NetServices Stack source
Dependents: HelloWorld ServoInterfaceBoardExample1 4180_Lab4
PPPNetIf.cpp
00001 00002 /* 00003 Copyright (c) 2010 Donatien Garnier (donatiengar [at] gmail [dot] com) 00004 00005 Permission is hereby granted, free of charge, to any person obtaining a copy 00006 of this software and associated documentation files (the "Software"), to deal 00007 in the Software without restriction, including without limitation the rights 00008 to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00009 copies of the Software, and to permit persons to whom the Software is 00010 furnished to do so, subject to the following conditions: 00011 00012 The above copyright notice and this permission notice shall be included in 00013 all copies or substantial portions of the Software. 00014 00015 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00016 IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00017 FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00018 AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00019 LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00020 OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00021 THE SOFTWARE. 00022 */ 00023 00024 #include "PPPNetIf.h" 00025 #include "mbed.h" 00026 #include "ppp/ppp.h" 00027 #include "lwip/init.h" 00028 #include "lwip/sio.h" 00029 00030 #define __DEBUG 00031 #include "dbg/dbg.h" 00032 00033 #include "netCfg.h" 00034 #if NET_PPP 00035 00036 #define PPP_TIMEOUT 60000 00037 00038 #define BUF_SIZE 256 00039 00040 PPPNetIf::PPPNetIf(GPRSModem* pIf) : LwipNetIf(), m_pIf(pIf),/* m_open(false),*/ m_connected(false), m_status(PPP_DISCONNECTED), m_fd(0) //, m_id(0) 00041 { 00042 //FIXME: Check static refcount 00043 m_buf = new uint8_t[BUF_SIZE]; 00044 } 00045 00046 PPPNetIf::~PPPNetIf() 00047 { 00048 delete[] m_buf; 00049 } 00050 00051 #if 0 00052 PPPErr PPPNetIf::open(Serial* pSerial) 00053 { 00054 GPRSErr err = m_pIf->open(pSerial); 00055 if(err) 00056 return PPP_MODEM; 00057 m_open = true; 00058 #if 0 00059 m_id = sioMgr::registerSerialIf(this); 00060 if(!m_id) 00061 { 00062 close(); 00063 return PPP_CLOSED; 00064 } 00065 #endif 00066 return PPP_OK; 00067 } 00068 #endif 00069 00070 00071 PPPErr PPPNetIf::GPRSConnect(const char* apn, const char* userId, const char* password) //Connect using GPRS 00072 { 00073 LwipNetIf::init(); 00074 pppInit(); 00075 //TODO: Tell ATIf that we get ownership of the serial port 00076 00077 GPRSErr gprsErr; 00078 gprsErr = m_pIf->connect(apn); 00079 if(gprsErr) 00080 return PPP_NETWORK; 00081 00082 DBG("PPPNetIf: If Connected.\n"); 00083 00084 if( userId == NULL ) 00085 pppSetAuth(PPPAUTHTYPE_NONE, NULL, NULL); 00086 else 00087 pppSetAuth(PPPAUTHTYPE_PAP, userId, password); //TODO: Allow CHAP as well 00088 00089 DBG("PPPNetIf: Set Auth.\n"); 00090 00091 //wait(1.); 00092 00093 //m_pIf->flushBuffer(); //Flush buffer before passing serial port to PPP 00094 00095 m_status = PPP_CONNECTING; 00096 DBG("m_pIf = %p\n", m_pIf); 00097 int res = pppOverSerialOpen((void*)m_pIf, sPppCallback, (void*)this); 00098 DBG("PPP connected\n"); 00099 if(res<0) 00100 { 00101 disconnect(); 00102 return PPP_PROTOCOL; 00103 } 00104 00105 DBG("PPPNetIf: PPP Started with res = %d.\n", res); 00106 00107 m_fd = res; 00108 m_connected = true; 00109 Timer t; 00110 t.start(); 00111 while( m_status == PPP_CONNECTING ) //Wait for callback 00112 { 00113 poll(); 00114 if(t.read_ms()>PPP_TIMEOUT) 00115 { 00116 DBG("PPPNetIf: Timeout.\n"); 00117 disconnect(); 00118 return PPP_PROTOCOL; 00119 } 00120 } 00121 00122 DBG("PPPNetIf: Callback returned.\n"); 00123 00124 if( m_status == PPP_DISCONNECTED ) 00125 { 00126 disconnect(); 00127 return PPP_PROTOCOL; 00128 } 00129 00130 return PPP_OK; 00131 00132 } 00133 00134 PPPErr PPPNetIf::ATConnect(const char* number) //Connect using a "classic" voice modem or GSM 00135 { 00136 //TODO: IMPL 00137 return PPP_MODEM; 00138 } 00139 00140 PPPErr PPPNetIf::disconnect() 00141 { 00142 if(m_fd) 00143 pppClose(m_fd); //0 if ok, else should gen a WARN 00144 m_connected = false; 00145 00146 m_pIf->flushBuffer(); 00147 m_pIf->printf("+++\r\n"); 00148 wait(.5); 00149 m_pIf->flushBuffer(); 00150 00151 GPRSErr gprsErr; 00152 gprsErr = m_pIf->disconnect(); 00153 if(gprsErr) 00154 return PPP_NETWORK; 00155 00156 return PPP_OK; 00157 } 00158 00159 #if 0 00160 PPPErr PPPNetIf::close() 00161 { 00162 GPRSErr err = m_pIf->close(); 00163 if(err) 00164 return PPP_MODEM; 00165 m_open = false; 00166 return PPP_OK; 00167 } 00168 #endif 00169 00170 00171 #if 0 00172 //We have to use : 00173 00174 /** Pass received raw characters to PPPoS to be decoded. This function is 00175 * thread-safe and can be called from a dedicated RX-thread or from a main-loop. 00176 * 00177 * @param pd PPP descriptor index, returned by pppOpen() 00178 * @param data received data 00179 * @param len length of received data 00180 */ 00181 void 00182 pppos_input(int pd, u_char* data, int len) 00183 { 00184 pppInProc(&pppControl[pd].rx, data, len); 00185 } 00186 #endif 00187 00188 void PPPNetIf::poll() 00189 { 00190 if(!m_connected) 00191 return; 00192 LwipNetIf::poll(); 00193 //static u8_t buf[128]; 00194 int len; 00195 do 00196 { 00197 len = sio_tryread((sio_fd_t) m_pIf, m_buf, BUF_SIZE); 00198 if(len > 0) 00199 pppos_input(m_fd, m_buf, len); 00200 } while(len>0); 00201 } 00202 00203 //Link Callback 00204 void PPPNetIf::pppCallback(int errCode, void *arg) 00205 { 00206 switch ( errCode ) 00207 { 00208 //No error 00209 case PPPERR_NONE: 00210 { 00211 struct ppp_addrs* addrs = (struct ppp_addrs*) arg; 00212 m_ip = IpAddr(&(addrs->our_ipaddr)); //Set IP 00213 } 00214 m_status = PPP_CONNECTED; 00215 break; 00216 default: 00217 //Disconnected 00218 DBG("PPPNetIf: Callback errCode = %d.\n", errCode); 00219 m_status = PPP_DISCONNECTED; 00220 break; 00221 } 00222 } 00223 00224 #endif
Generated on Tue Jul 12 2022 11:52:58 by
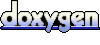