
Use serial port, not SD for datalogging.
Dependencies: MMA8451Q_tb SDFileSystem mbed
Fork of StepLogger by
main.cpp
00001 #include "mbed.h" 00002 #include "MMA8451Q_tb.h" 00003 #include "SDFileSystem.h" 00004 #include "math.h" 00005 00006 00007 #define MMA8451_I2C_ADDRESS (0x1d<<1) 00008 float acc_all[3]; 00009 DigitalOut pinout(PTD4); 00010 Timer timer; 00011 Serial pc(USBTX, USBRX); 00012 SDFileSystem sd(PTD2, PTD3, PTD1, PTD0, "sd"); // (mosi, miso, sclok, cs, name) 00013 00014 int main(void) { 00015 00016 //Initialize 00017 int now; //used for timestamping 00018 int counter = 0; //used for step counting 00019 int step_flag = 0; //used to sense transition into step 00020 float threshold = 1.43; //tunable in g's [m/s^2] 00021 float mag = 0; 00022 float x = 0; 00023 float y = 0; 00024 float z = 0; 00025 timer.start(); 00026 MMA8451Q acc(PTE25, PTE24, MMA8451_I2C_ADDRESS); 00027 printf("MMA8451 ID: %d\n", acc.getWhoAmI()); 00028 00029 //Open File 00030 //mkdir("/sd/mydir", 0777); 00031 //FILE *fp = fopen("/sd/mydir/sdtest.txt", "a"); 00032 //if(fp == NULL) { 00033 // error("Could not open file for write\n"); 00034 //} 00035 00036 while(1){ 00037 //for(int i=0; i<2000; i++) { 00038 pinout = !pinout; 00039 now = timer.read_ms(); 00040 acc.fastRead(&acc_all[0]); 00041 x = acc_all[0]; 00042 y = acc_all[1]; 00043 z = acc_all[2]; 00044 00045 mag = sqrt(x*x + y*y + z*z); 00046 //Print to file 00047 // fprintf(fp, "%d, %f,%f,%f\n",now, acc_all[0],acc_all[1],acc_all[2]); 00048 00049 //} 00050 //fclose(fp); 00051 // pc.printf("mag: %f\n", mag); 00052 if (mag > threshold){ 00053 if (step_flag == 0){ 00054 counter++; 00055 pc.printf("step_count:%d mag: %f\n", counter, mag); 00056 } 00057 step_flag = 1; 00058 } 00059 else { 00060 step_flag = 0; 00061 } 00062 00063 } 00064 }
Generated on Sun Jul 17 2022 08:27:18 by
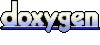