
test sending sensor results over lora radio. Accelerometer and temp/pressure.
Functions | |
static DrvStatusTypeDef | LPS22HH_P_Init (DrvContextTypeDef *handle) |
Initialize the LPS22HH pressure sensor. | |
static DrvStatusTypeDef | LPS22HH_P_DeInit (DrvContextTypeDef *handle) |
Deinitialize the LPS22HH pressure sensor. | |
static DrvStatusTypeDef | LPS22HH_P_Sensor_Enable (DrvContextTypeDef *handle) |
Enable the LPS22HH pressure sensor. | |
static DrvStatusTypeDef | LPS22HH_P_Sensor_Disable (DrvContextTypeDef *handle) |
Disable the LPS22HH pressure sensor. | |
static DrvStatusTypeDef | LPS22HH_P_Get_WhoAmI (DrvContextTypeDef *handle, uint8_t *who_am_i) |
Get the WHO_AM_I ID of the LPS22HH pressure sensor. | |
static DrvStatusTypeDef | LPS22HH_P_Check_WhoAmI (DrvContextTypeDef *handle) |
Check the WHO_AM_I ID of the LPS22HH pressure sensor. | |
static DrvStatusTypeDef | LPS22HH_P_Get_Press (DrvContextTypeDef *handle, float *pressure) |
Get the pressure value of the LPS22HH pressure sensor. | |
static DrvStatusTypeDef | LPS22HH_P_Get_ODR (DrvContextTypeDef *handle, float *odr) |
Get the LPS22HH pressure sensor output data rate. | |
static DrvStatusTypeDef | LPS22HH_P_Set_ODR (DrvContextTypeDef *handle, SensorOdr_t odr) |
Set the LPS22HH pressure sensor output data rate. | |
static DrvStatusTypeDef | LPS22HH_P_Set_ODR_Value (DrvContextTypeDef *handle, float odr) |
Set the LPS22HH pressure sensor output data rate. | |
static DrvStatusTypeDef | LPS22HH_P_Read_Reg (DrvContextTypeDef *handle, uint8_t reg, uint8_t *data) |
Read the data from register. | |
static DrvStatusTypeDef | LPS22HH_P_Write_Reg (DrvContextTypeDef *handle, uint8_t reg, uint8_t data) |
Write the data to register. | |
static DrvStatusTypeDef | LPS22HH_P_Get_DRDY_Status (DrvContextTypeDef *handle, uint8_t *status) |
Get pressure data ready status. | |
static DrvStatusTypeDef | LPS22HH_T_Init (DrvContextTypeDef *handle) |
Initialize the LPS22HH temperature sensor. | |
static DrvStatusTypeDef | LPS22HH_T_DeInit (DrvContextTypeDef *handle) |
Denitialize the LPS22HH temperature sensor. | |
static DrvStatusTypeDef | LPS22HH_T_Sensor_Enable (DrvContextTypeDef *handle) |
Enable the LPS22HH temperature sensor. | |
static DrvStatusTypeDef | LPS22HH_T_Sensor_Disable (DrvContextTypeDef *handle) |
Disable the LPS22HH temperature sensor. | |
static DrvStatusTypeDef | LPS22HH_T_Get_WhoAmI (DrvContextTypeDef *handle, uint8_t *who_am_i) |
Get the WHO_AM_I ID of the LPS22HH temperature sensor. | |
static DrvStatusTypeDef | LPS22HH_T_Check_WhoAmI (DrvContextTypeDef *handle) |
Check the WHO_AM_I ID of the LPS22HH temperature sensor. | |
static DrvStatusTypeDef | LPS22HH_T_Get_Temp (DrvContextTypeDef *handle, float *temperature) |
Get the temperature value of the LPS22HH temperature sensor. | |
static DrvStatusTypeDef | LPS22HH_T_Get_ODR (DrvContextTypeDef *handle, float *odr) |
Get the LPS22HH temperature sensor output data rate. | |
static DrvStatusTypeDef | LPS22HH_T_Set_ODR (DrvContextTypeDef *handle, SensorOdr_t odr) |
Set the LPS22HH temperature sensor output data rate. | |
static DrvStatusTypeDef | LPS22HH_T_Set_ODR_Value (DrvContextTypeDef *handle, float odr) |
Set the LPS22HH temperature sensor output data rate. | |
static DrvStatusTypeDef | LPS22HH_T_Read_Reg (DrvContextTypeDef *handle, uint8_t reg, uint8_t *data) |
Read the data from register. | |
static DrvStatusTypeDef | LPS22HH_T_Write_Reg (DrvContextTypeDef *handle, uint8_t reg, uint8_t data) |
Write the data to register. | |
static DrvStatusTypeDef | LPS22HH_T_Get_DRDY_Status (DrvContextTypeDef *handle, uint8_t *status) |
Get temperature data ready status. |
Function Documentation
static DrvStatusTypeDef LPS22HH_P_Check_WhoAmI | ( | DrvContextTypeDef * | handle ) | [static] |
Check the WHO_AM_I ID of the LPS22HH pressure sensor.
