
star-mesh LoRa network
Embed:
(wiki syntax)
Show/hide line numbers
app_gateway.cpp
00001 00002 #include "main.h" 00003 #ifdef GATEWAY 00004 #include "app.h" 00005 00006 00007 void cmd_downlink(uint8_t argsAt) 00008 { 00009 uint32_t requested_id; 00010 const char* strPtr; 00011 uint8_t n, lenIdx; 00012 00013 /* if (flags.CallTXRequest) { 00014 pc.printf("busy\r\n"); 00015 return; 00016 }*/ 00017 00018 if (sscanf(pcbuf+argsAt, "%lx", &requested_id) != 1) { 00019 pc.printf("provide destination ID\r\n"); 00020 return; 00021 } 00022 00023 tx_dest_id = find_dest_id(requested_id); 00024 if (tx_dest_id == ID_NONE) { 00025 pc.printf("id %lx not found\r\n", requested_id); 00026 return; 00027 } 00028 pc.printf("downlink to %lx ", tx_dest_id); 00029 00030 reqFlags.bits.currentOp = CMD_USER_PAYLOAD_DN_REQ; 00031 txBuf[txBuf_idx++] = CMD_USER_PAYLOAD_DN_REQ; 00032 putu32ToBuf(&txBuf[txBuf_idx], requested_id); 00033 txBuf_idx += 4; 00034 lenIdx = txBuf_idx++; 00035 00036 strPtr = pcbuf + argsAt; 00037 strPtr = strchr(++strPtr, ' '); 00038 n = 0; 00039 while (strPtr) { 00040 unsigned octet; 00041 sscanf(strPtr, "%x", &octet); 00042 txBuf[txBuf_idx++] = octet; 00043 strPtr = strchr(++strPtr, ' '); 00044 n++; 00045 } 00046 txBuf[lenIdx] = n; 00047 00048 flags.CallTXRequest = 1; // transmit will occur in idle-rxsingle cycle 00049 00050 pc.printf("\r\n"); 00051 } 00052 00053 void gateway_uplink(uint8_t len, uint32_t orig_src, const uint8_t* payload) 00054 { 00055 unsigned n; 00056 pc.printf("userPayload %u from src:%lx: ", len, orig_src); 00057 /* take here for parsing*/ 00058 for (n = 0; n < len; n++) { 00059 pc.printf("%02x ", payload[n]); 00060 } 00061 pc.printf("\r\n"); 00062 } 00063 00064 void app_init() 00065 { 00066 } 00067 #endif /* GATEWAY */
Generated on Fri Jul 15 2022 20:28:20 by
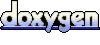