Embed:
(wiki syntax)
Show/hide line numbers
cnn_news.cpp
00001 00002 #include "mbed.h" 00003 #include "EthernetNetIf.h" 00004 #include "HTTPClient.h" 00005 #include "NokiaLCD.h" 00006 00007 NokiaLCD lcd(p5, p7, p8, p9, NokiaLCD::LCD6610); // mosi, sclk, cs, rst, type 00008 00009 EthernetNetIf eth; 00010 HTTPClient http; 00011 LocalFileSystem local("local"); 00012 HTTPResult result; 00013 bool completed = false; 00014 00015 void request_callback(HTTPResult r) 00016 { 00017 result = r; 00018 completed = true; 00019 } 00020 00021 int main() { 00022 00023 lcd.background(0x0000FF); 00024 lcd.cls(); 00025 00026 printf("Start\n"); 00027 00028 printf("Setting up...\n"); 00029 EthernetErr ethErr = eth.setup(); 00030 if(ethErr) 00031 { 00032 printf("Error %d in setup.\n", ethErr); 00033 return -1; 00034 } 00035 printf("Setup OK\n"); 00036 00037 HTTPStream stream; 00038 00039 int flag = 0; 00040 int count = 0; 00041 int pixel = 1; 00042 char c; 00043 char buffer[100]; 00044 char BigBuf[512 + 1] = {0}; 00045 stream.readNext((byte*)BigBuf, 512); //Point to buffer for the first read 00046 00047 HTTPResult r = http.get("http://rss.cnn.com/rss/cnn_topstories.rss", &stream, request_callback); //Load a very large page, such as the hackaday RSS feed 00048 FILE *fp = fopen("/local/out.txt", "w"); // Open "out.txt" on the local file system for writing 00049 while(!completed) 00050 { 00051 Net::poll(); //Polls the Networking stack 00052 if(stream.readable()) 00053 { 00054 BigBuf[stream.readLen()] = 0; //Transform this buffer in a zero-terminated char* string 00055 fprintf(fp,BigBuf); 00056 //Note: some servers do not like if you throttle them too much, so printf'ing during a request is generally bad practice 00057 stream.readNext((byte*)BigBuf, 512); //Buffer has been read, now we can put more data in it 00058 } 00059 } 00060 fclose(fp); 00061 fp = fopen( "/local/out.txt", "r"); 00062 if (fp==NULL) printf ("Error opening file"); 00063 else 00064 { 00065 do 00066 { 00067 c = fgetc (fp); 00068 if (c == '<') 00069 { 00070 c = fgetc(fp); 00071 if(c == 't') 00072 { 00073 c = fgetc(fp); 00074 if(c == 'i') 00075 { 00076 c = fgetc(fp); 00077 if(c == 't') 00078 { 00079 c = fgetc(fp); 00080 if( c == 'l') 00081 { 00082 c = fgetc(fp); 00083 if( c == 'e') 00084 { 00085 c = fgetc(fp); 00086 if(c == '>') 00087 { 00088 count++; 00089 if((count >=3) && (count <=5)) 00090 { 00091 if(count == 6) break; 00092 int i = 0; 00093 c = fgetc(fp); 00094 while(c != '<') 00095 { 00096 buffer[i] = c; 00097 i++; 00098 c = fgetc(fp); 00099 } 00100 buffer[i] = '\0'; 00101 lcd.locate(0,pixel); 00102 lcd.printf("%s",buffer); 00103 pixel += 5; 00104 } 00105 } 00106 } 00107 } 00108 } 00109 } 00110 } 00111 } 00112 }while (count <= 5); 00113 fclose (fp); 00114 } 00115 00116 if(result == HTTP_OK) 00117 { 00118 printf("Read completely\n"); 00119 } 00120 else 00121 { 00122 printf("Error %d\n", result); 00123 } 00124 00125 return 0; 00126 00127 } 00128 00129
Generated on Fri Jul 15 2022 09:37:33 by
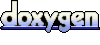