LoRaWAN end device MAC layer for SX1272 and SX1276. Supports LoRaWAN-1.0 and LoRaWAN-1.1
Dependents: LoRaWAN-SanJose_Bootcamp LoRaWAN-grove-cayenne LoRaWAN-classC-demo LoRaWAN-grove-cayenne ... more
LoRaMac1v1.h
00001 #ifndef _LORAMAC_H_ 00002 #define _LORAMAC_H_ 00003 00004 #include "Commissioning.h" 00005 00006 typedef enum { 00007 /* 0 */ LORAMAC_STATUS_OK = 0, 00008 /* 1 */ LORAMAC_STATUS_LSE, 00009 /* 2 */ LORAMAC_STATUS_WAITING_FOR_TXSTART, 00010 /* 3 */ LORAMAC_STATUS_WAITING_FOR_TXDONE, 00011 /* 4 */ LORAMAC_STATUS_WAITING_FOR_RX1, 00012 /* 5 */ LORAMAC_STATUS_WAITING_FOR_RX2, 00013 /* 6 */ LORAMAC_STATUS_BUSY_UPCONF, 00014 /* 7 */ LORAMAC_STATUS_SERVICE_UNKNOWN, 00015 /* 8 */ LORAMAC_STATUS_PARAMETER_INVALID, 00016 /* 9 */ LORAMAC_STATUS_DATARATE_INVALID, 00017 /* 10 */ LORAMAC_STATUS_FREQUENCY_INVALID, 00018 /* 11 */ LORAMAC_STATUS_LENGTH_ERROR, 00019 /* 12 */ LORAMAC_STATUS_DEVICE_OFF, 00020 /* 13 */ LORAMAC_STATUS_FREQ_AND_DR_INVALID, 00021 /* 14 */ LORAMAC_STATUS_EEPROM_FAIL, 00022 /* 15 */ LORAMAC_STATUS_MAC_CMD_LENGTH_ERROR, 00023 #ifdef LORAWAN_JOIN_EUI 00024 /* 16 */ LORAMAC_STATUS_NO_NETWORK_JOINED 00025 #endif 00026 } LoRaMacStatus_t; 00027 00028 typedef enum { 00029 LORAMAC_EVENT_INFO_STATUS_OK, 00030 LORAMAC_EVENT_INFO_STATUS_INCR_FAIL, 00031 LORAMAC_EVENT_INFO_STATUS_MLMEREQ, 00032 LORAMAC_EVENT_INFO_STATUS_UNKNOWN_MTYPE, 00033 LORAMAC_EVENT_INFO_STATUS_SENDING, 00034 LORAMAC_EVENT_INFO_STATUS_MCPSREQ, 00035 LORAMAC_EVENT_INFO_STATUS_TX_TIMEOUT, 00036 LORAMAC_EVENT_INFO_STATUS_RX2_TIMEOUT, 00037 LORAMAC_EVENT_INFO_STATUS_RX2_ERROR, 00038 LORAMAC_EVENT_INFO_STATUS_DOWNLINK_REPEATED, 00039 LORAMAC_EVENT_INFO_STATUS_TX_DR_PAYLOAD_SIZE_ERROR, 00040 LORAMAC_EVENT_INFO_STATUS_DOWNLINK_TOO_MANY_FRAMES_LOSS, 00041 LORAMAC_EVENT_INFO_STATUS_ADDRESS_FAIL, 00042 LORAMAC_EVENT_INFO_STATUS_MIC_FAIL, 00043 LORAMAC_EVENT_INFO_STATUS_MULTICAST_FAIL, 00044 LORAMAC_EVENT_INFO_STATUS_BEACON_LOCKED, 00045 LORAMAC_EVENT_INFO_STATUS_BEACON_LOST, 00046 LORAMAC_EVENT_INFO_STATUS_BEACON_NOT_FOUND, 00047 LORAMAC_EVENT_INFO_STATUS_NO_APPKEY, 00048 LORAMAC_EVENT_INFO_BAD_RX_DELAY, 00049 LORAMAC_EVENT_INFO_STATUS_CHANNEL_BUSY, 00050 #ifdef LORAWAN_JOIN_EUI 00051 LORAMAC_EVENT_INFO_STATUS_JOIN_FAIL, 00052 LORAMAC_EVENT_INFO_STATUS_JOINNONCE 00053 #endif 00054 } LoRaMacEventInfoStatus_t; 00055 00056 typedef enum { 00057 MCPS_NONE, 00058 MCPS_UNCONFIRMED, 00059 MCPS_CONFIRMED, 00060 MCPS_MULTICAST, 00061 MCPS_PROPRIETARY 00062 } Mcps_t; 00063 00064 #include <stdint.h> 00065 typedef struct { 00066 LoRaMacEventInfoStatus_t Status; 00067 Mcps_t McpsRequest; 00068 uint8_t NbRetries; 00069 bool AckReceived; 00070 uint32_t UpLinkCounter; 00071 uint8_t Datarate; 00072 int8_t TxPower; 00073 uint32_t UpLinkFreqHz; 00074 //us_timestamp_t TxTimeOnAir; 00075 } McpsConfirm_t; 00076 00077 typedef struct { 00078 uint8_t Snr; 00079 int16_t Rssi; 00080 int8_t RxSlot; 00081 LoRaMacEventInfoStatus_t Status; 00082 Mcps_t McpsIndication; 00083 bool RxData; 00084 uint8_t RxDatarate; 00085 uint8_t Port; 00086 uint8_t BufferSize; 00087 uint8_t *Buffer; 00088 uint8_t FramePending; 00089 bool AckReceived; 00090 uint32_t