
star-mesh LoRa network
Embed:
(wiki syntax)
Show/hide line numbers
app_endDevice.cpp
00001 #include "main.h" 00002 #ifndef GATEWAY 00003 #include "app.h" 00004 00005 InterruptIn but(USER_BUTTON); 00006 00007 /* downlink handler */ 00008 void app_downlink(uint8_t len, const uint8_t* payload) 00009 { 00010 unsigned n; 00011 pc.printf("downlink %u: ", len); 00012 for (n = 0; n < len; n++) 00013 pc.printf("%02x ", payload[n]); 00014 00015 pc.printf("\r\n"); 00016 } 00017 00018 void button_check() 00019 { 00020 static uint8_t cnt = 0; 00021 00022 if (but.read() == 0) { 00023 uint8_t buf[2]; 00024 buf[0] = BUTTON_PRESS; 00025 buf[1] = cnt++; 00026 pc.printf("appUp "); 00027 if (uplink(buf, 2)) 00028 pc.printf("upFail\r\n"); 00029 } else { 00030 pc.printf("appBounce\r\n"); 00031 but.enable_irq(); 00032 } 00033 } 00034 00035 void button_pressed() 00036 { 00037 queue.call_in(10, button_check); 00038 but.disable_irq(); 00039 } 00040 00041 void app_uplink_complete() 00042 { 00043 but.enable_irq(); 00044 } 00045 00046 void app_init() 00047 { 00048 /* nucleo: user button active low, inactive hi */ 00049 but.fall(button_pressed); 00050 } 00051 #endif /* !GATEWAY */
Generated on Fri Jul 15 2022 20:28:20 by
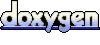