LoRaWAN end device MAC layer for SX1272 and SX1276. Supports LoRaWAN-1.0 and LoRaWAN-1.1
Dependents: LoRaWAN-SanJose_Bootcamp LoRaWAN-grove-cayenne LoRaWAN-classC-demo LoRaWAN-grove-cayenne ... more
LoRaMacCrypto.h
00001 #include <stdint.h> 00002 00003 #define LORA_EUI_LENGTH 8 00004 #define UP_LINK 0 00005 #define DOWN_LINK 1 00006 00007 typedef struct { 00008 uint8_t AppSKey[16]; 00009 uint8_t FNwkSIntKey[16]; 00010 uint8_t SNwkSIntKey[16]; 00011 uint8_t NwkSEncKey[16]; 00012 } skey_t; 00013 00014 typedef union { 00015 struct { 00016 uint8_t header; 00017 uint16_t confFCnt; 00018 uint8_t dr; 00019 uint8_t ch; 00020 uint8_t dir; 00021 uint32_t DevAddr; 00022 uint32_t FCnt; 00023 uint8_t zero8; 00024 uint8_t lenMsg; 00025 } __attribute__((packed)) b; 00026 uint8_t octets[16]; 00027 } block_t; 00028 00029 void LoRaMacEncrypt( uint8_t ctr, const uint8_t *buffer, uint16_t size, const uint8_t *key, uint32_t address, uint8_t dir, uint32_t sequenceCounter, uint8_t *encBuffer ); 00030 //void LoRaMacComputeMic( bool OptNeg, const uint8_t *buffer, uint16_t size, skey_t* kptr, uint32_t address, uint8_t dir, uint32_t sequenceCounter, uint16_t ConfFCnt, uint8_t dr, uint8_t ch, uint32_t *mic ); 00031 void LoRaMacPayloadDecrypt( const uint8_t *buffer, uint16_t size, const uint8_t *key, uint32_t address, uint8_t dir, uint32_t sequenceCounter, uint8_t *decBuffer ); 00032 uint32_t LoRaMacComputeMic( const block_t* block, const uint8_t* pktPayload, const uint8_t* key); 00033 #ifdef LORAWAN_JOIN_EUI 00034 void LoRaMacGenerateJoinKey(uint8_t token, const uint8_t* root_key, const uint8_t* devEui, uint8_t* output); 00035 int LoRaMacJoinComputeMic(bool verbose, const uint8_t *buffer, uint16_t size, const uint8_t *key, uint32_t *mic ); 00036 void LoRaMacJoinDecrypt( const uint8_t *buffer, uint16_t size, const uint8_t *key, uint8_t *decBuffer ); 00037 void LoRaMacJoinComputeSKeys_1v0( const uint8_t *root_key, const uint8_t *ja_rx, uint16_t devNonce, skey_t* keys); 00038 void LoRaMacJoinComputeSKeys_1v1( const uint8_t *nwk_root_key, const uint8_t *app_root_key, const uint8_t *joinNonce, const uint8_t *joinEUI, uint16_t devNonce, skey_t* keys); 00039 #endif /* LORAWAN_JOIN_EUI */ 00040 void LoRaMacCryptoInit(void);
Generated on Mon Jul 18 2022 19:16:49 by
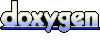