- Parameters:
-
handle the device handle
- Return values:
-
COMPONENT_OK in case of success COMPONENT_ERROR in case of failure
Definition at line 319 of file LPS22HH_Driver_HL.c.
static DrvStatusTypeDef LPS22HH_P_DeInit | ( | DrvContextTypeDef * | handle ) | [static] |
Deinitialize the LPS22HH pressure sensor.
- Parameters:
-
handle the device handle
- Return values:
-
COMPONENT_OK in case of success COMPONENT_ERROR in case of failure
Definition at line 211 of file LPS22HH_Driver_HL.c.
static DrvStatusTypeDef LPS22HH_P_Get_DRDY_Status | ( | DrvContextTypeDef * | handle, |
uint8_t * | status | ||
) | [static] |
Get pressure data ready status.
- Parameters:
-
handle the device handle status the data ready status
- Return values:
-
COMPONENT_OK in case of success COMPONENT_ERROR in case of failure
Definition at line 456 of file LPS22HH_Driver_HL.c.
static DrvStatusTypeDef LPS22HH_P_Get_ODR | ( | DrvContextTypeDef * | handle, |
float * | odr | ||
) | [static] |
Get the LPS22HH pressure sensor output data rate.
- Parameters:
-
handle the device handle odr pointer where the output data rate is written
- Return values:
-
COMPONENT_OK in case of success COMPONENT_ERROR in case of failure
Definition at line 345 of file LPS22HH_Driver_HL.c.
static DrvStatusTypeDef LPS22HH_P_Get_Press | ( | DrvContextTypeDef * | handle, |
float * | pressure | ||
) | [static] |
Get the pressure value of the LPS22HH pressure sensor.
- Parameters:
-
handle the device handle pressure pointer where the value is written
- Return values:
-
COMPONENT_OK in case of success COMPONENT_ERROR in case of failure
Definition at line 332 of file LPS22HH_Driver_HL.c.
static DrvStatusTypeDef LPS22HH_P_Get_WhoAmI | ( | DrvContextTypeDef * | handle, |
uint8_t * | who_am_i | ||
) | [static] |
Get the WHO_AM_I ID of the LPS22HH pressure sensor.
- Parameters:
-
handle the device handle who_am_i pointer to the value of WHO_AM_I register
- Return values:
-
COMPONENT_OK in case of success COMPONENT_ERROR in case of failure
Definition at line 307 of file LPS22HH_Driver_HL.c.
static DrvStatusTypeDef LPS22HH_P_Init | ( | DrvContextTypeDef * | handle ) | [static] |
Initialize the LPS22HH pressure sensor.
- Parameters:
-
handle the device handle
- Return values:
-
COMPONENT_OK in case of success COMPONENT_ERROR in case of failure
Definition at line 182 of file LPS22HH_Driver_HL.c.
static DrvStatusTypeDef LPS22HH_P_Read_Reg | ( | DrvContextTypeDef * | handle, |
uint8_t | reg, | ||
uint8_t * | data | ||
) | [static] |
Read the data from register.
