
RTC DS3234 library
Fork of WDtester by
Embed:
(wiki syntax)
Show/hide line numbers
DS3234.cpp
00001 #include <mbed.h> 00002 #include "DS3234.h" 00003 00004 /* 00005 The below mentioned are the bits in Read mode. 00006 seconds 0x80 00007 minuntes 0x81 00008 hours 0x82 00009 day 0x83 00010 date 0x84 00011 month 0x85 00012 year 0x86 00013 */ 00014 00015 void set(PinName pin, struct ts t) 00016 { 00017 SPI spi(PTD6, PTD7, PTD5); 00018 uint8_t i, century; 00019 00020 if (t.year > 2000) { 00021 century = 0x80; 00022 t.year_s = t.year - 2000; 00023 } else { 00024 century = 0; 00025 t.year_s = t.year - 1900; 00026 } 00027 00028 uint8_t TimeDate[7] = { t.sec, t.min, t.hour, t.wday, t.mday, t.mon, t.year_s }; 00029 for (i = 0; i <= 6; i++) { 00030 00031 DigitalOut cs(pin); 00032 cs = 0; 00033 spi.write(i + 0x80); 00034 if (i == 5) 00035 spi.write(dectobcd(TimeDate[5]) + century); 00036 else 00037 spi.write(dectobcd(TimeDate[i])); 00038 cs = 1; 00039 } 00040 00041 } 00042 void DS3234_get(PinName pin, struct ts *t) 00043 { 00044 SPI spi(PTD6, PTD7, PTD5); 00045 uint8_t TimeDate[7]; //second,minute,hour,dow,day,month,year 00046 uint8_t century = 0; 00047 uint8_t i, n; 00048 uint16_t year_full; 00049 DigitalOut cs(pin); 00050 00051 for (i = 0; i <= 6; i++) { 00052 cs = 0; 00053 spi.write(i + 0x00); 00054 n = spi.write(0x00); 00055 00056 if (i == 5) { // month address also contains the century on bit7 00057 TimeDate[5] = bcdtodec(n & 0x1F); 00058 century = (n & 0x80) >> 7; 00059 } else { 00060 TimeDate[i] = bcdtodec(n); 00061 } 00062 } 00063 00064 if (century == 1) 00065 year_full = 2000 + TimeDate[6]; 00066 else 00067 year_full = 1900 + TimeDate[6]; 00068 00069 t->sec = TimeDate[0]; 00070 t->min = TimeDate[1]; 00071 t->hour = TimeDate[2]; 00072 t->mday = TimeDate[4]; 00073 t->mon = TimeDate[5]; 00074 t->year = year_full; 00075 t->wday = TimeDate[3]; 00076 t->year_s = TimeDate[6]; 00077 } 00078 00079 00080 00081 uint8_t dectobcd(const uint8_t val) 00082 { 00083 return ((val / 10 * 16) + (val % 10)); 00084 } 00085 00086 uint8_t bcdtodec(const uint8_t val) 00087 { 00088 return ((val / 16 * 10) + (val % 16)); 00089 }
Generated on Wed Jul 27 2022 17:33:39 by
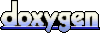