expectedFCntDown; 00091 uint16_t receivedFCntDown; 00092 uint16_t ADR_ACK_CNT; 00093 } McpsIndication_t; 00094 00095 typedef enum { 00096 MLME_NONE = 0, 00097 MLME_LINK_CHECK, 00098 MLME_SWITCH_CLASS, 00099 MLME_PING_SLOT_INFO, 00100 MLME_BEACON_TIMING, 00101 MLME_BEACON_ACQUISITION, 00102 MLME_TIME_REQ, 00103 MLME_BEACON, 00104 MLME_TXCW, 00105 #ifdef LORAWAN_JOIN_EUI 00106 MLME_JOIN, 00107 MLME_REJOIN_0, 00108 MLME_REJOIN_1, 00109 MLME_REJOIN_2 00110 #endif 00111 } Mlme_t; 00112 00113 #include "lorawan_board.h" 00114 00115 #define DR_0 0 00116 #define DR_1 1 00117 #define DR_2 2 00118 #define DR_3 3 00119 #define DR_4 4 00120 #define DR_5 5 00121 #define DR_6 6 00122 #define DR_7 7 00123 #define DR_8 8 00124 #define DR_9 9 00125 #define DR_10 10 00126 #define DR_11 11 00127 #define DR_12 12 00128 #define DR_13 13 00129 #define DR_14 14 00130 #define DR_15 15 00131 00132 00133 typedef union { 00134 uint8_t Value; 00135 00136 struct { 00137 int8_t Min : 4; 00138 int8_t Max : 4; 00139 } Fields; 00140 } DrRange_t; 00141 00142 typedef struct { 00143 uint32_t FreqHz; 00144 DrRange_t DrRange; 00145 uint8_t Band; 00146 } ChannelParams_t; 00147 00148 00149 typedef struct { 00150 Mcps_t Type; 00151 struct { 00152 void *fBuffer; 00153 uint16_t fBufferSize; 00154 uint8_t Datarate; 00155 uint8_t fPort; 00156 } Req; 00157 } McpsReq_t; 00158 00159 typedef struct { 00160 uint8_t MaxPossiblePayload; 00161 uint8_t CurrentPayloadSize; 00162 } LoRaMacTxInfo_t; 00163 00164 typedef enum { 00165 MIB_DEV_ADDR, 00166 MIB_DEVICE_CLASS, 00167 MIB_ADR, 00168 MIB_PUBLIC_NETWORK, 00169 MIB_RX2_CHANNEL, 00170 MIB_CHANNELS_MASK, 00171 MIB_FNwkSIntKey, 00172 MIB_APP_SKEY, 00173 MIB_SNwkSIntKey, 00174 MIB_NwkSEncKey, 00175 MIB_NwkSKey, /* lorawan 1.0 */ 00176 MIB_MAX_LISTEN_TIME, 00177 #ifdef LORAWAN_JOIN_EUI 00178 MIB_NETWORK_JOINED 00179 #endif 00180 } Mib_t; 00181 00182 typedef enum { 00183 CLASS_A = 0, 00184 CLASS_B, 00185 CLASS_C 00186 } DeviceClass_t; 00187 00188 typedef struct { 00189 uint32_t FrequencyHz; 00190 uint8_t Datarate; 00191 } Rx2ChannelParams_t; 00192 00193 typedef union { 00194 uint32_t DevAddr; 00195 bool AdrEnable; 00196 DeviceClass_t Class; 00197 bool IsNetworkJoined; 00198 bool EnablePublicNetwork; 00199 uint16_t* ChannelsMask; 00200 const uint8_t* key; 00201 Rx2ChannelParams_t Rx2Channel; 00202 us_timestamp_t MaxListenTime; 00203 } MibParam_t; 00204 00205 typedef struct { 00206 Mib_t Type; 00207 MibParam_t Param; 00208 } MibRequestConfirm_t; 00209 00210 00211 00212 typedef struct { 00213 Mlme_t Type; 00214 00215 union { 00216 struct { 00217 DeviceClass_t Class; 00218 } SwitchClass; 00219 00220 union { 00221 uint8_t Value; 00222 struct sInfoFields { 00223 uint8_t Periodicity : 3; 00224 uint8_t RFU : 5; 00225 } Fields; 00226 } PingSlotInfo; 00227 00228 #ifdef LORAWAN_JOIN_EUI 00229 struct { 00230 const uint8_t *DevEui; 00231 const uint8_t *JoinEui; 00232 const uint8_t *NwkKey; 00233 const uint8_t *AppKey; 00234 uint8_t NbTrials; 00235 } Join; 00236 #endif /* LORAWAN_JOIN_EUI */ 00237 00238 struct { 00239 uint16_t Timeout; 00240 } TxCw; 00241 } Req; 00242 } MlmeReq_t; 00243 00244 00245 typedef struct { 00246 