driver for sx1280
Dependents: alarm_slave_extended_SX1280 alarm_master_extended_Vance_SX1280
sx12xx.h
00001 #include "mbed.h" 00002 #ifndef SX128x_H 00003 #define SX128x_H 00004 00005 /***************************************************************/ 00006 #define OPCODE_GET_PACKET_TYPE 0x03 00007 #define OPCODE_CLEAR_DEVICE_ERRORS 0x07 00008 #define OPCODE_GET_IRQ_STATUS 0x15 00009 #define OPCODE_GET_RX_BUFFER_STATUS 0x17 00010 #define OPCODE_WRITE_REGISTER 0x18 00011 #define OPCODE_READ_REGISTER 0x19 00012 #define OPCODE_WRITE_BUFFER 0x1a 00013 #define OPCODE_READ_BUFFER 0x1b 00014 #define OPCODE_GET_PACKET_STATUS 0x1d 00015 #define OPCODE_GET_RSSIINST 0x1f 00016 #define OPCODE_SET_STANDBY 0x80 00017 #define OPCODE_SET_RX 0x82 00018 #define OPCODE_SET_TX 0x83 00019 #define OPCODE_SET_SLEEP 0x84 00020 #define OPCODE_SET_RF_FREQUENCY 0x86 00021 #define OPCODE_SET_CAD_PARAM 0x88 00022 #define OPCODE_CALIBRATE 0x89 00023 #define OPCODE_SET_PACKET_TYPE 0x8a 00024 #define OPCODE_SET_MODULATION_PARAMS 0x8b 00025 #define OPCODE_SET_PACKET_PARAMS 0x8c 00026 #define OPCODE_SET_DIO_IRQ_PARAMS 0x8d 00027 #define OPCODE_SET_TX_PARAMS 0x8e 00028 #define OPCODE_SET_BUFFER_BASE_ADDR 0x8f 00029 #define OPCODE_SET_PA_CONFIG 0x95 00030 #define OPCODE_SET_REGULATOR_MODE 0x96 00031 #define OPCODE_CLEAR_IRQ_STATUS 0x97 00032 #define OPCODE_SET_RANGING_ROLE 0xa3 00033 #define OPCODE_GET_STATUS 0xc0 00034 #define OPCODE_SET_FS 0xc1 00035 #define OPCODE_SET_CAD 0xc5 00036 #define OPCODE_SET_TX_CARRIER 0xd1 00037 #define OPCODE_SET_TX_PREAMBLE 0xd2 00038 #define OPCODE_SAVE_CONTEXT 0xd5 00039 /***************************************************************/ 00040 #define REG_ADDR_AFIRQ 0x053 // 7bit 00041 #define REG_ADDR_RXBW 0x881 // 7bit 00042 #define REG_ADDR_GFSK_DEMODSTATUS 0x8cb // 5bit 00043 #define REG_ADDR_LORA_TX_PAYLOAD_LENGTH 0x901 // 8bit 00044 #define REG_ADDR_LORA_PKTPAR0 0x902 // 8bit 00045 #define REG_ADDR_LORA_PKTPAR1 0x903 // 8bit 00046 #define REG_ADDR_LORA_LRCTL 0x904 // 8bit 00047 #define REG_ADDR_LORA_SD_FREQ 0x906 // 24bit 00048 #define REG_ADDR_LORA_IRQMASK 0x90f // 24bit 00049 #define REG_ADDR_LORA_MASTER_REQ_ID 0x912 // 32bit 00050 #define REG_ADDR_LORA_SLAVE_ID 0x916 // 32bit 00051 #define REG_ADDR_RNGFLTWNDSIZE 0x91e // 32bit 00052 #define REG_ADDR_RNGCFG0 0x923 // 8bit 00053 #define REG_ADDR_RNGCFG1 0x924 // 7bit 00054 #define REG_ADDR_LORA_FE_GAIN 0x929 // 8bit 00055 #define REG_ADDR_LORA_DELAY_CAL 0x92b // 24bit 00056 #define REG_ADDR_LORA_RNGDEBTH2 0x931 // 8bit 00057 #define REG_ADDR_RNGDEBTH4H 0x935 // 8bit 00058 00059 #define REG_ADDR_LORA_PREAMBLE 0x93f // 8bit 00060 #define REG_ADDR_LORA_SYNC 0x944 // LrCfg7, LrCfg8 post-preamble gap (AKA lora sync) 00061 #define REG_ADDR_LORA_MODEMSTAT 0x95c // 8bit 00062 #define REG_ADDR_RNGRESULT 0x961 // 24bit 00063 #define REG_ADDR_RNGRSSI 0x964 // 8bit 00064 00065 #define REG_ADDR_FSK_CFG 0x9a0 // 8bit 00066 #define REG_ADDR_FSK_MODDFH 0x9a1 // 8bit 00067 #define REG_ADDR_FSK_MODDFL 0x9a2 // 8bit 00068 #define REG_ADDR_RFFREQ 0x9a3 // 24bit 00069 00070 #define REG_ADDR_PKTCTRL0 