
test sending sensor results over lora radio. Accelerometer and temp/pressure.
Public functions
[Accelerometer]
Functions | |
DrvStatusTypeDef | BSP_ACCELERO_Init (ACCELERO_ID_t id, void **handle) |
Initialize an accelerometer sensor. | |
DrvStatusTypeDef | BSP_ACCELERO_DeInit (void **handle) |
Deinitialize accelerometer sensor. | |
DrvStatusTypeDef | BSP_ACCELERO_Sensor_Enable (void *handle) |
Enable accelerometer sensor. | |
DrvStatusTypeDef | BSP_ACCELERO_Sensor_Disable (void *handle) |
Disable accelerometer sensor. | |
DrvStatusTypeDef | BSP_ACCELERO_IsInitialized (void *handle, uint8_t *status) |
Check if the accelerometer sensor is initialized. | |
DrvStatusTypeDef | BSP_ACCELERO_IsEnabled (void *handle, uint8_t *status) |
Check if the accelerometer sensor is enabled. | |
DrvStatusTypeDef | BSP_ACCELERO_IsCombo (void *handle, uint8_t *status) |
Check if the accelerometer sensor is combo. | |
DrvStatusTypeDef | BSP_ACCELERO_Get_Instance (void *handle, uint8_t *instance) |
Get the accelerometer sensor instance. | |
DrvStatusTypeDef | BSP_ACCELERO_Get_WhoAmI (void *handle, uint8_t *who_am_i) |
Get the WHO_AM_I ID of the accelerometer sensor. | |
DrvStatusTypeDef | BSP_ACCELERO_Check_WhoAmI (void *handle) |
Check the WHO_AM_I ID of the accelerometer sensor. | |
DrvStatusTypeDef | BSP_ACCELERO_Get_Axes (void *handle, SensorAxes_t *acceleration) |
Get the accelerometer sensor axes. | |
DrvStatusTypeDef | BSP_ACCELERO_Get_AxesRaw (void *handle, SensorAxesRaw_t *value) |
Get the accelerometer sensor raw axes. | |
DrvStatusTypeDef | BSP_ACCELERO_Get_Sensitivity (void *handle, float *sensitivity) |
Get the accelerometer sensor sensitivity. | |
DrvStatusTypeDef | BSP_ACCELERO_Get_ODR (void *handle, float *odr) |
Get the accelerometer sensor output data rate. | |
DrvStatusTypeDef | BSP_ACCELERO_Set_ODR (void *handle, SensorOdr_t odr) |
Set the accelerometer sensor output data rate. | |
DrvStatusTypeDef | BSP_ACCELERO_Set_ODR_Value (void *handle, float odr) |
Set the accelerometer sensor output data rate. | |
DrvStatusTypeDef | BSP_ACCELERO_Get_FS (void *handle, float *fullScale) |
Get the accelerometer sensor full scale. | |
DrvStatusTypeDef | BSP_ACCELERO_Set_FS (void *handle, SensorFs_t fullScale) |
Set the accelerometer sensor full scale. | |
DrvStatusTypeDef | BSP_ACCELERO_Set_FS_Value (void *handle, float fullScale) |
Set the accelerometer sensor full scale. | |
DrvStatusTypeDef | BSP_ACCELERO_Get_Axes_Status (void *handle, uint8_t *xyz_enabled) |
Get the accelerometer sensor axes status. | |
DrvStatusTypeDef | BSP_ACCELERO_Set_Axes_Status (void *handle, uint8_t *enable_xyz) |
Set the enabled/disabled status of the accelerometer sensor axes. | |
DrvStatusTypeDef | BSP_ACCELERO_Read_Reg (void *handle, uint8_t reg, uint8_t *data) |
Read the data from register. | |
DrvStatusTypeDef | BSP_ACCELERO_Write_Reg (void *handle, uint8_t reg, uint8_t data) |
Write the data to register. | |
DrvStatusTypeDef | BSP_ACCELERO_Get_DRDY_Status (void *handle, uint8_t *status) |
Get accelerometer data ready status. |
Function Documentation
DrvStatusTypeDef BSP_ACCELERO_Check_WhoAmI | ( | void * | handle ) |
Check the WHO_AM_I ID of the accelerometer sensor.
- Parameters:
-
handle the device handle
- Return values:
-
COMPONENT_OK in case of success COMPONENT_ERROR in case of failure
Definition at line 376 of file x_nucleo_iks01a2_accelero.c.
DrvStatusTypeDef BSP_ACCELERO_DeInit | ( | void ** | handle ) |
Deinitialize accelerometer sensor.
- Parameters:
-
handle the device handle
- Return values:
-
COMPONENT_OK in case of success COMPONENT_ERROR in case of failure
Definition at line 127 of file x_nucleo_iks01a2_accelero.c.
