
Dependencies: EthernetNetIf mbed
TwitterExample.cpp
00001 /* 00002 Update: 21-06-2010 00003 The basic authentication service for twitter is going down at the end of the week. 00004 To continue using that program, the code has been updated to use http://supertweet.net which acts as an API proxy. 00005 Simply visit the website to setup your twitter account for this API. 00006 See: http://www.supertweet.net/about/documentation 00007 */ 00008 00009 #include "mbed.h" 00010 #include "EthernetNetIf.h" 00011 #include "HTTPClient.h" 00012 00013 EthernetNetIf eth; 00014 00015 int main() { 00016 00017 printf("Init\n"); 00018 00019 printf("\r\nSetting up...\r\n"); 00020 EthernetErr ethErr = eth.setup(); 00021 if(ethErr) 00022 { 00023 printf("Error %d in setup.\n", ethErr); 00024 return -1; 00025 } 00026 printf("\r\nSetup OK\r\n"); 00027 00028 HTTPClient twitter; 00029 00030 HTTPMap msg; 00031 msg["status"] = "I am tweeting from my mbed!"; //A good example of Key/Value pair use with Web APIs 00032 00033 twitter.basicAuth("myuser", "mypass"); //We use basic authentication, replace with you account's parameters 00034 00035 //No need to retieve data sent back by the server 00036 HTTPResult r = twitter.post("http://api.supertweet.net/1/statuses/update.xml", msg, NULL); 00037 if( r == HTTP_OK ) 00038 { 00039 printf("Tweet sent with success!\n"); 00040 } 00041 else 00042 { 00043 printf("Problem during tweeting, return code %d\n", r); 00044 } 00045 00046 return 0; 00047 00048 }
Generated on Tue Jul 19 2022 04:20:58 by
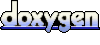