MPL3115A2 driver
Dependents: lmic_NAmote_GPS_tjm lmic_NAmote_GPS_tjm Senet NAMote scpi_sx127x ... more
mpl3115a2.cpp
00001 #include "mpl3115a2.h" 00002 00003 #define MPL3115A_I2C_ADDRESS 0xc0 //0x60 00004 00005 MPL3115A2::MPL3115A2(I2C& r, DigitalIn& int_pin) : m_i2c(r), m_int_pin(int_pin) 00006 { 00007 write(CTRL_REG3, 0x10); // PP_OD1: INT1 to open-drain 00008 } 00009 00010 MPL3115A2::~MPL3115A2() 00011 { 00012 } 00013 00014 void MPL3115A2::init() 00015 { 00016 //MPL3115Reset( ); 00017 ctrl_reg1.octet = 0; 00018 ctrl_reg1.bits.RST = 1; 00019 write(CTRL_REG1, /*4*/ ctrl_reg1.octet); 00020 wait(0.05); 00021 00022 do 00023 { // Wait for the RST bit to clear 00024 wait(0.01); 00025 ctrl_reg1.octet = read(CTRL_REG1); 00026 } while (ctrl_reg1.octet); 00027 00028 write( PT_DATA_CFG_REG, 0x07 ); // Enable data flags 00029 write( CTRL_REG3, 0x11 ); // Open drain, active low interrupts 00030 write( CTRL_REG4, 0x80 ); // Enable DRDY interrupt 00031 write( CTRL_REG5, 0x00 ); // DRDY interrupt routed to INT2 - PTD3 00032 00033 ctrl_reg1.bits.ALT = 1; // altitude mode 00034 ctrl_reg1.bits.OS = 5; // OSR = 32 00035 ctrl_reg1.bits.SBYB = 1; // Active 00036 write(CTRL_REG1, ctrl_reg1.octet); 00037 00038 SetModeActive( ); 00039 } 00040 00041 void MPL3115A2::setOSR(uint8_t osr) 00042 { 00043 ctrl_reg1.bits.OS = osr; 00044 write(CTRL_REG1, ctrl_reg1.octet); 00045 } 00046 00047 uint8_t MPL3115A2::getOSR(void) 00048 { 00049 ctrl_reg1.octet = read(CTRL_REG1); 00050 return ctrl_reg1.bits.OS; 00051 } 00052 00053 bool MPL3115A2::GetModeActive( ) 00054 { 00055 ctrl_reg1.octet = read(CTRL_REG1); 00056 return ctrl_reg1.bits.SBYB; 00057 } 00058 00059 void MPL3115A2::SetModeActive( ) 00060 { 00061 ctrl_reg1.bits.SBYB = 1; 00062 write(CTRL_REG1, ctrl_reg1.octet); 00063 } 00064 00065 void MPL3115A2::SetModeStandby( ) 00066 { 00067 ctrl_reg1.bits.SBYB = 0; 00068 write(CTRL_REG1, ctrl_reg1.octet); 00069 } 00070 00071 void MPL3115A2::write(uint8_t a, uint8_t d) 00072 { 00073 char cmd[2]; 00074 00075 cmd[0] = a; 00076 cmd[1] = d; 00077 00078 if (m_i2c.write(MPL3115A_I2C_ADDRESS, cmd, 2)) 00079 printf("MPL write-fail %02x %02x\n", cmd[0], cmd[1]); 00080 00081 if (a == CTRL_REG4) 00082 ctrl_reg4 = d; 00083 } 00084 00085 uint8_t MPL3115A2::read(uint8_t a) 00086 { 00087 char cmd[2]; 00088 00089 cmd[0] = a; 00090 if (m_i2c.write(MPL3115A_I2C_ADDRESS, cmd, 1, true)) 00091 printf("MPL write-fail %02x\n", cmd[0]); 00092 if (m_i2c.read(MPL3115A_I2C_ADDRESS, cmd, 1)) 00093 printf("MPL read-fail\n"); 00094 00095 if (a == CTRL_REG4) 00096 ctrl_reg4 = cmd[0]; 00097 00098 return cmd[0]; 00099 } 00100 00101 float MPL3115A2::ReadBarometer(void) 00102 { 00103 uint32_t pasc; 00104 volatile uint8_t stat; 00105 00106 SetModeBarometer(); 00107 ToggleOneShot( ); 00108 00109 stat = read(STATUS_REG); 00110 while( (stat & 0x04) != 0x04 ) { 00111 wait(0.01); 00112 stat = read(STATUS_REG); 00113 } 00114 00115 pasc = read(OUT_P_MSB_REG); 00116 pasc <<= 8; 00117 pasc |= read(OUT_P_CSB_REG); 00118 pasc <<= 8; 00119 pasc |= read(OUT_P_LSB_REG); 00120 00121 return pasc / 64.0; 00122 } 00123 00124 float MPL3115A2::ReadAltitude( void ) 00125 { 00126 uint8_t counter = 0; 00127 uint8_t val = 0; 00128 uint8_t msb = 0, csb = 0, lsb = 0; 00129 float decimal = 0; 00130 00131 /*if( MPL3115Initialized == false ) 00132 { 00133 return 0; 00134 }*/ 00135 00136 SetModeAltimeter( ); 00137 ToggleOneShot( ); 00138 00139 while( ( val & 0x04 ) != 0x04 ) 00140 { 00141 val = read( STATUS_REG); 00142 wait(0.01); //DelayMs( 10 ); 00143 counter++; 00144 00145 if( counter > 20 ) 00146 { 00147 //MPL3115Initialized = false; 00148 init( ); 00149 SetModeAltimeter( ); 00150 ToggleOneShot( ); 00151 counter = 0; 00152 while( ( val & 0x04 ) != 0x04 ) 00153 { 00154 val = read( STATUS_REG); 00155 wait(0.01); //DelayMs( 10 ); 00156 counter++; 00157 if( counter > 20 ) 00158 { 00159 write( CTRL_REG4, 0x00 ); 00160 return( 0 ); //Error out after max of 512ms for a read 00161 } 00162 } 00163 } 00164 } 00165 00166 msb = read( OUT_P_MSB_REG); // High byte of integer part of altitude, 00167 csb = read( OUT_P_CSB_REG); // Low byte of integer part of altitude 00168 lsb = read( OUT_P_LSB_REG); // Decimal part of altitude in bits 7-4 00169 00170 decimal = ( ( float )( lsb >> 4 ) ) / 16.0; 00171 //Altitude = ( float )( ( msb << 8 ) | csb ) + decimal; 00172 Altitude = ( float )( ( int16_t )( ( msb << 8 ) | csb ) ) + decimal; 00173 00174 write( CTRL_REG4, 0x00 ); 00175 00176 return( Altitude ); 00177 } 00178 00179 void MPL3115A2::SetModeAltimeter( void ) 00180 { 00181 SetModeStandby( ); 00182 00183 ctrl_reg1.bits.ALT = 1; 00184 write(CTRL_REG1, ctrl_reg1.octet); 00185 00186 SetModeActive( ); 00187 } 00188 00189 void MPL3115A2::SetModeBarometer(void) 00190 { 00191 SetModeStandby( ); 00192 00193 ctrl_reg1.bits.ALT = 0; 00194 write(CTRL_REG1, ctrl_reg1.octet); 00195 00196 SetModeActive( ); 00197 } 00198 00199 void MPL3115A2::ToggleOneShot( void ) 00200 { 00201 SetModeStandby( ); 00202 00203 ctrl_reg1.bits.OST = 0; 00204 write(CTRL_REG1, ctrl_reg1.octet); 00205 00206 ctrl_reg1.bits.OST = 1; 00207 write(CTRL_REG1, ctrl_reg1.octet); 00208 00209 SetModeActive( ); 00210 } 00211 00212 float MPL3115A2::ReadTemperature( void ) 00213 { 00214 uint8_t counter = 0; 00215 bool negSign = false; 00216 uint8_t val = 0; 00217 uint8_t msb = 0, lsb = 0; 00218 00219 /*if( MPL3115Initialized == false ) 00220 { 00221 return 0; 00222 }*/ 00223 00224 ToggleOneShot( ); 00225 00226 while( ( val & 0x02 ) != 0x02 ) 00227 { 00228 val = read( STATUS_REG); 00229 wait(0.01); 00230 counter++; 00231 00232 if( counter > 20 ) 00233 { 00234 //MPL3115Initialized = false; 00235 init( ); 00236 ToggleOneShot( ); 00237 counter = 0; 00238 while( ( val & 0x02 ) != 0x02 ) 00239 { 00240 val = read( STATUS_REG); 00241 wait(0.01); 00242 counter++; 00243 00244 if( counter > 20 ) 00245 { 00246 write( CTRL_REG4, 0x00 ); 00247 return( 0 ); //Error out after max of 512ms for a read 00248 } 00249 } 00250 00251 } 00252 } 00253 00254 msb = read( OUT_T_MSB_REG); // Integer part of temperature 00255 lsb = read( OUT_T_LSB_REG); // Decimal part of temperature in bits 7-4 00256 00257 if( msb > 0x7F ) 00258 { 00259 val = ~( ( msb << 8 ) + lsb ) + 1; //2?s complement 00260 msb = val >> 8; 00261 lsb = val & 0x00F0; 00262 negSign = true; 00263 } 00264 00265 if( negSign == true ) 00266 { 00267 Temperature = 0 - ( msb + ( float )( ( lsb >> 4 ) / 16.0 ) ); 00268 } 00269 else 00270 { 00271 Temperature = msb + ( float )( ( lsb >> 4 ) / 16.0 ); 00272 } 00273 00274 ToggleOneShot( ); 00275 00276 write( CTRL_REG4, 0x00 ); 00277 00278 return( Temperature ); 00279 } 00280 00281 void MPL3115A2::service() 00282 { 00283 mpl_int_source_t int_src; 00284 00285 if ((ctrl_reg4 == 0x00) || m_int_pin) // if no interrupts enabled and no interrupt occuring 00286 return; 00287 00288 int_src.octet = read(INT_SOURCE_REG); 00289 00290 if (int_src.bits.SRC_TCHG) { 00291 } 00292 if (int_src.bits.SRC_PCHG) { 00293 } 00294 if (int_src.bits.SRC_TTH) { 00295 } 00296 if (int_src.bits.SRC_PTH) { 00297 } 00298 if (int_src.bits.SRC_TW) { 00299 } 00300 if (int_src.bits.SRC_PW) { 00301 } 00302 if (int_src.bits.SRC_FIFO) { 00303 read(F_STATUS_REG); 00304 } 00305 if (int_src.bits.SRC_DRDY) { 00306 read(STATUS_REG); 00307 00308 read( OUT_T_MSB_REG); // Integer part of temperature 00309 read( OUT_T_LSB_REG); // Decimal part of temperature in bits 7-4 00310 00311 read( OUT_P_MSB_REG); // High byte of integer part of altitude, 00312 read( OUT_P_CSB_REG); // Low byte of integer part of altitude 00313 read( OUT_P_LSB_REG); // Decimal part of altitude in bits 7-4 00314 } 00315 00316 }
Generated on Fri Jul 15 2022 13:13:16 by
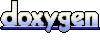