
star-mesh LoRa network
Embed:
(wiki syntax)
Show/hide line numbers
app_sx126x.cpp
00001 #include "sx12xx.h" 00002 #ifdef SX126x_H 00003 #include "main.h" 00004 00005 uint8_t tx_param_buf[2]; 00006 uint8_t pa_config_buf[4]; 00007 00008 void tx_dbm_print() 00009 { 00010 PwrCtrl_t PwrCtrl; 00011 PaCtrl1b_t PaCtrl1b; 00012 unsigned v = Radio::radio.readReg(REG_ADDR_ANACTRL16, 1); 00013 00014 if (v & 0x10) { 00015 pc.printf("%d", PA_OFF_DBM); 00016 return; 00017 } 00018 00019 PwrCtrl.octet = Radio::radio.readReg(REG_ADDR_PWR_CTRL, 1); 00020 00021 PaCtrl1b.octet = Radio::radio.readReg(REG_ADDR_PA_CTRL1B, 1); 00022 pa_config_buf[2] = PaCtrl1b.bits.tx_mode_bat; // deviceSel 00023 00024 if (PaCtrl1b.bits.tx_mode_bat) 00025 pc.printf("%ddBm ", PwrCtrl.bits.tx_pwr - 17); 00026 else 00027 pc.printf("%ddBm ", PwrCtrl.bits.tx_pwr - 9); 00028 } 00029 00030 bool isRadioRxing() 00031 { 00032 status_t status; 00033 Radio::radio.xfer(OPCODE_GET_STATUS, 0, 1, &status.octet); 00034 return status.bits.chipMode == 5; 00035 } 00036 00037 void radio_printOpMode() 00038 { 00039 status_t status; 00040 Radio::radio.xfer(OPCODE_GET_STATUS, 0, 1, &status.octet); 00041 00042 /* translate opmode_status_strs to opmode_select_strs */ 00043 switch (status.bits.chipMode) { 00044 case 2: pc.printf("STBY_RC"); break; 00045 case 3: pc.printf("STBY_XOSC"); break; 00046 case 4: pc.printf("FS"); break; 00047 case 5: pc.printf("RX"); break; 00048 case 6: pc.printf("TX"); break; 00049 default: pc.printf("<%d>", status.bits.chipMode); break; 00050 } 00051 } 00052 00053 void lora_printHeaderMode() 00054 { 00055 loraConfig1_t conf1; 00056 conf1.octet = Radio::radio.readReg(REG_ADDR_LORA_CONFIG1, 1); 00057 if (conf1.bits.implicit_header) 00058 pc.printf("implicit "); 00059 else 00060 pc.printf("explicit "); 00061 } 00062 00063 void radio_print_status() 00064 { 00065 tx_dbm_print(); 00066 pc.printf(" %.3fMHz ", Radio::radio.getMHz()); 00067 radio_printOpMode(); 00068 pc.printf("\r\n"); 00069 } 00070 00071 bool tx_dbm_write(int dbm) 00072 { 00073 unsigned v = Radio::radio.readReg(REG_ADDR_ANACTRL16, 1); 00074 00075 if (dbm == PA_OFF_DBM) { 00076 /* bench test: prevent overloading receiving station (very low tx power) */ 00077 v |= 0x10; // pa dac atb tst 00078 Radio::radio.writeReg(REG_ADDR_ANACTRL16, v, 1); 00079 } else { 00080 tx_param_buf[0] = dbm; 00081 Radio::radio.xfer(OPCODE_SET_TX_PARAMS, 2, 0, tx_param_buf); 00082 00083 if (v & 0x10) { 00084 v &= ~0x10; 00085 Radio::radio.writeReg(REG_ADDR_ANACTRL16, v, 1); 00086 } 00087 } 00088 00089 return false; 00090 } 00091 00092 void cmd_op(uint8_t argsAt) 00093 { 00094 int dbm; 00095 if (sscanf(pcbuf+argsAt, "%d", &dbm) == 1) { 00096 tx_dbm_write(dbm); 00097 } 00098 00099 tx_dbm_print(); 00100 } 00101 00102 #endif /* ..SX126x_H */ 00103
Generated on Fri Jul 15 2022 20:28:20 by
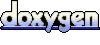