- Parameters:
-
handle the device handle reg register address data register data
- Return values:
-
COMPONENT_OK in case of success COMPONENT_ERROR in case of failure
Definition at line 421 of file LPS22HH_Driver_HL.c.
static DrvStatusTypeDef LPS22HH_P_Sensor_Disable | ( | DrvContextTypeDef * | handle ) | [static] |
Disable the LPS22HH pressure sensor.
- Parameters:
-
handle the device handle
- Return values:
-
COMPONENT_OK in case of success COMPONENT_ERROR in case of failure
Definition at line 270 of file LPS22HH_Driver_HL.c.
static DrvStatusTypeDef LPS22HH_P_Sensor_Enable | ( | DrvContextTypeDef * | handle ) | [static] |
Enable the LPS22HH pressure sensor.
- Parameters:
-
handle the device handle
- Return values:
-
COMPONENT_OK in case of success COMPONENT_ERROR in case of failure
Definition at line 240 of file LPS22HH_Driver_HL.c.
static DrvStatusTypeDef LPS22HH_P_Set_ODR | ( | DrvContextTypeDef * | handle, |
SensorOdr_t | odr | ||
) | [static] |
Set the LPS22HH pressure sensor output data rate.
- Parameters:
-
handle the device handle odr the functional output data rate to be set
- Return values:
-
COMPONENT_OK in case of success COMPONENT_ERROR in case of failure
Definition at line 358 of file LPS22HH_Driver_HL.c.
static DrvStatusTypeDef LPS22HH_P_Set_ODR_Value | ( | DrvContextTypeDef * | handle, |
float | odr | ||
) | [static] |
Set the LPS22HH pressure sensor output data rate.
- Parameters:
-
handle the device handle odr the output data rate value to be set
- Return values:
-
COMPONENT_OK in case of success COMPONENT_ERROR in case of failure
Definition at line 389 of file LPS22HH_Driver_HL.c.
static DrvStatusTypeDef LPS22HH_P_Write_Reg | ( | DrvContextTypeDef * | handle, |
uint8_t | reg, | ||
uint8_t | data | ||
) | [static] |
Write the data to register.
- Parameters:
-
handle the device handle reg register address data register data
- Return values:
-
COMPONENT_OK in case of success COMPONENT_ERROR in case of failure
Definition at line 439 of file LPS22HH_Driver_HL.c.
static DrvStatusTypeDef LPS22HH_T_Check_WhoAmI | ( | DrvContextTypeDef * | handle ) | [static] |
Check the WHO_AM_I ID of the LPS22HH temperature sensor.
- Parameters:
-
handle the device handle
- Return values:
-
COMPONENT_OK in case of success COMPONENT_ERROR in case of failure
Definition at line 611 of file LPS22HH_Driver_HL.c.
static DrvStatusTypeDef LPS22HH_T_DeInit | ( | DrvContextTypeDef * | handle ) | [static] |
Denitialize the LPS22HH temperature sensor.
- Parameters:
-
handle the device handle
- Return values:
-
COMPONENT_OK in case of success COMPONENT_ERROR in case of failure
Definition at line 503 of file LPS22HH_Driver_HL.c.
static DrvStatusTypeDef LPS22HH_T_Get_DRDY_Status | ( | DrvContextTypeDef * | handle, |
uint8_t * | status | ||
) | [static] |
Get temperature data ready status.
- Parameters:
-
handle the device handle status the data ready status
- Return values:
-
COMPONENT_OK in case of success COMPONENT_ERROR in case of failure
Definition at line 750 of file LPS22HH_Driver_HL.c.
static DrvStatusTypeDef LPS22HH_T_Get_ODR | ( | DrvContextTypeDef * | handle, |
float * | odr | ||
) | [static] |
Get the LPS22HH temperature sensor output data rate.
