LoRaWAN end device MAC layer for SX1272 and SX1276. Supports LoRaWAN-1.0 and LoRaWAN-1.1
Dependents: LoRaWAN-SanJose_Bootcamp LoRaWAN-grove-cayenne LoRaWAN-classC-demo LoRaWAN-grove-cayenne ... more
utilities.cpp
00001 #include "lorawan_board.h" 00002 00003 00004 // Assumes 0 <= max <= RAND_MAX 00005 // Returns in the closed interval [0, max] 00006 long random_at_most(long max) { 00007 unsigned long 00008 // max <= RAND_MAX < ULONG_MAX, so this is okay. 00009 num_bins = (unsigned long) max + 1, 00010 num_rand = (unsigned long) RAND_MAX + 1, 00011 bin_size = num_rand / num_bins, 00012 defect = num_rand % num_bins; 00013 00014 long x; 00015 do { 00016 //x = random(); 00017 x = rand(); 00018 } 00019 // This is carefully written not to overflow 00020 while (num_rand - defect <= (unsigned long)x); 00021 00022 // Truncated division is intentional 00023 return x/bin_size; 00024 } 00025 00026 void memcpyr( uint8_t *dst, const uint8_t *src, uint16_t size ) 00027 { 00028 dst = dst + ( size - 1 ); 00029 while( size-- ) 00030 { 00031 *dst-- = *src++; 00032 } 00033 } 00034 00035 void 00036 #ifdef ENABLE_VT100 00037 print_buf(const uint8_t* const buf, uint8_t len, const char* txt, uint8_t row) 00038 { 00039 uint8_t i; 00040 vt.SetCursorPos(row, 1 ); 00041 vt.printf("%s: ", txt); 00042 for (i = 0; i < len; i++) 00043 vt.printf("%02x ", buf[i]); 00044 vt.printf("\e[K"); 00045 #else 00046 print_buf(const uint8_t* const buf, uint8_t len, const char* txt) 00047 { 00048 uint8_t i; 00049 pc.printf("%s: ", txt); 00050 for (i = 0; i < len; i++) 00051 pc.printf("%02x ", buf[i]); 00052 pc.printf("\r\n"); 00053 #endif /* ENABLE_VT100 */ 00054 } 00055 00056 bool ValueInRange( int8_t value, int8_t min, int8_t max ) 00057 { 00058 if( ( value >= min ) && ( value <= max ) ) 00059 { 00060 return true; 00061 } 00062 return false; 00063 } 00064
Generated on Mon Jul 18 2022 19:16:49 by
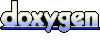