0x9c0 // 8bit 00071 #define REG_ADDR_PKTCTRL1 0x9c1 // 8bit 00072 #define REG_ADDR_PKT_TX_HEADER 0x9c2 // 8bit 00073 #define REG_ADDR_PAYLOAD_LEN 0x9c3 // 8bit 00074 #define REG_ADDR_PKT_BITSTREAM_CTRL 0x9c4 // 8bit 00075 #define REG_ADDR_FLORA_PREAMBLE_HI 0x9ca // 8bit contains data rate 00076 #define REG_ADDR_PKT_SYNC_ADRS_CTRL 0x9cd // 8bit 00077 #define REG_ADDR_PKT_SYNC_ADRS_1 0x9ce // 40bit / 5bytes 00078 #define REG_ADDR_PKT_SYNC_ADRS_2 0x9d3 // 40bit / 5bytes 00079 #define REG_ADDR_PKT_SYNC_ADRS_3 0x9d8 // 40bit / 5bytes 00080 00081 #define REG_ADDR_FLRC_SYNCWORDCTRL 0x98b // 5bit 00082 #define REG_ADDR_FLRC_IRQSTATUS 0x990 // 3bit 00083 #define REG_ADDR_XTA_TRIM 0xa0e // crystal trim 00084 #define REG_ADDR_XTB_TRIM 0xa0f // crystal trim 00085 #define REG_ADDR_PA_PWR_CTRL 0xa53 // 8bit 00086 /***************************************************************/ 00087 #define PLL_STEP_HZ 198.364257812 // 52MHz / 2^18 00088 #define PLL_STEP_MHZ 0.000198364257812 // 52 / 2^18 00089 00090 #define RX_CONTINUOUS_TIMEOUT 0xffff // receive forever 00091 #define RX_SINGLE_TIMEOUT 0x0000 // receive until packet received 00092 00093 #define PACKET_TYPE_GFSK 0 00094 #define PACKET_TYPE_LORA 1 00095 #define PACKET_TYPE_RANGING 2 00096 #define PACKET_TYPE_FLRC 3 00097 #define PACKET_TYPE_BLE 4 00098 00099 00100 #define RADIO_PACKET_FIXED_LENGTH 0x00 00101 #define RADIO_PACKET_VARIABLE_LENGTH 0x20 00102 00103 // birate & bandwidth modParam1: 00104 #define GFSK_BLE_BR_2_000_BW_2_4 0x04 // Mbps:2 bw:2.4MHz 0000 0100 00105 #define GFSK_BLE_BR_1_600_BW_2_4 0x28 // Mbps:1.6 bw:2.4MHz 0010 1000 00106 #define GFSK_BLE_BR_1_000_BW_2_4 0x4C // Mbps:1 bw:2.4MHz 0100 1100 00107 #define GFSK_BLE_BR_1_000_BW_1_2 0x45 // Mbps:1 bw:1.2MHz 0100 0101 00108 #define GFSK_BLE_BR_0_800_BW_2_4 0x70 // Mbps:0.8 bw:2.4MHz 0111 0000 00109 #define GFSK_BLE_BR_0_800_BW_1_2 0x69 // Mbps:0.8 bw:1.2MHz 0110 1001 00110 #define GFSK_BLE_BR_0_500_BW_1_2 0x8D // Mbps:0.5 bw:1.2MHz 1000 1101 00111 #define GFSK_BLE_BR_0_500_BW_0_6 0x86 // Mbps:0.5 bw:0.6MHz 1000 0110 00112 #define GFSK_BLE_BR_0_400_BW_1_2 0xB1 // Mbps:0.4 bw:1.2MHz 1011 0001 00113 #define GFSK_BLE_BR_0_400_BW_0_6 0xAA // Mbps:0.4 bw:0.6MHz 1010 1010 00114 #define GFSK_BLE_BR_0_250_BW_0_6 0xCE // Mbps:0.25 bw:0.6MHz 1100 1110 00115 #define GFSK_BLE_BR_0_250_BW_0_3 0xC7 // Mbps:0.25 bw:0.3MHz 1100 0111 00116 #define GFSK_BLE_BR_0_125_BW_0_3 0xEF // Mbps:0.125 bw:0.3MHz 1110 1111 00117 00118 // modulationIndex modParam2 00119 #define MOD_IND_0_35 0x00 // 0.35 00120 #define MOD_IND_0_5 0x01 // 0.5 00121 #define MOD_IND_0_75 0x02 // 0.75 00122 #define MOD_IND_1_00 0x03 // 1 00123 #define MOD_IND_1_25 0x04 // 1.25 00124 #define MOD_IND_1_50 0x05 // 1.5 00125 #define MOD_IND_1_75 0x06 // 1.75 00126 #define MOD_IND_2_00 0x07 // 2 00127 #define MOD_IND_2_25 0x08 // 2.25 00128 #define MOD_IND_2_50 0x09 // 2.5 00129 #define MOD_IND_2_75 0x0A // 2.75 00130 #define MOD_IND_3_00 0x0B // 3 00131 #define MOD_IND_3_25 0x0C // 3.25 00132 #define MOD_IND_3_50 0x0D // 3.5 00133 #define MOD_IND_3_75 0x0E // 3.75 00134 #define MOD_IND_4_00 0x0F // 4 00135 00136 // modulationShaping modParam3: 00137 #define BT_OFF 0x00 // No filtering 00138 #define BT_1_0 0x10 // BT1 00139 #define BT_0_5 0x20 // BT0.5 00140 00141 // packetParam6: 00142 #define RADIO_CRC_OFF 0x00 // CRC off 00143 #define RADIO_CRC_1_BYTES 0x10 // CRC field used 1 byte 00144 #define RADIO_CRC_2_BYTES 0x20 // CRC field uses 2 bytes 00145 #define RADIO_CRC_3_BYTES 0x30 // ??? CRC field uses 3 bytes FLRC ???? 