Lib for FTDI FT800 graphic controller "EVE" The API is changed from the FTDI original names. It use smaller names now. DL() will add something to the display list instead of Ft_App_WrCoCmd_Buffer ... The FTDI programmer Guide is also using this commands.
Dependents: FT800_RGB_demo FT800_RGB_demo2 FT800_demo_for_habr Temp_&_RH_at_TFT-demo ... more
Fork of FT800 by
FT_Hal_Utils.cpp
00001 #include "FT_Platform.h" 00002 #include "mbed.h" 00003 00004 /* function to load jpg file from filesystem */ 00005 /* return 0 if jpg is ok */ 00006 /* return x_size and y_size of jpg */ 00007 00008 int FT800::Load_jpg(char* filename, ft_int16_t* x_size, ft_int16_t* y_size) 00009 { 00010 unsigned char pbuff[8291]; 00011 unsigned short marker; 00012 unsigned short length; 00013 unsigned char data[4]; 00014 00015 ft_uint16_t blocklen; 00016 00017 FILE *fp = fopen(filename, "r"); 00018 if(fp == NULL) return (-1); // connot open file 00019 00020 // search for 0xFFC0 marker 00021 fseek(fp, 0, SEEK_END); 00022 unsigned int Fsize = ftell(fp); 00023 fseek(fp, 2, SEEK_SET); 00024 fread(data,4,1,fp); 00025 marker = data[0] << 8 | data[1]; 00026 length = data[2] << 8 | data[3]; 00027 do { 00028 if(marker == 0xFFC0) break; 00029 if(marker & 0xFF00 != 0xFF00) break; 00030 if (fseek(fp, length - 2,SEEK_CUR) != 0) break; 00031 fread(data,4,1,fp); 00032 marker = data[0] << 8 | data[1]; 00033 length = data[2] << 8 | data[3]; 00034 } while(1); 00035 if(marker != 0xFFC0) return (-2); // no FFC0 Marker, wrong format no baseline DCT-based JPEG 00036 fseek(fp, 1,SEEK_CUR); 00037 fread(data,4,1,fp); 00038 *y_size = (data[0] << 8 | data[1]); 00039 *x_size = (data[2] << 8 | data[3]); 00040 00041 //if(*x_size > DispWidth || *y_size > DispHeight) return (-3); // to big to fit on screen 00042 00043 fseek(fp, 0, SEEK_SET); 00044 WrCmd32(CMD_LOADIMAGE); // load a JPEG image 00045 WrCmd32(0); //destination address of jpg decode 00046 WrCmd32(0); //output format of the bitmap - default is rgb565 00047 while(Fsize > 0) { 00048 /* download the data into the command buffer by 8kb one shot */ 00049 blocklen = Fsize>8192?8192:Fsize; 00050 /* copy the data into pbuff and then transfter it to command buffer */ 00051 fread(pbuff,1,blocklen,fp); 00052 Fsize -= blocklen; 00053 /* copy data continuously into command memory */ 00054 WrCmdBuf(pbuff, blocklen); //alignment is already taken care by this api 00055 } 00056 fclose(fp); 00057 00058 return(0); 00059 } 00060 00061 00062 /* calibrate touch */ 00063 ft_void_t FT800::Calibrate() 00064 { 00065 /*************************************************************************/ 00066 /* Below code demonstrates the usage of calibrate function. Calibrate */ 00067 /* function will wait untill user presses all the three dots. Only way to*/ 00068 /* come out of this api is to reset the coprocessor bit. */ 00069 /*************************************************************************/ 00070 { 00071 00072 DLstart(); // start a new display command list 00073 DL(CLEAR_COLOR_RGB(64,64,64)); // set the clear color R, G, B 00074 DL(CLEAR(1,1,1)); // clear buffers -> color buffer,stencil buffer, tag buffer 00075 DL(COLOR_RGB(0xff,0xff,0xff)); // set the current color R, G, B 00076 Text((DispWidth/2), (DispHeight/2), 27, OPT_CENTER, "Please Tap on the dot"); // draw Text at x,y, font 27, centered 00077 Calibrate(0); // start the calibration of touch screen 00078 Flush_Co_Buffer(); // download the commands into FT800 FIFO 00079 WaitCmdfifo_empty(); // Wait till coprocessor completes the operation 00080 } 00081 } 00082 00083 00084 /* API to give fadeout effect by changing the display PWM from 100 till 0 */ 00085 ft_void_t FT800::fadeout() 00086 { 00087 ft_int32_t i; 00088 00089 for (i = 100; i >= 0; i -= 3) 00090 { 00091 Wr8(REG_PWM_DUTY,i); 00092 Sleep(2);//sleep for 2 ms 00093 } 00094 } 00095 00096 /* API to perform display fadein effect by changing the display PWM from 0 till 100 and finally 128 */ 00097 ft_void_t FT800::fadein() 00098 { 00099 ft_int32_t i; 00100 00101 for (i = 0; i <=100 ; i += 3) 00102 { 00103 Wr8(REG_PWM_DUTY,i); 00104 Sleep(2);//sleep for 2 ms 00105 } 00106 /* Finally make the PWM 100% */ 00107 i = 128; 00108 Wr8(REG_PWM_DUTY,i); 00109 } 00110 00111 ft_void_t FT800::read_calibrate(ft_uint8_t data[24]){ 00112 unsigned int i; 00113 for(i=0;i<24;i++){ 00114 data[i] = Rd8(REG_TOUCH_TRANSFORM_A + i); 00115 } 00116 } 00117 00118 ft_void_t FT800::write_calibrate(ft_uint8_t data[24]){ 00119 unsigned int i; 00120 for(i=0;i<24;i++) { 00121 Wr8(REG_TOUCH_TRANSFORM_A + i,data[i]); 00122 } 00123 } 00124 00125 ft_uint32_t FT800::color_rgb(ft_uint8_t red,ft_uint8_t green, ft_uint8_t blue){ 00126 return ((4UL<<24)|(((red)&255UL)<<16)|(((green)&255UL)<<8)|(((blue)&255UL)<<0)); 00127 } 00128 00129 ft_uint32_t FT800::clear_color_rgb(ft_uint8_t red,ft_uint8_t green, ft_uint8_t blue){ 00130 return ((2UL<<24)|(((red)&255UL)<<16)|(((green)&255UL)<<8)|(((blue)&255UL)<<0)); 00131 } 00132
Generated on Tue Jul 12 2022 19:49:40 by
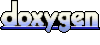