
RTC hard code
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 SPI spi(PTD6, PTD7, PTD5); // mosi, miso, sclk 00003 DigitalOut cs(PTD2); 00004 00005 00006 int main() 00007 { 00008 00009 00010 00011 // Setup the spi for 8 bit data, high steady state clock, 00012 // second edge capture, with a 1MHz clock rate 00013 spi.format(8,3); 00014 spi.frequency(1000000); 00015 00016 // Send the commands to write to test the seconds register 00017 cs = 0; 00018 spi.write(0x80); // set write register to seconds 00019 spi.write(0x00); // send value of one 00020 00021 cs=1; 00022 cs=0; 00023 spi.write(0x81); //write reg to minutes 00024 spi.write(0x00); 00025 00026 cs=1; 00027 cs=0; 00028 00029 spi.write(0x82); //write reg to minutes 00030 spi.write(0x01); 00031 00032 cs=1; 00033 00034 cs = 0; 00035 spi.write(0x83); // set write register to seconds 00036 spi.write(0x01); // send value of one 00037 00038 cs=1; 00039 00040 cs = 0; 00041 spi.write(0x84); // set write register to seconds 00042 spi.write(0x01); // send value of one 00043 00044 cs=1; 00045 00046 cs = 0; 00047 spi.write(0x85); // set write register to seconds 00048 spi.write(0x01); // send value of one 00049 00050 cs=1; 00051 00052 cs = 0; 00053 spi.write(0x86); // set write register to seconds 00054 spi.write(0x01); // send value of one 00055 00056 cs=1; 00057 // Receive the contents of the seconds register 00058 00059 for(int i=0;i<1000000;i++){ 00060 00061 00062 cs=0; 00063 spi.write(0x00); // set read register to seconds 00064 int seconds = spi.write(0x00); // read the value 00065 00066 cs=1; 00067 cs=0; 00068 00069 spi.write(0x01); 00070 int minutes =spi.write(0x00); 00071 00072 00073 cs=1; 00074 cs=0; 00075 00076 spi.write(0x02); 00077 int hours =spi.write(0x01); 00078 cs = 1; //This cs=1 is to make the chipselect line high to "deselect" the slave in our case RTC 00079 00080 cs=0; 00081 00082 spi.write(0x03); 00083 int day =spi.write(0x01); 00084 cs = 1; 00085 00086 cs=0; 00087 00088 spi.write(0x04); 00089 int date =spi.write(0x01); 00090 cs = 1; 00091 00092 cs=0; 00093 00094 spi.write(0x05); 00095 int month =spi.write(0x01); 00096 cs = 1; 00097 00098 cs=0; 00099 00100 spi.write(0x06); 00101 int year =spi.write(0x01); 00102 cs = 1; 00103 00104 wait(1); 00105 //This printf function is to check the timestamp function in the terminal output 00106 printf("Seconds register = %2X : %2X : %2X : %2X : %2X : %2X : %2X \n\r",year,month,date,day, hours,minutes,seconds); 00107 } 00108 00109 return 0; 00110 00111 00112 00113 }
Generated on Tue Aug 9 2022 02:27:41 by
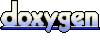