00146 00147 // GFSK packetParam7: 00148 #define WHITENING_ENABLE 0x00 00149 #define WHITENING_DISABLE 0x08 00150 00151 // LoRa spreadingFactor modParam1: 00152 #define LORA_SF_5 0x50 00153 #define LORA_SF_6 0x60 00154 #define LORA_SF_7 0x70 00155 #define LORA_SF_8 0x80 00156 #define LORA_SF_9 0x90 00157 #define LORA_SF_10 0xA0 00158 #define LORA_SF_11 0xB0 00159 #define LORA_SF_12 0xC0 00160 00161 // LoRa modParam2 00162 #define LORA_BW_1600 0x0A // 1625.0KHz 00163 #define LORA_BW_800 0x18 // 812.5KHz 00164 #define LORA_BW_400 0x26 // 406.25KHz 00165 #define LORA_BW_200 0x34 // 203.125KHz 00166 #define LORA_BW_100 0x42 // 00167 #define LORA_BW_50 0x51 // 00168 00169 // LoRa modParam3 00170 #define LORA_CR_4_5 0x01 // 4/5 00171 #define LORA_CR_4_6 0x02 // 4/6 00172 #define LORA_CR_4_7 0x03 // 4/7 00173 #define LORA_CR_4_8 0x04 // 4/8 00174 #define LORA_CR_LI_4_5 0x05 // 4/5* 00175 #define LORA_CR_LI_4_6 0x06 // 4/6* 00176 #define LORA_CR_LI_4_7 0x07 // 4/8* 00177 00178 // LoRa packetParam2: 00179 #define EXPLICIT_HEADER 0x00 // var length 00180 #define IMPLICIT_HEADER 0x80 // fixed Length 00181 00182 // LoRa packetParam4: 00183 #define LORA_CRC_ENABLE 0x20 00184 #define LORA_CRC_DISABLE 0x00 00185 00186 // LoRa packetParam5 00187 #define LORA_IQ_STD 0x40 // IQ as defined 00188 #define LORA_IQ_INVERTED 0x00 // IQ swapped 00189 00190 // transmitter power ramping 00191 #define RADIO_RAMP_02_US 0x00 // 2 microseconds 00192 #define RADIO_RAMP_04_US 0x20 // 4 microseconds 00193 #define RADIO_RAMP_06_US 0x40 // 6 microseconds 00194 #define RADIO_RAMP_08_US 0x60 // 8 microseconds 00195 #define RADIO_RAMP_10_US 0x80 // 10 microseconds 00196 #define RADIO_RAMP_12_US 0xA0 // 12 microseconds 00197 #define RADIO_RAMP_16_US 0xC0 // 16 microseconds 00198 #define RADIO_RAMP_20_US 0xE0 // 20 microseconds 00199 00200 // BLE packetParam1 00201 #define BLE_PAYLOAD_LENGTH_MAX_31_BYTES 0x00 // maxlen:31 Bluetooth® 4.1 and above 00202 #define BLE_PAYLOAD_LENGTH_MAX_37_BYTES 0x20 // maxlen:37 Bluetooth® 4.1 and above 00203 #define BLE_TX_TEST_MODE 0x40 // maxlen:63 Bluetooth® 4.1 and above 00204 #define BLE_PAYLOAD_LENGTH_MAX_255_BYTES 0x80 // maxlen:255 Bluetooth® 4.2 and above 00205 00206 // BLE packetParam2 00207 #define BLE_CRC_OFF 0x00 // No CRC No 00208 #define BLE_CRC_3B 0x10 // CRC field used 3bytes Yes 00209 00210 // BLE packetParam3 ignored in BLE_TX_TEST_MODE 00211 #define BLE_PRBS_9 0x00// P7(x) = x9+ x5+ 1 PRBS9 sequence ‘1111111110000011110 1....’ (in transmission order) 00212 #define BLE_EYELONG_1_0 0x04 // Repeated ‘11110000’ (in transmission order) sequence 00213 #define BLE_EYESHORT_1_0 0x08 // Repeated ‘10101010’ (in transmission order) sequence 00214 #define BLE_PRBS_15 0x0C // Pseudo Random Binary Sequence based on 15th degree polynomial P15(x) = x15+ x14+ x13 + x12+ x2+ x + 1 00215 #define BLE_ALL_1 0x10 // Repeated ‘11111111’ (in transmission order) sequence 00216 #define BLE_ALL_0 0x14 // Repeated ‘11111111’ (in transmission order) sequence 00217 #define BLE_EYELONG_0_1 0x18 // Repeated ‘00001111’ (in transmission order) sequence 00218 #define BLE_EYESHORT_0_1 0x1C // Repeated ‘01010101’ (in transmission order) sequence 00219 00220 // packetParam1 00221 #define PREAMBLE_LENGTH_4_BITS 0x00 // 4bits 00222 #define PREAMBLE_LENGTH_8_BITS 0x10 // 8bits 00223 #define PREAMBLE_LENGTH_12_BITS 0x20 // 12bits 00224 #define PREAMBLE_LENGTH_16_BITS 0x30 // 16bits 00225 #define PREAMBLE_LENGTH_20_BITS 0x40 // 20bits 00226 #define PREAMBLE_LENGTH_24_BITS 0x50 // 24bits 00227 #define PREAMBLE_LENGTH_28_BITS 0x60 // 28bits 00228 #define PREAMBLE_LENGTH_32_BITS 0x70 // 32bits 00229 00230 // FLRC pktParam2 00231 #define FLRC_SYNC_NOSYNC 0x00 // 21 bits preamble 00232 #define FLRC_SYNC_WORD_LEN_P32S 0x04 // 21 bits preamble + 32bit syncword 00233 00234 // FLRC modParam1 00235 #define FLRC_BR_1_300_BW_1_2 0x45 // 1.3Mb/s 1.2MHz 00236 #define FLRC_BR_1_000_BW_1_2 0x69 // 1.04Mb/s 1.2MHz 00237 #define FLRC_BR_0_650_BW_0_6 0x86 // 0.65Mb/s 0.6MHz 00238 #define FLRC_BR_0_520_BW_0_6 0xAA // 0.52Mb/s 0.6MHz 00239 #define FLRC_BR_0_325_BW_0_3 0xC7 // 0.325Mb/s 0.3MHz 00240 #define FLRC_BR_0_260_BW_0_3 0xEB // 0.26Mb/s 0.3MHz 00241 00242 // FLRC modParam2 00243 #define FLRC_CR_1_2 0x00 00244 #define FLRC_CR_3_4 0x02 00245 #define FLRC_CR_1_0 0x04 00246 00247 typedef enum { 00248 STDBY_RC = 0, // Device running on RC 13MHz, set STDBY_RC mode 00249 STDBY_XOSC // Device running on XTAL 52MHz, set STDBY_XOSC mode 00250 } stby_t; 00251 00252 typedef union { 00253 struct { 00254 uint8_t PreambleLength; // param1 00255 uint8_t HeaderType; // param2 00256 uint8_t PayloadLength; // param3 00257 uint8_t crc; // param4 00258 uint8_t InvertIQ; // param5 00259 uint8_t unused[2]; 00260 } lora; 00261 struct { 00262 uint8_t PreambleLength; // param1 00263 uint8_t SyncWordLength; // param2 bytes 00264 uint8_t SyncWordMatch; // param3 00265 uint8_t HeaderType; // param4 00266 uint8_t PayloadLength; // param5 00267 uint8_t CRCLength; // param6 00268 uint8_t Whitening; // param7 00269 } gfskFLRC; 00270 struct { 00271 uint8_t ConnectionState; // param1 00272 uint8_t CrcLength; // param2 00273 uint8_t BleTestPayload; // param3 00274 uint8_t Whitening; // param4 00275 uint8_t unused[3]; 00276 } ble; 00277 uint8_t buf[7]; 00278 } PacketParams_t; 00279 00280 //TODO -- uint8_t LowDatarateOptimize; // param4 00281 typedef union { 00282 struct { 00283 uint8_t spreadingFactor; // param1 00284 uint8_t bandwidth; // param2 00285 uint8_t codingRate; // param3 00286 } lora; 00287 struct { 00288 uint8_t bitrateBandwidth; // param1 00289 uint8_t ModulationIndex; // param1 00290 uint8_t ModulationShaping; // param3 00291 } gfskBle; 00292 struct { 00293 uint8_t bitrateBandwidth; // param1 