Lib for FTDI FT800 graphic controller "EVE" The API is changed from the FTDI original names. It use smaller names now. DL() will add something to the display list instead of Ft_App_WrCoCmd_Buffer ... The FTDI programmer Guide is also using this commands.
Dependents: FT800_RGB_demo FT800_RGB_demo2 FT800_demo_for_habr Temp_&_RH_at_TFT-demo ... more
Fork of FT800 by
FT_CoPro_Cmds.cpp
00001 /* mbed Library for FTDI FT800 Enbedded Video Engine "EVE" 00002 * based on Original Code Sample from FTDI 00003 * ported to mbed by Peter Drescher, DC2PD 2014 00004 * Released under the MIT License: http://mbed.org/license/mit */ 00005 00006 #include "FT_Platform.h" 00007 00008 00009 ft_void_t FT800::SendCmd( ft_uint32_t cmd) 00010 { 00011 Transfer32( cmd); 00012 } 00013 00014 ft_void_t FT800::SendStr( const ft_char8_t *s) 00015 { 00016 TransferString( s); 00017 } 00018 00019 00020 ft_void_t FT800::StartFunc( ft_uint16_t count) 00021 { 00022 CheckCmdBuffer( count); 00023 StartCmdTransfer( FT_GPU_WRITE,count); 00024 } 00025 00026 ft_void_t FT800::EndFunc( ft_uint16_t count) 00027 { 00028 EndTransfer( ); 00029 Updatecmdfifo( count); 00030 } 00031 00032 ft_void_t FT800::Text( ft_int16_t x, ft_int16_t y, ft_int16_t font, ft_uint16_t options, const ft_char8_t* s) 00033 { 00034 StartFunc( FT_CMD_SIZE*3 + strlen(s) + 1); 00035 SendCmd( CMD_TEXT); 00036 //Copro_SendCmd( (((ft_uint32_t)y<<16)|(ft_uint32_t)x)); 00037 SendCmd( (((ft_uint32_t)y<<16)|(x & 0xffff))); 00038 SendCmd( (((ft_uint32_t)options<<16)|(ft_uint32_t)font)); 00039 SendStr( s); 00040 EndFunc( (FT_CMD_SIZE*3 + strlen(s) + 1)); 00041 } 00042 00043 ft_void_t FT800::Number( ft_int16_t x, ft_int16_t y, ft_int16_t font, ft_uint16_t options, ft_int32_t n) 00044 { 00045 StartFunc( FT_CMD_SIZE*4); 00046 SendCmd( CMD_NUMBER); 00047 SendCmd( (((ft_uint32_t)y<<16)|(x & 0xffff))); 00048 SendCmd( (((ft_uint32_t)options<<16)|font)); 00049 SendCmd( n); 00050 EndFunc( (FT_CMD_SIZE*4)); 00051 } 00052 00053 ft_void_t FT800::LoadIdentity( ) 00054 { 00055 StartFunc( FT_CMD_SIZE*1); 00056 SendCmd( CMD_LOADIDENTITY); 00057 EndFunc( (FT_CMD_SIZE*1)); 00058 } 00059 00060 ft_void_t FT800::Toggle( ft_int16_t x, ft_int16_t y, ft_int16_t w, ft_int16_t font, ft_uint16_t options, ft_uint16_t state, const ft_char8_t* s) 00061 { 00062 StartFunc( FT_CMD_SIZE*4 + strlen(s) + 1); 00063 SendCmd( CMD_TOGGLE); 00064 SendCmd( (((ft_uint32_t)y<<16)|(x & 0xffff))); 00065 SendCmd( (((ft_uint32_t)font<<16)|w)); 00066 SendCmd( (((ft_uint32_t)state<<16)|options)); 00067 SendStr( s); 00068 EndFunc( (FT_CMD_SIZE*4 + strlen(s) + 1)); 00069 } 00070 00071 /* Error handling for val is not done, so better to always use range of 65535 in order that needle is drawn within display region */ 00072 ft_void_t