
Lab Checkoff
Dependencies: SDFileSystem TextLCD mbed-rtos mbed wave_player FATFileSystem
CommModule.h
00001 /********************************** 00002 * Author: Clifton Thomas 00003 * Date: 3/28/13 00004 * Institution: Georgia Tech 00005 * 00006 * Title: Communication Module 00007 * Class: ECE2035 00008 * Assignment: Project 2 00009 **********************************/ 00010 00011 #ifndef COMM_H 00012 #define COMM_H 00013 00014 #include <string.h> 00015 #include "mbed.h" 00016 00017 class commSerial: public Serial { 00018 public: 00019 //constructor 00020 commSerial(PinName tx, PinName rx, int baudrate): Serial(tx,rx) { 00021 Serial::baud(baudrate); 00022 } 00023 00024 //fcn to send data 00025 void sendData(char *str) { 00026 Serial::printf(str); 00027 } 00028 00029 //fcn to receive data 00030 void receiveData(char *buffer) { 00031 Serial::scanf("%s", buffer); 00032 } 00033 00034 }; 00035 00036 #endif
Generated on Fri Jul 15 2022 13:41:34 by
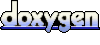