00294 uint8_t CodingRate; // param1 00295 uint8_t ModulationShaping; // param3 00296 } flrc; 00297 uint8_t buf[3]; 00298 } ModulationParams_t; 00299 00300 typedef union { 00301 struct { // 00302 uint16_t TxDone : 1; // 0 00303 uint16_t RxDone : 1; // 1 00304 uint16_t SyncWordValid: 1; // 2 00305 uint16_t SyncWordError: 1; // 3 00306 uint16_t HeaderValid: 1; // 4 00307 uint16_t HeaderError: 1; // 5 00308 uint16_t CrcError: 1; // 6 00309 uint16_t RangingSlaveResponseDone: 1; // 7 00310 uint16_t RangingSlaveRequestDiscard: 1; // 8 00311 uint16_t RangingMasterResultValid: 1; // 9 00312 uint16_t RangingMasterTimeout: 1; // 10 00313 uint16_t RangingMasterRequestValid: 1; // 11 00314 uint16_t CadDone: 1; // 12 00315 uint16_t CadDetected: 1; // 13 00316 uint16_t RxTxTimeout: 1; // 14 00317 uint16_t PreambleDetected: 1; // 15 00318 } bits; 00319 uint16_t word; 00320 } IrqFlags_t; 00321 00322 typedef union { 00323 struct { // 00324 uint8_t busy : 1; // 0 00325 uint8_t reserved : 1; // 1 00326 uint8_t cmdStatus : 3; // 2,3,4 00327 uint8_t chipMode : 3; // 5,6,7 00328 } bits; 00329 uint8_t octet; 00330 } status_t; 00331 00332 typedef enum { 00333 CHIPMODE_NONE = 0, 00334 CHIPMODE_RX, 00335 CHIPMODE_TX 00336 } chipMode_e; 00337 00338 typedef union { 00339 struct { 00340 uint8_t dataRAM : 1; // 0 00341 uint8_t dataBuffer : 1; // 1 00342 uint8_t instructionRAM : 1; // 2 00343 } retentionBits; 00344 uint8_t octet; 00345 } sleepConfig_t; 00346 00347 00348 typedef union { 00349 struct { 00350 status_t status; 00351 uint8_t rfu; 00352 uint8_t rssiSync; 00353 struct { 00354 uint8_t pktCtrlBusy : 1; // 0 00355 uint8_t packetReceived : 1; // 1 00356 uint8_t headerReceived : 1; // 2 00357 uint8_t AbortErr : 1; // 3 00358 uint8_t CrcError : 1; // 4 00359 uint8_t LengthError : 1; // 5 00360 uint8_t SyncError : 1; // 6 00361 uint8_t reserved : 1; // 7 00362 } errors; 00363 struct { 00364 uint8_t pktSent : 1; // 0 00365 uint8_t res41 : 4; // 1,2,3,4 00366 uint8_t rxNoAck : 1; // 5 00367 uint8_t res67 : 1; // 6,7 00368 } pkt_status; 00369 struct { 00370 uint8_t syncAddrsCode : 3; // 0,1,2 00371 uint8_t reserved : 5; // 3,4,5,6,7 00372 } sync; 00373 } ble_gfsk_flrc; 00374 struct { 00375 status_t status; 00376 uint8_t rssiSync; 00377 uint8_t snr; 00378 uint8_t reserved[3]; 00379 } lora; 00380 uint8_t buf[6]; 00381 } pktStatus_t; 00382 00383 /**********************************************************/ 00384 00385 typedef union { 00386 struct { 00387 uint8_t irq0 : 2; // 0,1 00388 uint8_t irq1 : 2; // 2,3 00389 uint8_t alt_func : 4; // 4,5,6,7 00390 } bits; 00391 uint8_t octet; 00392 } RegAfIrq_t; // 0x053 00393 00394 typedef union { 00395 struct { 00396 uint8_t dig_fe_en : 1; // 0 00397 uint8_t zero_if : 1; // 1 00398 uint8_t if_sel : 1; // 2 00399 uint8_t lora_mode : 1; // 3 00400 uint8_t res : 4; // 4,5,6,7 00401 } bits; 00402 uint8_t octet; 00403 } RegCfg_t; // 0x880 00404 00405 typedef union { 00406 struct { 00407 uint8_t bw : 3; // 0,1,2 00408 uint8_t res3 : 1; // 3 00409 uint8_t interp_factor : 1; // 4,5,6 00410 uint8_t res7 : 1; // 7 00411 } bits; 00412 uint8_t octet; 00413 } RegRxBw_t; // 0x881 00414 00415 typedef union { 00416 struct { 00417 uint8_t DccBw : 2; // 0,1 00418 uint8_t DccInit : 1; // 2 00419 uint8_t IgnoreAutoDccRestart : 1; // 3 00420 uint8_t DccForce : 1; // 4 00421 uint8_t DccBypass : 1; // 5 00422 uint8_t DccFreeze : 1; // 6 00423 uint8_t res7 : 1; // 7 00424 } bits; 00425 uint8_t octet; 00426 } RegDcc_t; // 0x882 00427 00428 typedef union { 00429 struct { 00430 uint8_t SyncAdrsIrqStatus : 1; // 0 00431 uint8_t PreambleIrqStatus : 1; // 1 00432 uint8_t dtb_pa2sel_sel : 2; // 2,3 00433 uint8_t db_out_sel : 1; // 4 00434 uint8_t reserved : 3; // 5,6,7 00435 } bits; 00436 uint8_t octet; 00437 } GfskDemodStatus_t; // 0x8cb 00438 00439 typedef union { 00440 struct { 00441 uint8_t cont_rx : 1; // 0 00442 uint8_t modem_bw : 3; // 1,2,3 00443 uint8_t modem_sf : 4; // 4,5,6,7 00444 } bits; 00445 uint8_t octet; 00446 } LoRaPktPar0_t; // 0x902 00447 00448 typedef union { 00449 struct { 00450 uint8_t coding_rate : 3; // 0,1,2 00451 uint8_t ppm_offset : 2; // 3,4 00452 uint8_t tx_mode : 1; // 5 00453 uint8_t rxinvert_iq : 1; // 6 00454 uint8_t implicit_header : 1; // 7 00455 } bits; 00456 uint8_t octet; 00457 } LoRaPktPar1_t; // 0x903 00458 00459 typedef union { 00460 struct { 00461 uint8_t cadrxtx : 2; // 0,1 00462 uint8_t sd_en : 1; // 2 00463 uint8_t modem_en : 1; // 3 00464 uint8_t lora_register_clear : 1; // 4 00465 uint8_t crc_en : 1; // 5 00466 uint8_t fine_sync_en : 1; // 6 00467 uint8_t sd_force_tx_mode : 1; // 7 00468 } bits; 00469 uint8_t octet; 00470 } LoRaLrCtl_t; // 0x904 00471 00472 00473 typedef union { 00474 struct { 00475 uint8_t ranging_res_bits : 2; // 0,1 00476 uint8_t ranging_resp_en : 1; // 2 slave enable 00477 uint8_t timing_synch_en : 1; // 3 00478 uint8_t ranging_synched_start_en : 1; // 4 00479 uint8_t ranging_result_clear_reg : 1; // 5 00480 uint8_t rx_fifo_addr_clear : 1; // 6 00481 uint8_t counters_clear_reg : 1; // 7 00482 } bits; 00483 uint8_t octet; 00484 } RngCfg0_t; // 0x923 00485 00486 typedef union { 00487 struct { 00488 uint8_t muxed_counter_select : 4; // 0,1,2,3 00489 uint8_t ranging_result_mux_sel : 2; // 4,5 00490 uint8_t ranging_result_trigger_sel : 1; // 6 00491 uint8_t res : 1; // 7 00492 } bits; 00493 uint8_t octet; 00494 } RngCfg1_t; // 0x924 00495 00496 typedef union { 00497 struct { 00498 uint8_t ranging_filter_debias_th2 : 6; // 0,1,2,3,4,5 00499 uint8_t ranging_id_check_length : 2; // 6,7 00500 } bits; 00501 uint8_t octet; 00502 } RngDebTh2_t; // 0x931 00503 00504 typedef union { 00505 struct { 00506 uint8_t debias_th4 : 2; // 0,1 00507 uint8_t rng_rssi_threshold : 6; // 2...7 00508 } bits; 00509 uint8_t octet; 00510 } RngDebTh4H_t; // 0x935 00511 00512 typedef union { 00513 struct { 00514 uint8_t preamble_symb1_nb : 4; // 0,1,2,3 00515 uint8_t preamble_symb_nb_exp : 4; // 4,5,6,7 00516 } bits; 00517 uint8_t octet; 00518 } LoRaPreambleReg_t; // 0x93f 00519 00520 typedef union { 00521 struct { 00522 uint8_t rx_state : 4; // 0,1,2,3 00523 uint8_t tx_state : 3; // 4,5,6 00524 uint8_t tx_ranging_on : 1; // 7 00525 } bits; 00526 uint8_t octet; 00527 } LoRaModemStat_t; // 0x95c 00528 00529 typedef union { 00530 struct { 00531 uint8_t mod_on : 1; // 0 00532 uint8_t sd_on : 1; // 1 00533 uint8_t mod_mode : 1; // 2 0=gfsk 1=flora 00534 uint8_t tx_mode : 1; // 3 00535 uint8_t gf_bt : 2; // 4,5 0=noFilter 1=BT1.0 2=BT0.5 3=BT0.3 00536 uint8_t rxtxn : 1; // 6 0=tx 1=rx 00537 uint8_t cal_en : 1; // 7 00538 } bits; 00539 uint8_t octet; 00540 } FskCfg_t; // 0x9a0 00541 00542 typedef union { 00543 struct { 00544 uint8_t freqDev : 5; // 0,1,2,3,4 00545 uint8_t sd_in_lsb : 1; // 5 00546 uint8_t disable_dclk : 1; // 7 00547 } bits; 00548 uint8_t octet; 00549 } FskModDfH_t; // 0x9a1 00550 00551 typedef union { 00552 struct { 00553 uint8_t pkt_ctrl_on : 1; // 0 00554 uint8_t pkt_start_p : 1; // 1 0=idle 1=busy 00555 uint8_t pkt_abort_p : 1; // 2 00556 uint8_t pkt_software_clear_p : 1; // 3 software reset pulse 00557 uint8_t pkt_rx_ntx : 1; // 4 0=tx 1=rx 00558 uint8_t pkt_len_format : 1; // 5 0=fixed 1=dynamic 00559 uint8_t pkt_protocol : 2; // 6,7 1=flora 2=ble 3=generic 00560 } bits; 00561 uint8_t octet; 00562 } PktCtrl0_t; // 0x9c0 00563 00564 typedef union { 00565 struct { 00566 uint8_t infinite_preamble : 1; // 0 00567 uint8_t sync_adrs_len : 3; // 1,2,3 bytes = sync_adrs_len + 1 00568 uint8_t preamble_len : 3; // 4,5,6 bits = (preamble_len*4) + 4 00569 uint8_t preamble_det_on : 1; // 7 00570 } gfsk; 00571 struct { 00572 uint8_t reserved : 1; // 0 00573 uint8_t sync_adrs_len : 2; // 1,2 0=preamble only 1=preamble+16bitSync 2=preamble+32bitSync 00574 uint8_t reserved3 : 1; // 3 00575 uint8_t agc_preamble_len : 3; // 4,5,6 00576 uint8_t reserved7 : 1; // 7 00577 } flrc; 00578 uint8_t octet; 00579 } PktCtrl1_t; // 0x9c1 00580 00581 typedef union { 00582 struct { 00583 uint8_t payload_len_N : 1; // 0 00584 uint8_t tx_reserved : 4; // 1,2,3,4 00585 uint8_t tx_no_ack : 1; // 5 00586 uint8_t tx_pkt_type : 2; // 6,7 defines packet type field in header 00587 } bits; 00588 struct { 00589 uint8_t infinite_rxtx : 1; // 0 00590 uint8_t tx_payload_src : 1; // 1 0=fifo 1=prbs 00591 uint8_t tx_type : 3; // 2,3,4 payload for BLE tx test 00592 uint8_t cs_type : 3; // 5,6,7 connection state 00593 } ble; 00594 uint8_t octet; 00595 } PktTxHeader_t; // 0x9c2 00596 00597 typedef union { 00598 struct { 00599 uint8_t crc_in_fifo : 1; // 0 1=received CRC written to FIFO at end 00600 uint8_t flora_coding_rate : 2; // 1,2 00601 uint8_t whit_disable : 1; // 3 00602 uint8_t crc_mode : 2; // 4,5 0=nocrc 1=1byteCRC 2=2byteCRC 3=reserved 00603 uint8_t rssi_mode : 2; // 6,7 enables rssi read mode of received packets 0=none 1=syncReg 2=avgReg 3=bothRegs 00604 } bits; 00605 uint8_t octet; 00606 } PktBitStreamCtrl_t; // 0x9c4 00607 00608 typedef union { 00609 struct { 00610 uint8_t flora_preamble_20_16 : 5; // 0,1,2,3,4 00611 uint8_t data_rate : 3; // 5,6,7 00612 } bits; 00613 uint8_t octet; 00614 } FloraPreambleHi_t; // 0x9ca 00615 00616 typedef union { 00617 struct { 00618 uint8_t sync_adrs_bit_detections : 2; // 0,1 last bits matching 0=none 1=2bits 2=4bits 3=8bits 00619 uint8_t reserved : 2; // 2,3 00620 uint8_t sync_addr_mask : 3; // 4,5,6 00621 uint8_t cont_rx : 1; // 7 00622 } ble; 00623 struct { 00624 uint8_t sync_adrs_errors : 4; // 0,12,3 how many bit errors are tolerated in sync word 00625 uint8_t sync_addr_mask : 3; // 4,5,6 00626 uint8_t cont_rx : 1; // 7 00627 } gfskflrc; 00628 uint8_t octet; 00629 } PktSyncAdrs_t; // 0x9cd 00630 00631 typedef union { 00632 struct { 00633 uint8_t tolerated_errors : 4; // 0,1,2,3 for sync word 00634 uint8_t valid_status : 1; // 4 0x98c-98f is valid 00635 uint8_t reserved : 3; // 5,6,7 00636 } bits; 00637 uint8_t octet; 00638 } FlrcSyncWordCtrl_t; // 0x98b 00639 00640 typedef union { 00641 struct { 00642 uint8_t sync_word_irq : 1; // 0 00643 uint8_t preamble_irq : 1; // 1 00644 uint8_t stop_radio : 1; // 3 00645 uint8_t reserved : 1; // 4,5,6,7 00646 } bits; 00647 uint8_t octet; 00648 } FlrcIrqStatus_t; // 0x990 00649 00650 typedef union { 00651 struct { 00652 uint8_t tx_pwr : 5; // 0,1,2,3,4 00653 uint8_t ramp_time : 3; // 5,6,7 00654 } bits; 00655 uint8_t octet; 00656 } PaPwrCtrl_t; // 0xa53 00657 00658 class SX128x { 00659 public: 00660 SX128x(SPI&, PinName nss, PinName busy, PinName diox, PinName nrst); 00661 00662 static Callback<void()> diox_topHalf; // low latency ISR context 00663 Callback<void()> txDone; // user context 00664 void (*rxDone)(uint8_t size, const pktStatus_t*); // user context 00665 Callback<void()> chipModeChange; // read chipMode_e chipMode 00666 void (*timeout)(bool tx); // user context 00667 void (*cadDone)(bool); 00668 00669 //! RF transmit packet buffer 00670 uint8_t tx_buf[256]; // lora fifo size 00671 00672 //! RF receive packet buffer 00673 uint8_t rx_buf[256]; // lora fifo size 00674 00675 void service(void); 00676 uint8_t xfer(uint8_t opcode, uint8_t writeLen, uint8_t readLen, uint8_t* buf); 00677 void start_tx(uint8_t pktLen, float timeout_ms); // tx_buf must be filled prior to calling 00678 void start_rx(float timeout_ms); // timeout given in milliseconds, -1 = forever 00679 void hw_reset(void); 00680 void set_tx_dbm(int8_t dbm); 00681 void setMHz(float); 00682 float getMHz(void); 00683 void setStandby(stby_t); 00684 void setSleep(bool warm); 00685 void setFS(void); 00686 void setCAD(void); 00687 void writeReg(uint16_t addr, uint32_t data, uint8_t len); 00688 uint32_t readReg(uint16_t addr, uint8_t len); 00689 void setPacketType(uint8_t); 00690 uint8_t getPacketType(void); 00691 void ReadBuffer(uint8_t size, uint8_t offset); 00692 uint64_t getSyncAddr(uint8_t num); 00693 void setSyncAddr(uint8_t num, uint64_t sa); 00694 void setRegulator(uint8_t); 00695 void setBufferBase(uint8_t txAddr, uint8_t rxAddr); 00696 00697 chipMode_e chipMode; 00698 uint8_t periodBase; 00699 static const float timeOutStep[4]; 00700 uint8_t pktType; 00701 00702 private: 00703 SPI& spi; 00704 DigitalOut nss; 00705 DigitalIn busy; 00706 InterruptIn diox; 00707 DigitalInOut nrst; 00708 static void dioxisr(void); 00709 bool sleeping; 00710 }; 00711 00712 #endif /* SX126x_H */ 00713
Generated on Sun Jul 17 2022 01:33:56 by
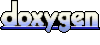