FT800::Gauge( ft_int16_t x, ft_int16_t y, ft_int16_t r, ft_uint16_t options, ft_uint16_t major, ft_uint16_t minor, ft_uint16_t val, ft_uint16_t range) 00073 { 00074 StartFunc( FT_CMD_SIZE*5); 00075 SendCmd( CMD_GAUGE); 00076 SendCmd( (((ft_uint32_t)y<<16)|(x & 0xffff))); 00077 SendCmd( (((ft_uint32_t)options<<16)|r)); 00078 SendCmd( (((ft_uint32_t)minor<<16)|major)); 00079 SendCmd( (((ft_uint32_t)range<<16)|val)); 00080 EndFunc( (FT_CMD_SIZE*5)); 00081 } 00082 00083 ft_void_t FT800::RegRead( ft_uint32_t ptr, ft_uint32_t result) 00084 { 00085 StartFunc( FT_CMD_SIZE*3); 00086 SendCmd( CMD_REGREAD); 00087 SendCmd( ptr); 00088 SendCmd( 0); 00089 EndFunc( (FT_CMD_SIZE*3)); 00090 00091 } 00092 00093 ft_void_t FT800::GetProps( ft_uint32_t ptr, ft_uint32_t w, ft_uint32_t h) 00094 { 00095 StartFunc( FT_CMD_SIZE*4); 00096 SendCmd( CMD_GETPROPS); 00097 SendCmd( ptr); 00098 SendCmd( w); 00099 SendCmd( h); 00100 EndFunc( (FT_CMD_SIZE*4)); 00101 } 00102 00103 ft_void_t FT800::Memcpy( ft_uint32_t dest, ft_uint32_t src, ft_uint32_t num) 00104 { 00105 StartFunc( FT_CMD_SIZE*4); 00106 SendCmd( CMD_MEMCPY); 00107 SendCmd( dest); 00108 SendCmd( src); 00109 SendCmd( num); 00110 EndFunc( (FT_CMD_SIZE*4)); 00111 } 00112 00113 ft_void_t FT800::Spinner( ft_int16_t x, ft_int16_t y, ft_uint16_t style, ft_uint16_t scale) 00114 { 00115 StartFunc( FT_CMD_SIZE*3); 00116 SendCmd( CMD_SPINNER); 00117 SendCmd( (((ft_uint32_t)y<<16)|(x & 0xffff))); 00118 SendCmd( (((ft_uint32_t)scale<<16)|style)); 00119 EndFunc( (FT_CMD_SIZE*3)); 00120 } 00121 00122 ft_void_t FT800::BgColor( ft_uint32_t c) 00123 { 00124 StartFunc( FT_CMD_SIZE*2); 00125 SendCmd( CMD_BGCOLOR); 00126 SendCmd( c); 00127 EndFunc( (FT_CMD_SIZE*2)); 00128 } 00129 00130 ft_void_t FT800::Swap() 00131 { 00132 StartFunc( FT_CMD_SIZE*1); 00133 SendCmd( CMD_SWAP); 00134 EndFunc( (FT_CMD_SIZE*1)); 00135 } 00136 00137 ft_void_t FT800::Inflate( ft_uint32_t ptr) 00138 { 00139 StartFunc( FT_CMD_SIZE*2); 00140 SendCmd( CMD_INFLATE); 00141 SendCmd( ptr); 00142 EndFunc( (FT_CMD_SIZE*2)); 00143 } 00144 00145 ft_void_t FT800::Translate( ft_int32_t tx, ft_int32_t ty) 00146 { 00147 StartFunc( FT_CMD_SIZE*3); 00148 SendCmd( CMD_TRANSLATE); 00149 SendCmd( tx); 00150 SendCmd( ty); 00151 EndFunc( (FT_CMD_SIZE*3)); 00152 } 00153 00154 ft_void_t FT800::Stop() 00155 { 00156 StartFunc( FT_CMD_SIZE*1); 00157 SendCmd( CMD_STOP); 00158 EndFunc( (FT_CMD_SIZE*1)); 00159 } 00160 00161 ft_void_t FT800::Slider( ft_int16_t x, ft_int16_t y, ft_int16_t w, ft_int16_t h, ft_uint16_t options, ft_uint16_t val, ft_uint16_t range) 00162 { 00163 StartFunc( FT_CMD_SIZE*5); 00164 SendCmd( CMD_SLIDER); 00165 SendCmd( (((ft_uint32_t)y<<16)|(x & 0xffff))); 00166 SendCmd( (((ft_uint32_t)h<<16)|w)); 00167 