00055 va_start (args, message); 00056 log(NOTICE, message, args); 00057 va_end (args); 00058 } 00059 00060 void SerialLogger::info(const char * message, ...) 00061 { 00062 va_list args; 00063 va_start (args, message); 00064 log(INFO, message, args); 00065 va_end (args); 00066 } 00067 00068 void SerialLogger::debug(const char * message, ...) 00069 { 00070 va_list args; 00071 va_start (args, message); 00072 log(DEBUG, message, args); 00073 va_end (args); 00074 } 00075 00076 void SerialLogger::log(Level level, const char * message, ...) 00077 { 00078 va_list args; 00079 va_start (args, message); 00080 log(level, message, args); 00081 va_end (args); 00082 } 00083 00084 void SerialLogger::log(Level level, const char * message, va_list args) 00085 { 00086 if(level > this->level){ 00087 return; 00088 } 00089 00090 char levelString[16]; 00091 switch(level) 00092 { 00093 case EMERGENCY: 00094 strcpy(levelString, "EMERGENCY"); 00095 break; 00096 case ALERT: 00097 strcpy(levelString, "ALERT"); 00098 break; 00099 case CRITICAL: 00100 strcpy(levelString, "CRITICAL"); 00101 break; 00102 case ERROR: 00103 strcpy(levelString, "ERROR"); 00104 break; 00105 case WARNING: 00106 strcpy(levelString, "WARNING"); 00107 break; 00108 case NOTICE: 00109 strcpy(levelString, "NOTICE"); 00110 break; 00111 case INFO: 00112 strcpy(levelString, "INFO"); 00113 break; 00114 case DEBUG: 00115 strcpy(levelString, "DEBUG"); 00116 break; 00117 default: 00118 strcpy(levelString, "DEBUG"); 00119 break; 00120 } 00121 00122 char buffer[256]; 00123 vsprintf (buffer, message, args); 00124 serial->printf("%9s: %s\r\n", levelString, buffer); 00125 } 00126 00127 void SerialLogger::setLevel(Level level) 00128 { 00129 this->level = level; 00130 } 00131 00132 };
Generated on Thu Jul 14 2022 14:54:23 by
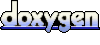