
Example of UART-DMA transfers taken form the npx cmsis driver libary
Embed:
(wiki syntax)
Show/hide line numbers
lpc17xx_pinsel.c
Go to the documentation of this file.
00001 /***********************************************************************//** 00002 * @file lpc17xx_pinsel.c 00003 * @brief Contains all functions support for Pin connect block firmware 00004 * library on LPC17xx 00005 * @version 2.0 00006 * @date 21. May. 2010 00007 * @author NXP MCU SW Application Team 00008 ************************************************************************** 00009 * Software that is described herein is for illustrative purposes only 00010 * which provides customers with programming information regarding the 00011 * products. This software is supplied "AS IS" without any warranties. 00012 * NXP Semiconductors assumes no responsibility or liability for the 00013 * use of the software, conveys no license or title under any patent, 00014 * copyright, or mask work right to the product. NXP Semiconductors 00015 * reserves the right to make changes in the software without 00016 * notification. NXP Semiconductors also make no representation or 00017 * warranty that such application will be suitable for the specified 00018 * use without further testing or modification. 00019 **********************************************************************/ 00020 00021 /* Peripheral group ----------------------------------------------------------- */ 00022 /** @addtogroup PINSEL 00023 * @{ 00024 */ 00025 00026 /* Includes ------------------------------------------------------------------- */ 00027 #include "lpc17xx_pinsel.h" 00028 00029 /* Public Functions ----------------------------------------------------------- */ 00030 00031 static void set_PinFunc ( uint8_t portnum, uint8_t pinnum, uint8_t funcnum); 00032 static void set_ResistorMode ( uint8_t portnum, uint8_t pinnum, uint8_t modenum); 00033 static void set_OpenDrainMode( uint8_t portnum, uint8_t pinnum, uint8_t modenum); 00034 00035 /*********************************************************************//** 00036 * @brief Setup the pin selection function 00037 * @param[in] portnum PORT number, 00038 * should be one of the following: 00039 * - PINSEL_PORT_0 : Port 0 00040 * - PINSEL_PORT_1 : Port 1 00041 * - PINSEL_PORT_2 : Port 2 00042 * - PINSEL_PORT_3 : Port 3 00043 * 00044 * @param[in] pinnum Pin number, 00045 * should be one of the following: 00046 - PINSEL_PIN_0 : Pin 0 00047 - PINSEL_PIN_1 : Pin 1 00048 - PINSEL_PIN_2 : Pin 2 00049 - PINSEL_PIN_3 : Pin 3 00050 - PINSEL_PIN_4 : Pin 4 00051 - PINSEL_PIN_5 : Pin 5 00052 - PINSEL_PIN_6 : Pin 6 00053 - PINSEL_PIN_7 : Pin 7 00054 - PINSEL_PIN_8 : Pin 8 00055 - PINSEL_PIN_9 : Pin 9 00056 - PINSEL_PIN_10 : Pin 10 00057 - PINSEL_PIN_11 : Pin 11 00058 - PINSEL_PIN_12 : Pin 12 00059 - PINSEL_PIN_13 : Pin 13 00060 - PINSEL_PIN_14 : Pin 14 00061 - PINSEL_PIN_15 : Pin 15 00062 - PINSEL_PIN_16 : Pin 16 00063 - PINSEL_PIN_17 : Pin 17 00064 - PINSEL_PIN_18 : Pin 18 00065 - PINSEL_PIN_19 : Pin 19 00066 - PINSEL_PIN_20 : Pin 20 00067 - PINSEL_PIN_21 : Pin 21 00068 - PINSEL_PIN_22 : Pin 22 00069 - PINSEL_PIN_23 : Pin 23 00070 - PINSEL_PIN_24 : Pin 24 00071 - PINSEL_PIN_25 : Pin 25 00072 - PINSEL_PIN_26 : Pin 26 00073 - PINSEL_PIN_27 : Pin 27 00074 - PINSEL_PIN_28 : Pin 28 00075 - PINSEL_PIN_29 : Pin 29 00076 - PINSEL_PIN_30 : Pin 30 00077 - PINSEL_PIN_31 : Pin 31 00078 00079 * @param[in] funcnum Function number, 00080 * should be one of the following: 00081 * - PINSEL_FUNC_0 : default function 00082 * - PINSEL_FUNC_1 : first alternate function 00083 * - PINSEL_FUNC_2 : second alternate function 00084 * - PINSEL_FUNC_3 : third alternate function 00085 * 00086 * @return None 00087 **********************************************************************/ 00088 static void set_PinFunc ( uint8_t portnum, uint8_t