SendCmd( (((ft_uint32_t)val<<16)|options)); 00168 SendCmd( range); 00169 EndFunc( (FT_CMD_SIZE*5)); 00170 } 00171 00172 ft_void_t FT800::TouchTransform( ft_int32_t x0, ft_int32_t y0, ft_int32_t x1, ft_int32_t y1, ft_int32_t x2, ft_int32_t y2, ft_int32_t tx0, ft_int32_t ty0, ft_int32_t tx1, ft_int32_t ty1, ft_int32_t tx2, ft_int32_t ty2, ft_uint16_t result) 00173 { 00174 StartFunc( FT_CMD_SIZE*6*2+FT_CMD_SIZE*2); 00175 SendCmd( CMD_TOUCH_TRANSFORM); 00176 SendCmd( x0); 00177 SendCmd( y0); 00178 SendCmd( x1); 00179 SendCmd( y1); 00180 SendCmd( x2); 00181 SendCmd( y2); 00182 SendCmd( tx0); 00183 SendCmd( ty0); 00184 SendCmd( tx1); 00185 SendCmd( ty1); 00186 SendCmd( tx2); 00187 SendCmd( ty2); 00188 SendCmd( result); 00189 EndFunc( (FT_CMD_SIZE*6*2+FT_CMD_SIZE*2)); 00190 } 00191 00192 ft_void_t FT800::Interrupt( ft_uint32_t ms) 00193 { 00194 StartFunc( FT_CMD_SIZE*2); 00195 SendCmd( CMD_INTERRUPT); 00196 SendCmd( ms); 00197 EndFunc( (FT_CMD_SIZE*2)); 00198 } 00199 00200 ft_void_t FT800::FgColor( ft_uint32_t c) 00201 { 00202 StartFunc( FT_CMD_SIZE*2); 00203 SendCmd( CMD_FGCOLOR); 00204 SendCmd( c); 00205 EndFunc( (FT_CMD_SIZE*2)); 00206 } 00207 00208 ft_void_t FT800::Rotate( ft_int32_t a) 00209 { 00210 StartFunc( FT_CMD_SIZE*2); 00211 SendCmd( CMD_ROTATE); 00212 SendCmd( a); 00213 EndFunc( (FT_CMD_SIZE*2)); 00214 } 00215 00216 ft_void_t FT800::Button( ft_int16_t x, ft_int16_t y, ft_int16_t w, ft_int16_t h, ft_int16_t font, ft_uint16_t options, const ft_char8_t* s) 00217 { 00218 StartFunc( FT_CMD_SIZE*4 + strlen(s) + 1); 00219 SendCmd( CMD_BUTTON); 00220 SendCmd( (((ft_uint32_t)y<<16)|(x & 0xffff))); 00221 SendCmd( (((ft_uint32_t)h<<16)|w)); 00222 SendCmd( (((ft_uint32_t)options<<16)|font)); // patch from Ivano Pelicella to draw flat buttons 00223 SendStr( s); 00224 EndFunc( (FT_CMD_SIZE*4 + strlen(s) + 1)); 00225 } 00226 00227 ft_void_t FT800::MemWrite( ft_uint32_t ptr, ft_uint32_t num) 00228 { 00229 StartFunc( FT_CMD_SIZE*3); 00230 SendCmd( CMD_MEMWRITE); 00231 SendCmd( ptr); 00232 SendCmd( num); 00233 EndFunc( (FT_CMD_SIZE*3)); 00234 } 00235 00236 ft_void_t FT800::Scrollbar( ft_int16_t x, ft_int16_t y, ft_int16_t w, ft_int16_t h, ft_uint16_t options, ft_uint16_t val, ft_uint16_t size, ft_uint16_t range) 00237 { 00238 StartFunc( FT_CMD_SIZE*5); 00239 SendCmd( CMD_SCROLLBAR); 00240 SendCmd( (((ft_uint32_t)y<<16)|(x & 0xffff))); 00241 SendCmd( (((ft_uint32_t)h<<16)|w)); 00242 SendCmd( (((ft_uint32_t)val<<16)|options)); 00243 SendCmd( (((ft_uint32_t)range<<16)|size)); 00244 EndFunc( (FT_CMD_SIZE*5)); 00245 } 00246 00247 ft_void_t FT800::GetMatrix( ft_int32_t a, ft_int32_t b, ft_int32_t c, ft_int32_t d, ft_int32_t e, ft_int32_t f) 00248 { 00249 StartFunc( FT_CMD_SIZE*7); 00250 SendCmd( CMD_GETMATRIX); 