LoRaMacEventInfoStatus_t Status; 00247 Mlme_t MlmeRequest; 00248 union { 00249 struct { 00250 uint8_t DemodMargin; 00251 uint8_t NbGateways; 00252 } link; 00253 struct { 00254 uint32_t Seconds; // seconds since epoch 00255 uint32_t uSeconds; // fractional part 00256 } time; 00257 struct { 00258 uint32_t rxJoinNonce; 00259 uint32_t myJoinNonce; 00260 } join; 00261 } fields; 00262 //us_timestamp_t TxTimeOnAir; 00263 } MlmeConfirm_t; 00264 00265 00266 typedef struct { 00267 Mlme_t MlmeIndication; 00268 LoRaMacEventInfoStatus_t Status; 00269 uint32_t freqHz; 00270 #ifdef LORAWAN_JOIN_EUI 00271 uint8_t JoinRequestTrials; 00272 #endif /* LORAWAN_JOIN_EUI */ 00273 } MlmeIndication_t; 00274 00275 00276 /*! 00277 * LoRaMAC events structure 00278 * Used to notify upper layers of MAC events 00279 */ 00280 typedef struct sLoRaMacPrimitives 00281 { 00282 /*! 00283 * \brief MCPS-Confirm primitive 00284 * 00285 * \param [OUT] MCPS-Confirm parameters 00286 */ 00287 void (* const MacMcpsConfirm )( const McpsConfirm_t *McpsConfirm ); 00288 /*! 00289 * \brief MCPS-Indication primitive 00290 * 00291 * \param [OUT] MCPS-Indication parameters 00292 */ 00293 void (* const MacMcpsIndication )( const McpsIndication_t *McpsIndication ); 00294 /*! 00295 * \brief MLME-Confirm primitive 00296 * 00297 * \param [OUT] MLME-Confirm parameters 00298 */ 00299 void (* const MacMlmeConfirm )( const MlmeConfirm_t *MlmeConfirm ); 00300 /*! 00301 * \brief MLME-Indication primitive 00302 * 00303 * \param [OUT] MLME-Indication parameters 00304 */ 00305 void (* const MacMlmeIndication )( const MlmeIndication_t *MlmeIndication ); 00306 } LoRaMacPrimitives_t ; 00307 00308 typedef struct sLoRaMacCallback 00309 { 00310 /*! 00311 * \brief Measures the battery level 00312 * 00313 * \retval Battery level [0: node is connected to an external 00314 * power source, 1..254: battery level, where 1 is the minimum 00315 * and 254 is the maximum value, 255: the node was not able 00316 * to measure the battery level] 00317 */ 00318 uint8_t (* const GetBatteryLevel )( void ); 00319 /*! 00320 * \brief Measures the temperature level 00321 * 00322 * \retval Temperature level 00323 */ 00324 float (* const GetTemperatureLevel )( void ); 00325 } LoRaMacCallback_t; 00326 00327 LoRaMacStatus_t LoRaMacInitialization( const LoRaMacPrimitives_t *primitives, const LoRaMacCallback_t *callbacks ); 00328 us_timestamp_t LoRaMacReadTimer(void); 00329 LoRaMacStatus_t LoRaMacQueryTxPossible(uint8_t size, LoRaMacTxInfo_t* txInfo); 00330 LoRaMacStatus_t LoRaMacMcpsRequest( McpsReq_t *mcpsRequest ); 00331 LoRaMacStatus_t LoRaMacMibGetRequestConfirm( MibRequestConfirm_t *mibGet ); 00332 LoRaMacStatus_t LoRaMacMlmeRequest( const MlmeReq_t *mlmeRequest ); 00333 LoRaMacStatus_t LoRaMacMibSetRequestConfirm( MibRequestConfirm_t *mibSet ); 00334 LoRaMacStatus_t LoRaMacChannelAdd( uint8_t id, ChannelParams_t params ); 00335 void LoRaMacPrintStatus(void); 00336 uint32_t get_fcntdwn(bool); 00337 int8_t LoRaMacGetRxSlot(void); 00338 void LoRaMacUserContext(void); 00339 00340 #endif /* _LORAMAC_H_ */
Generated on Mon Jul 18 2022 19:16:49 by
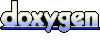