- Parameters:
-
handle the device handle odr pointer where the output data rate is written
- Return values:
-
COMPONENT_OK in case of success COMPONENT_ERROR in case of failure
Definition at line 637 of file LPS22HH_Driver_HL.c.
static DrvStatusTypeDef LPS22HH_T_Get_Temp | ( | DrvContextTypeDef * | handle, |
float * | temperature | ||
) | [static] |
Get the temperature value of the LPS22HH temperature sensor.
- Parameters:
-
handle the device handle temperature pointer where the value is written
- Return values:
-
COMPONENT_OK in case of success COMPONENT_ERROR in case of failure
Definition at line 624 of file LPS22HH_Driver_HL.c.
static DrvStatusTypeDef LPS22HH_T_Get_WhoAmI | ( | DrvContextTypeDef * | handle, |
uint8_t * | who_am_i | ||
) | [static] |
Get the WHO_AM_I ID of the LPS22HH temperature sensor.
- Parameters:
-
handle the device handle who_am_i pointer to the value of WHO_AM_I register
- Return values:
-
COMPONENT_OK in case of success COMPONENT_ERROR in case of failure
Definition at line 599 of file LPS22HH_Driver_HL.c.
static DrvStatusTypeDef LPS22HH_T_Init | ( | DrvContextTypeDef * | handle ) | [static] |
Initialize the LPS22HH temperature sensor.
- Parameters:
-
handle the device handle
- Return values:
-
COMPONENT_OK in case of success COMPONENT_ERROR in case of failure
Definition at line 474 of file LPS22HH_Driver_HL.c.
static DrvStatusTypeDef LPS22HH_T_Read_Reg | ( | DrvContextTypeDef * | handle, |
uint8_t | reg, | ||
uint8_t * | data | ||
) | [static] |
Read the data from register.
- Parameters:
-
handle the device handle reg register address data register data
- Return values:
-
COMPONENT_OK in case of success COMPONENT_ERROR in case of failure
Definition at line 713 of file LPS22HH_Driver_HL.c.
static DrvStatusTypeDef LPS22HH_T_Sensor_Disable | ( | DrvContextTypeDef * | handle ) | [static] |
Disable the LPS22HH temperature sensor.
- Parameters:
-
handle the device handle
- Return values:
-
COMPONENT_OK in case of success COMPONENT_ERROR in case of failure
Definition at line 562 of file LPS22HH_Driver_HL.c.
static DrvStatusTypeDef LPS22HH_T_Sensor_Enable | ( | DrvContextTypeDef * | handle ) | [static] |
Enable the LPS22HH temperature sensor.
- Parameters:
-
handle the device handle
- Return values:
-
COMPONENT_OK in case of success COMPONENT_ERROR in case of failure
Definition at line 532 of file LPS22HH_Driver_HL.c.
static DrvStatusTypeDef LPS22HH_T_Set_ODR | ( | DrvContextTypeDef * | handle, |
SensorOdr_t | odr | ||
) | [static] |
Set the LPS22HH temperature sensor output data rate.
- Parameters:
-
handle the device handle odr the functional output data rate to be set
- Return values:
-
COMPONENT_OK in case of success COMPONENT_ERROR in case of failure
Definition at line 650 of file LPS22HH_Driver_HL.c.
static DrvStatusTypeDef LPS22HH_T_Set_ODR_Value | ( | DrvContextTypeDef * | handle, |
float | odr | ||
) | [static] |
Set the LPS22HH temperature sensor output data rate.
- Parameters:
-
handle the device handle odr the output data rate value to be set
- Return values:
-
COMPONENT_OK in case of success COMPONENT_ERROR in case of failure
Definition at line 681 of file LPS22HH_Driver_HL.c.
static DrvStatusTypeDef LPS22HH_T_Write_Reg | ( | DrvContextTypeDef * | handle, |
uint8_t | reg, | ||
uint8_t | data | ||
) | [static] |
Write the data to register.
- Parameters:
-
handle the device handle reg register address data register data
- Return values:
-
COMPONENT_OK in case of success COMPONENT_ERROR in case of failure
Definition at line 732 of file LPS22HH_Driver_HL.c.
Generated on Tue Jul 12 2022 16:29:50 by
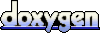