Timeout driver for event at absolute time
Dependents: demo_TimeoutAbs i2c_lora_slave
TimeoutAbs.h
00001 #ifndef _TOABS_H_ 00002 #define _TOABS_H_ 00003 #include "drivers/TimerEvent.h" 00004 #include "platform/Callback.h" 00005 #include "platform/mbed_toolchain.h" 00006 #include "platform/NonCopyable.h" 00007 #include "platform/mbed_power_mgmt.h" 00008 #include "hal/lp_ticker_api.h" 00009 #include "platform/mbed_critical.h" 00010 00011 namespace mbed { 00012 00013 class TimeoutAbs : public TimerEvent, private NonCopyable<TimeoutAbs> { 00014 public: 00015 TimeoutAbs() : TimerEvent(), _function(0), _lock_deepsleep(true) 00016 { 00017 } 00018 00019 TimeoutAbs(const ticker_data_t* data) : TimerEvent(data), _function(0), _lock_deepsleep(true) 00020 { 00021 #if DEVICE_LPTICKER 00022 _lock_deepsleep = (data != get_lp_ticker_data()); 00023 #endif 00024 } 00025 00026 virtual ~TimeoutAbs() 00027 { 00028 detach(); 00029 } 00030 00031 /** Detach the function 00032 */ 00033 void detach(); 00034 00035 void attach_us(Callback<void()> func, us_timestamp_t t) 00036 { 00037 core_util_critical_section_enter(); 00038 // lock only for the initial callback setup and this is not low power ticker 00039 if (!_function && _lock_deepsleep) { 00040 sleep_manager_lock_deep_sleep(); 00041 } 00042 _function = func; 00043 abs_setup(t); 00044 core_util_critical_section_exit(); 00045 } 00046 00047 us_timestamp_t read_us(void) 00048 { 00049 return ticker_read_us(_ticker_data); 00050 } 00051 00052 protected: 00053 void abs_setup(us_timestamp_t); 00054 virtual void handler(); 00055 Callback<void()> _function; /**< Callback. */ 00056 bool _lock_deepsleep; /**< Flag which indicates if deep sleep should be disabled. */ 00057 }; 00058 00059 } // namespace mbed 00060 #endif /* _TOABS_H_ */
Generated on Thu Jul 14 2022 01:54:54 by
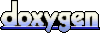