00251 SendCmd( a); 00252 SendCmd( b); 00253 SendCmd( c); 00254 SendCmd( d); 00255 SendCmd( e); 00256 SendCmd( f); 00257 EndFunc( (FT_CMD_SIZE*7)); 00258 } 00259 00260 ft_void_t FT800::Sketch( ft_int16_t x, ft_int16_t y, ft_uint16_t w, ft_uint16_t h, ft_uint32_t ptr, ft_uint16_t format) 00261 { 00262 StartFunc( FT_CMD_SIZE*5); 00263 SendCmd( CMD_SKETCH); 00264 SendCmd( (((ft_uint32_t)y<<16)|(x & 0xffff))); 00265 SendCmd( (((ft_uint32_t)h<<16)|w)); 00266 SendCmd( ptr); 00267 SendCmd( format); 00268 EndFunc( (FT_CMD_SIZE*5)); 00269 } 00270 ft_void_t FT800::MemSet( ft_uint32_t ptr, ft_uint32_t value, ft_uint32_t num) 00271 { 00272 StartFunc( FT_CMD_SIZE*4); 00273 SendCmd( CMD_MEMSET); 00274 SendCmd( ptr); 00275 SendCmd( value); 00276 SendCmd( num); 00277 EndFunc( (FT_CMD_SIZE*4)); 00278 } 00279 ft_void_t FT800::GradColor( ft_uint32_t c) 00280 { 00281 StartFunc( FT_CMD_SIZE*2); 00282 SendCmd( CMD_GRADCOLOR); 00283 SendCmd( c); 00284 EndFunc( (FT_CMD_SIZE*2)); 00285 } 00286 ft_void_t FT800::BitmapTransform( ft_int32_t x0, ft_int32_t y0, ft_int32_t x1, ft_int32_t y1, ft_int32_t x2, ft_int32_t y2, ft_int32_t tx0, ft_int32_t ty0, ft_int32_t tx1, ft_int32_t ty1, ft_int32_t tx2, ft_int32_t ty2, ft_uint16_t result) 00287 { 00288 StartFunc( FT_CMD_SIZE*6*2+FT_CMD_SIZE*2); 00289 SendCmd( CMD_BITMAP_TRANSFORM); 00290 SendCmd( x0); 00291 SendCmd( y0); 00292 SendCmd( x1); 00293 SendCmd( y1); 00294 SendCmd( x2); 00295 SendCmd( y2); 00296 SendCmd( tx0); 00297 SendCmd( ty0); 00298 SendCmd( tx1); 00299 SendCmd( ty1); 00300 SendCmd( tx2); 00301 SendCmd( ty2); 00302 SendCmd( result); 00303 EndFunc( (FT_CMD_SIZE*6*2+FT_CMD_SIZE*2)); 00304 } 00305 ft_void_t FT800::Calibrate( ft_uint32_t result) 00306 { 00307 StartFunc( FT_CMD_SIZE*2); 00308 SendCmd( CMD_CALIBRATE); 00309 SendCmd( result); 00310 EndFunc( (FT_CMD_SIZE*2)); 00311 WaitCmdfifo_empty( ); 00312 00313 } 00314 ft_void_t FT800::SetFont( ft_uint32_t font, ft_uint32_t ptr) 00315 { 00316 StartFunc( FT_CMD_SIZE*3); 00317 SendCmd( CMD_SETFONT); 00318 SendCmd( font); 00319 SendCmd( ptr); 00320 EndFunc( (FT_CMD_SIZE*3)); 00321 } 00322 ft_void_t FT800::Logo( ) 00323 { 00324 StartFunc( FT_CMD_SIZE*1); 00325 SendCmd( CMD_LOGO); 00326 EndFunc( FT_CMD_SIZE*1); 00327 } 00328 ft_void_t FT800::Append( ft_uint32_t ptr, ft_uint32_t num) 00329 { 00330 StartFunc( FT_CMD_SIZE*3); 00331 SendCmd( CMD_APPEND); 00332 SendCmd( ptr); 00333 SendCmd( num); 00334 EndFunc( (FT_CMD_SIZE*3)); 00335 } 00336 ft_void_t FT800::MemZero( ft_uint32_t ptr, ft_uint32_t num) 00337 { 00338 StartFunc( FT_CMD_SIZE*3); 00339 SendCmd( CMD_MEMZERO); 00340 SendCmd( ptr); 00341 SendCmd( num); 00342 EndFunc( (FT_CMD_SIZE*3)); 00343 } 00344 ft_void_t FT800::Scale( ft_int32_t sx, ft_int32_t sy) 00345 { 