DrvStatusTypeDef BSP_ACCELERO_Get_Axes | ( | void * | handle, |
SensorAxes_t * | acceleration | ||
) |
Get the accelerometer sensor axes.
- Parameters:
-
handle the device handle acceleration pointer where the values of the axes are written [mg]
- Return values:
-
COMPONENT_OK in case of success COMPONENT_ERROR in case of failure
Definition at line 411 of file x_nucleo_iks01a2_accelero.c.
DrvStatusTypeDef BSP_ACCELERO_Get_Axes_Status | ( | void * | handle, |
uint8_t * | xyz_enabled | ||
) |
Get the accelerometer sensor axes status.
- Parameters:
-
handle the device handle xyz_enabled the pointer to the axes enabled/disabled status
- Return values:
-
COMPONENT_OK in case of success COMPONENT_ERROR in case of failure
Definition at line 745 of file x_nucleo_iks01a2_accelero.c.
DrvStatusTypeDef BSP_ACCELERO_Get_AxesRaw | ( | void * | handle, |
SensorAxesRaw_t * | value | ||
) |
Get the accelerometer sensor raw axes.
- Parameters:
-
handle the device handle value pointer where the raw values of the axes are written
- Return values:
-
COMPONENT_OK in case of success COMPONENT_ERROR in case of failure
Definition at line 451 of file x_nucleo_iks01a2_accelero.c.
DrvStatusTypeDef BSP_ACCELERO_Get_DRDY_Status | ( | void * | handle, |
uint8_t * | status | ||
) |
Get accelerometer data ready status.
- Parameters:
-
handle the device handle status the data ready status
- Return values:
-
COMPONENT_OK in case of success COMPONENT_ERROR in case of failure
Definition at line 902 of file x_nucleo_iks01a2_accelero.c.
DrvStatusTypeDef BSP_ACCELERO_Get_FS | ( | void * | handle, |
float * | fullScale | ||
) |
Get the accelerometer sensor full scale.
- Parameters:
-
handle the device handle fullScale pointer where the full scale is written
- Return values:
-
COMPONENT_OK in case of success COMPONENT_ERROR in case of failure
Definition at line 637 of file x_nucleo_iks01a2_accelero.c.
DrvStatusTypeDef BSP_ACCELERO_Get_Instance | ( | void * | handle, |
uint8_t * | instance | ||
) |
Get the accelerometer sensor instance.
- Parameters:
-
handle the device handle instance the pointer to the device instance
- Return values:
-
COMPONENT_OK in case of success COMPONENT_ERROR in case of failure
Definition at line 314 of file x_nucleo_iks01a2_accelero.c.
DrvStatusTypeDef BSP_ACCELERO_Get_ODR | ( | void * | handle, |
float * | odr | ||
) |
Get the accelerometer sensor output data rate.
- Parameters:
-
handle the device handle odr pointer where the output data rate is written
- Return values:
-
COMPONENT_OK in case of success COMPONENT_ERROR in case of failure
Definition at line 529 of file x_nucleo_iks01a2_accelero.c.
DrvStatusTypeDef BSP_ACCELERO_Get_Sensitivity | ( | void * | handle, |
float * | sensitivity | ||
) |
Get the accelerometer sensor sensitivity.
- Parameters:
-
handle the device handle sensitivity pointer where the sensitivity value is written [mg/LSB]
- Return values:
-
COMPONENT_OK in case of success COMPONENT_ERROR in case of failure
Definition at line 489 of file x_nucleo_iks01a2_accelero.c.
DrvStatusTypeDef BSP_ACCELERO_Get_WhoAmI | ( | void * | handle, |
uint8_t * | who_am_i | ||
) |
Get the WHO_AM_I ID of the accelerometer sensor.
- Parameters:
-
handle the device handle who_am_i pointer to the value of WHO_AM_I register
- Return values:
-
COMPONENT_OK in case of success COMPONENT_ERROR in case of failure
Definition at line 342 of file x_nucleo_iks01a2_accelero.c.
DrvStatusTypeDef BSP_ACCELERO_Init | ( | ACCELERO_ID_t | id, |
void ** | handle | ||
) |
Initialize an accelerometer sensor.
- Parameters:
-
id the accelerometer sensor identifier handle the device handle
- Return values:
-
COMPONENT_OK in case of success COMPONENT_ERROR in case of failure
Definition at line 95 of file x_nucleo_iks01a2_accelero.c.
DrvStatusTypeDef BSP_ACCELERO_IsCombo | ( | void * | handle, |
uint8_t * | status | ||
) |
Check if the accelerometer sensor is combo.
- Parameters:
-
handle the device handle status the pointer to the combo status
- Return values:
-
COMPONENT_OK in case of success COMPONENT_ERROR in case of failure
Definition at line 287 of file x_nucleo_iks01a2_accelero.c.