pinnum, uint8_t funcnum) 00089 { 00090 uint32_t pinnum_t = pinnum; 00091 uint32_t pinselreg_idx = 2 * portnum; 00092 uint32_t *pPinCon = (uint32_t *)&LPC_PINCON->PINSEL0; 00093 00094 if (pinnum_t >= 16) { 00095 pinnum_t -= 16; 00096 pinselreg_idx++; 00097 } 00098 *(uint32_t *)(pPinCon + pinselreg_idx) &= ~(0x03UL << (pinnum_t * 2)); 00099 *(uint32_t *)(pPinCon + pinselreg_idx) |= ((uint32_t)funcnum) << (pinnum_t * 2); 00100 } 00101 00102 /*********************************************************************//** 00103 * @brief Setup resistor mode for each pin 00104 * @param[in] portnum PORT number, 00105 * should be one of the following: 00106 * - PINSEL_PORT_0 : Port 0 00107 * - PINSEL_PORT_1 : Port 1 00108 * - PINSEL_PORT_2 : Port 2 00109 * - PINSEL_PORT_3 : Port 3 00110 * @param[in] pinnum Pin number, 00111 * should be one of the following: 00112 - PINSEL_PIN_0 : Pin 0 00113 - PINSEL_PIN_1 : Pin 1 00114 - PINSEL_PIN_2 : Pin 2 00115 - PINSEL_PIN_3 : Pin 3 00116 - PINSEL_PIN_4 : Pin 4 00117 - PINSEL_PIN_5 : Pin 5 00118 - PINSEL_PIN_6 : Pin 6 00119 - PINSEL_PIN_7 : Pin 7 00120 - PINSEL_PIN_8 : Pin 8 00121 - PINSEL_PIN_9 : Pin 9 00122 - PINSEL_PIN_10 : Pin 10 00123 - PINSEL_PIN_11 : Pin 11 00124 - PINSEL_PIN_12 : Pin 12 00125 - PINSEL_PIN_13 : Pin 13 00126 - PINSEL_PIN_14 : Pin 14 00127 - PINSEL_PIN_15 : Pin 15 00128 - PINSEL_PIN_16 : Pin 16 00129 - PINSEL_PIN_17 : Pin 17 00130 - PINSEL_PIN_18 : Pin 18 00131 - PINSEL_PIN_19 : Pin 19 00132 - PINSEL_PIN_20 : Pin 20 00133 - PINSEL_PIN_21 : Pin 21 00134 - PINSEL_PIN_22 : Pin 22 00135 - PINSEL_PIN_23 : Pin 23 00136 - PINSEL_PIN_24 : Pin 24 00137 - PINSEL_PIN_25 : Pin 25 00138 - PINSEL_PIN_26 : Pin 26 00139 - PINSEL_PIN_27 : Pin 27 00140 - PINSEL_PIN_28 : Pin 28 00141 - PINSEL_PIN_29 : Pin 29 00142 - PINSEL_PIN_30 : Pin 30 00143 - PINSEL_PIN_31 : Pin 31 00144 00145 * @param[in] modenum: Mode number, 00146 * should be one of the following: 00147 - PINSEL_PINMODE_PULLUP : Internal pull-up resistor 00148 - PINSEL_PINMODE_TRISTATE : Tri-state 00149 - PINSEL_PINMODE_PULLDOWN : Internal pull-down resistor 00150 00151 * @return None 00152 **********************************************************************/ 00153 void set_ResistorMode ( uint8_t portnum, uint8_t pinnum, uint8_t modenum) 00154 { 00155 uint32_t pinnum_t = pinnum; 00156 uint32_t pinmodereg_idx = 2 * portnum; 00157 uint32_t *pPinCon = (uint32_t *)&LPC_PINCON->PINMODE0; 00158 00159 if (pinnum_t >= 16) { 00160 pinnum_t -= 16; 00161 pinmodereg_idx++ ; 00162 } 00163 00164 *(uint32_t *)(pPinCon + pinmodereg_idx) &= ~(0x03UL << (pinnum_t * 2)); 00165 *(uint32_t *)(pPinCon + pinmodereg_idx) |= ((uint32_t)modenum) << (pinnum_t * 2); 00166 } 00167 00168 /*********************************************************************//** 00169 * @brief Setup Open drain mode for each pin 00170 * @param[in] portnum PORT number, 00171 * should be one of the following: 00172 * - PINSEL_PORT_0 : Port 0 00173 * - PINSEL_PORT_1 : Port 1 00174 * - PINSEL_PORT_2 : Port 2 00175 * - PINSEL_PORT_3 : Port 3 00176 * 00177 * @param[in] pinnum Pin number, 00178 * should be one of the following: 00179 - PINSEL_PIN_0 : Pin 0 00180 - PINSEL_PIN_1 : Pin 1 00181 - PINSEL_PIN_2 : Pin 2 00182 - PINSEL_PIN_3 : Pin 3 00183 - PINSEL_PIN_4 : Pin 4 00184 - PINSEL_PIN_5 : Pin 5 00185 - PINSEL_PIN_6 : Pin 6 00186 - PINSEL_PIN_7 : Pin 7 00187 - PINSEL_PIN_8 : Pin 8 00188 - PINSEL_PIN_9 : Pin 9 00189 - PINSEL_PIN_10 : Pin 10 00190 - PINSEL_PIN_11 : Pin 11 00191 - PINSEL_PIN_12 : Pin 12 00192 - PINSEL_PIN_13 : Pin 13 00193 - PINSEL_PIN_14 : Pin 14 00194 - PINSEL_PIN_15 : Pin 15 00195 - PINSEL_PIN_16 : Pin 16 00196 - PINSEL_PIN_17 : Pin 17 00197 - PINSEL_PIN_18 : Pin 18 00198 - PINSEL_PIN_19 : Pin 19 00199 - PINSEL_PIN_20 : Pin 20 00200 - PINSEL_PIN_21 : Pin 21 00201 - PINSEL_PIN_22 : Pin 22 00202 - PINSEL_PIN_23 : Pin 23 00203 - PINSEL_PIN_24 : Pin 24 00204 - PINSEL_PIN_25 : Pin 25 00205 - PINSEL_PIN_26 : Pin 26 00206 - PINSEL_PIN_27 : Pin 27 00207 - PINSEL_PIN_28 : Pin 28 00208 - PINSEL_PIN_29 : Pin 29 00209 - PINSEL_PIN_30 : Pin 30 00210 - PINSEL_PIN_31 : Pin 31 00211 00212 * @param[in] modenum Open drain mode number, 00213 * should be one of the following: 00214 * - PINSEL_PINMODE_NORMAL : Pin is in the normal (not open drain) mode 00215 * - PINSEL_PINMODE_OPENDRAIN : Pin is in the open drain mode 00216 * 00217 * @return None 00218 **********************************************************************/ 00219 void set_OpenDrainMode( uint8_t portnum, uint8_t pinnum, uint8_t modenum) 00220 { 00221 uint32_t *pPinCon = (uint32_t *)&LPC_PINCON->PINMODE_OD0; 00222 00223 if (modenum == PINSEL_PINMODE_OPENDRAIN){ 00224 *(uint32_t *)(pPinCon + portnum) |= (0x01UL << pinnum); 00225 } else { 00226 *(uint32_t *)(pPinCon + portnum) &= ~(0x01UL << pinnum); 00227 } 00228 } 00229 00230 /* End of Public Functions ---------------------------------------------------- */ 00231 00232 /* Public Functions ----------------------------------------------------------- */ 00233 /** @addtogroup PINSEL_Public_Functions 00234 * @{ 00235 */ 00236 /*********************************************************************//** 00237 * @brief Configure trace function 00238 * @param[in] NewState State of the Trace function configuration, 00239 * should be one of the following: 00240 * - ENABLE : Enable Trace Function 00241 * - DISABLE : Disable Trace Function 00242 * 00243 * @return None 00244 **********************************************************************/ 00245 void PINSEL_ConfigTraceFunc(FunctionalState NewState) 00246 { 00247 if (NewState == ENABLE) { 00248 LPC_PINCON->PINSEL10 |= (0x01UL << 3); 00249 } else if (NewState == DISABLE) { 00250 LPC_PINCON->PINSEL10 &= ~(0x01UL << 3); 00251 } 00252 } 00253 00254 /*********************************************************************//** 00255 * @brief Setup I2C0 pins 00256 * @param[in] i2cPinMode I2C pin mode, 00257 * should be one of the following: 00258 * - PINSEL_I2C_Normal_Mode : The standard drive mode 00259 * - PINSEL_I2C_Fast_Mode : Fast Mode Plus drive mode 00260 * 00261 * @param[in] filterSlewRateEnable should be: 00262 * - ENABLE: Enable filter and slew rate. 00263 * - DISABLE: Disable filter and slew rate. 00264 * 00265 * @return None 00266 **********************************************************************/ 00267 void PINSEL_SetI2C0Pins(uint8_t i2cPinMode, FunctionalState filterSlewRateEnable) 00268 { 00269 uint32_t regVal = 0; 00270 00271 if (i2cPinMode == PINSEL_I2C_Fast_Mode){ 00272 regVal = PINSEL_I2CPADCFG_SCLDRV0 | PINSEL_I2CPADCFG_SDADRV0; 00273 } 00274 00275 if (filterSlewRateEnable == DISABLE){ 00276 regVal = PINSEL_I2CPADCFG_SCLI2C0 | PINSEL_I2CPADCFG_SDAI2C0; 00277 } 00278 LPC_PINCON->I2CPADCFG = regVal; 00279 } 00280 00281 00282 /*********************************************************************//** 00283 * @brief Configure Pin corresponding to specified parameters passed 00284 * in the PinCfg 00285 * @param[in] PinCfg Pointer to a PINSEL_CFG_Type structure 00286 * that contains the configuration information for the 00287 * specified pin. 00288 * @return None 00289 **********************************************************************/ 00290 void PINSEL_ConfigPin(PINSEL_CFG_Type *PinCfg) 00291 { 00292 set_PinFunc(PinCfg->Portnum, PinCfg->Pinnum, PinCfg->Funcnum); 00293 set_ResistorMode(PinCfg->Portnum, PinCfg->Pinnum, PinCfg->Pinmode); 00294 set_OpenDrainMode(PinCfg->Portnum, PinCfg->Pinnum, PinCfg->OpenDrain); 00295 } 00296 00297 00298 /** 00299 * @} 00300 */ 00301 00302 /** 00303 * @} 00304 */ 00305 00306 /* --------------------------------- End Of File ------------------------------ */
Generated on Tue Jul 12 2022 10:57:55 by
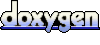