00346 StartFunc( FT_CMD_SIZE*3); 00347 SendCmd( CMD_SCALE); 00348 SendCmd( sx); 00349 SendCmd( sy); 00350 EndFunc( (FT_CMD_SIZE*3)); 00351 } 00352 ft_void_t FT800::Clock( ft_int16_t x, ft_int16_t y, ft_int16_t r, ft_uint16_t options, ft_uint16_t h, ft_uint16_t m, ft_uint16_t s, ft_uint16_t ms) 00353 { 00354 StartFunc( FT_CMD_SIZE*5); 00355 SendCmd( CMD_CLOCK); 00356 SendCmd( (((ft_uint32_t)y<<16)|(x & 0xffff))); 00357 SendCmd( (((ft_uint32_t)options<<16)|r)); 00358 SendCmd( (((ft_uint32_t)m<<16)|h)); 00359 SendCmd( (((ft_uint32_t)ms<<16)|s)); 00360 EndFunc( (FT_CMD_SIZE*5)); 00361 } 00362 00363 ft_void_t FT800::Gradient( ft_int16_t x0, ft_int16_t y0, ft_uint32_t rgb0, ft_int16_t x1, ft_int16_t y1, ft_uint32_t rgb1) 00364 { 00365 StartFunc( FT_CMD_SIZE*5); 00366 SendCmd( CMD_GRADIENT); 00367 SendCmd( (((ft_uint32_t)y0<<16)|(x0 & 0xffff))); 00368 SendCmd( rgb0); 00369 SendCmd( (((ft_uint32_t)y1<<16)|(x1 & 0xffff))); 00370 SendCmd( rgb1); 00371 EndFunc( (FT_CMD_SIZE*5)); 00372 } 00373 00374 ft_void_t FT800::SetMatrix( ) 00375 { 00376 StartFunc( FT_CMD_SIZE*1); 00377 SendCmd( CMD_SETMATRIX); 00378 EndFunc( (FT_CMD_SIZE*1)); 00379 } 00380 00381 ft_void_t FT800::Track( ft_int16_t x, ft_int16_t y, ft_int16_t w, ft_int16_t h, ft_int16_t tag) 00382 { 00383 StartFunc( FT_CMD_SIZE*4); 00384 SendCmd( CMD_TRACK); 00385 SendCmd( (((ft_uint32_t)y<<16)|(x & 0xffff))); 00386 SendCmd( (((ft_uint32_t)h<<16)|w)); 00387 SendCmd( tag); 00388 EndFunc( (FT_CMD_SIZE*4)); 00389 } 00390 00391 ft_void_t FT800::GetPtr( ft_uint32_t result) 00392 { 00393 StartFunc( FT_CMD_SIZE*2); 00394 SendCmd( CMD_GETPTR); 00395 SendCmd( result); 00396 EndFunc( (FT_CMD_SIZE*2)); 00397 } 00398 00399 ft_void_t FT800::Progress( ft_int16_t x, ft_int16_t y, ft_int16_t w, ft_int16_t h, ft_uint16_t options, ft_uint16_t val, ft_uint16_t range) 00400 { 00401 StartFunc( FT_CMD_SIZE*5); 00402 SendCmd( CMD_PROGRESS); 00403 SendCmd( (((ft_uint32_t)y<<16)|(x & 0xffff))); 00404 SendCmd( (((ft_uint32_t)h<<16)|w)); 00405 SendCmd( (((ft_uint32_t)val<<16)|options)); 00406 SendCmd( range); 00407 EndFunc( (FT_CMD_SIZE*5)); 00408 } 00409 00410 ft_void_t FT800::ColdStart( ) 00411 { 00412 StartFunc( FT_CMD_SIZE*1); 00413 SendCmd( CMD_COLDSTART); 00414 EndFunc( (FT_CMD_SIZE*1)); 00415 } 00416 00417 ft_void_t FT800::Keys( ft_int16_t x, ft_int16_t y, ft_int16_t w, ft_int16_t h, ft_int16_t font, ft_uint16_t options, const ft_char8_t* s) 00418 { 00419 StartFunc( FT_CMD_SIZE*4 + strlen(s) + 1); 00420 SendCmd( CMD_KEYS); 00421 SendCmd( (((ft_uint32_t)y<<16)|(x & 0xffff))); 00422 SendCmd( (((ft_uint32_t)h<<16)|w)); 00423 SendCmd( (((ft_uint32_t)options<<16)|font)); 00424 SendStr( s); 00425 EndFunc( (FT_CMD_SIZE*4 + strlen(s) + 1)); 00426 } 00427 00428 ft_void_t