DrvStatusTypeDef BSP_ACCELERO_IsEnabled | ( | void * | handle, |
uint8_t * | status | ||
) |
Check if the accelerometer sensor is enabled.
- Parameters:
-
handle the device handle status the pointer to the enable status
- Return values:
-
COMPONENT_OK in case of success COMPONENT_ERROR in case of failure
Definition at line 260 of file x_nucleo_iks01a2_accelero.c.
DrvStatusTypeDef BSP_ACCELERO_IsInitialized | ( | void * | handle, |
uint8_t * | status | ||
) |
Check if the accelerometer sensor is initialized.
- Parameters:
-
handle the device handle status the pointer to the initialization status
- Return values:
-
COMPONENT_OK in case of success COMPONENT_ERROR in case of failure
Definition at line 233 of file x_nucleo_iks01a2_accelero.c.
DrvStatusTypeDef BSP_ACCELERO_Read_Reg | ( | void * | handle, |
uint8_t | reg, | ||
uint8_t * | data | ||
) |
Read the data from register.
- Parameters:
-
handle the device handle reg register address data register data
- Return values:
-
COMPONENT_OK in case of success COMPONENT_ERROR in case of failure
Definition at line 826 of file x_nucleo_iks01a2_accelero.c.
DrvStatusTypeDef BSP_ACCELERO_Sensor_Disable | ( | void * | handle ) |
Disable accelerometer sensor.
- Parameters:
-
handle the device handle
- Return values:
-
COMPONENT_OK in case of success COMPONENT_ERROR in case of failure
Definition at line 199 of file x_nucleo_iks01a2_accelero.c.
DrvStatusTypeDef BSP_ACCELERO_Sensor_Enable | ( | void * | handle ) |
Enable accelerometer sensor.
- Parameters:
-
handle the device handle
- Return values:
-
COMPONENT_OK in case of success COMPONENT_ERROR in case of failure
Definition at line 165 of file x_nucleo_iks01a2_accelero.c.
DrvStatusTypeDef BSP_ACCELERO_Set_Axes_Status | ( | void * | handle, |
uint8_t * | enable_xyz | ||
) |
Set the enabled/disabled status of the accelerometer sensor axes.
- Parameters:
-
handle the device handle enable_xyz vector of the axes enabled/disabled status
- Return values:
-
COMPONENT_OK in case of success COMPONENT_ERROR in case of failure
Definition at line 785 of file x_nucleo_iks01a2_accelero.c.
DrvStatusTypeDef BSP_ACCELERO_Set_FS | ( | void * | handle, |
SensorFs_t | fullScale | ||
) |
Set the accelerometer sensor full scale.
- Parameters:
-
handle the device handle fullScale the functional full scale to be set
- Return values:
-
COMPONENT_OK in case of success COMPONENT_ERROR in case of failure
Definition at line 677 of file x_nucleo_iks01a2_accelero.c.
DrvStatusTypeDef BSP_ACCELERO_Set_FS_Value | ( | void * | handle, |
float | fullScale | ||
) |
Set the accelerometer sensor full scale.
- Parameters:
-
handle the device handle fullScale the full scale value to be set
- Return values:
-
COMPONENT_OK in case of success COMPONENT_ERROR in case of failure
Definition at line 711 of file x_nucleo_iks01a2_accelero.c.
DrvStatusTypeDef BSP_ACCELERO_Set_ODR | ( | void * | handle, |
SensorOdr_t | odr | ||
) |
Set the accelerometer sensor output data rate.
- Parameters:
-
handle the device handle odr the functional output data rate to be set
- Return values:
-
COMPONENT_OK in case of success COMPONENT_ERROR in case of failure
Definition at line 569 of file x_nucleo_iks01a2_accelero.c.
DrvStatusTypeDef BSP_ACCELERO_Set_ODR_Value | ( | void * | handle, |
float | odr | ||
) |
Set the accelerometer sensor output data rate.
- Parameters:
-
handle the device handle odr the output data rate value to be set
- Return values:
-
COMPONENT_OK in case of success COMPONENT_ERROR in case of failure
Definition at line 603 of file x_nucleo_iks01a2_accelero.c.
DrvStatusTypeDef BSP_ACCELERO_Write_Reg | ( | void * | handle, |
uint8_t | reg, | ||
uint8_t | data | ||
) |
Write the data to register.
- Parameters:
-
handle the device handle reg register address data register data
- Return values:
-
COMPONENT_OK in case of success COMPONENT_ERROR in case of failure
Definition at line 867 of file x_nucleo_iks01a2_accelero.c.
Generated on Tue Jul 12 2022 16:29:50 by
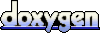