FT800::Dial( ft_int16_t x, ft_int16_t y, ft_int16_t r, ft_uint16_t options, ft_uint16_t val) 00429 { 00430 StartFunc( FT_CMD_SIZE*4); 00431 SendCmd( CMD_DIAL); 00432 SendCmd( (((ft_uint32_t)y<<16)|(x & 0xffff))); 00433 SendCmd( (((ft_uint32_t)options<<16)|r)); 00434 SendCmd( val); 00435 EndFunc( (FT_CMD_SIZE*4)); 00436 } 00437 00438 ft_void_t FT800::LoadImage( ft_uint32_t ptr, ft_uint32_t options) 00439 { 00440 StartFunc( FT_CMD_SIZE*3); 00441 SendCmd( CMD_LOADIMAGE); 00442 SendCmd( ptr); 00443 SendCmd( options); 00444 EndFunc( (FT_CMD_SIZE*3)); 00445 } 00446 00447 ft_void_t FT800::DLstart( ) 00448 { 00449 StartFunc( FT_CMD_SIZE*1); 00450 SendCmd( CMD_DLSTART); 00451 EndFunc( (FT_CMD_SIZE*1)); 00452 } 00453 00454 ft_void_t FT800::Snapshot( ft_uint32_t ptr) 00455 { 00456 StartFunc( FT_CMD_SIZE*2); 00457 SendCmd( CMD_SNAPSHOT); 00458 SendCmd( ptr); 00459 EndFunc( (FT_CMD_SIZE*2)); 00460 } 00461 00462 ft_void_t FT800::ScreenSaver( ) 00463 { 00464 StartFunc( FT_CMD_SIZE*1); 00465 SendCmd( CMD_SCREENSAVER); 00466 EndFunc( (FT_CMD_SIZE*1)); 00467 } 00468 00469 ft_void_t FT800::MemCrc( ft_uint32_t ptr, ft_uint32_t num, ft_uint32_t result) 00470 { 00471 StartFunc( FT_CMD_SIZE*4); 00472 SendCmd( CMD_MEMCRC); 00473 SendCmd( ptr); 00474 SendCmd( num); 00475 SendCmd( result); 00476 EndFunc( (FT_CMD_SIZE*4)); 00477 } 00478 00479 00480 ft_void_t FT800::DL(ft_uint32_t cmd) 00481 { 00482 WrCmd32(cmd); 00483 /* Increment the command index */ 00484 CmdBuffer_Index += FT_CMD_SIZE; 00485 } 00486 00487 ft_void_t FT800::WrDlCmd_Buffer(ft_uint32_t cmd) 00488 { 00489 Wr32((RAM_DL+DlBuffer_Index),cmd); 00490 /* Increment the command index */ 00491 DlBuffer_Index += FT_CMD_SIZE; 00492 } 00493 00494 ft_void_t FT800::Flush_DL_Buffer() 00495 { 00496 DlBuffer_Index = 0; 00497 00498 } 00499 00500 ft_void_t FT800::Flush_Co_Buffer() 00501 { 00502 CmdBuffer_Index = 0; 00503 } 00504 00505 00506 /* API to check the status of previous DLSWAP and perform DLSWAP of new DL */ 00507 /* Check for the status of previous DLSWAP and if still not done wait for few ms and check again */ 00508 ft_void_t FT800::DLSwap(ft_uint8_t DL_Swap_Type) 00509 { 00510 ft_uint8_t Swap_Type = DLSWAP_FRAME,Swap_Done = DLSWAP_FRAME; 00511 00512 if(DL_Swap_Type == DLSWAP_LINE) 00513 { 00514 Swap_Type = DLSWAP_LINE; 00515 } 00516 00517 /* Perform a new DL swap */ 00518 Wr8(REG_DLSWAP,Swap_Type); 00519 00520 /* Wait till the swap is done */ 00521 while(Swap_Done) 00522 { 00523 Swap_Done = Rd8(REG_DLSWAP); 00524 00525 if(DLSWAP_DONE != Swap_Done) 00526 { 00527 Sleep(10);//wait for 10ms 00528 } 00529 } 00530 } 00531 00532 00533 00534 /* Nothing beyond this */ 00535 00536 00537 00538
Generated on Tue Jul 12 2022 19:49:40 by
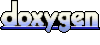