NetServices Stack source
Dependents: HelloWorld ServoInterfaceBoardExample1 4180_Lab4
SerialBuf.h
00001 00002 /* 00003 Copyright (c) 2010 Donatien Garnier (donatiengar [at] gmail [dot] com) 00004 00005 Permission is hereby granted, free of charge, to any person obtaining a copy 00006 of this software and associated documentation files (the "Software"), to deal 00007 in the Software without restriction, including without limitation the rights 00008 to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00009 copies of the Software, and to permit persons to whom the Software is 00010 furnished to do so, subject to the following conditions: 00011 00012 The above copyright notice and this permission notice shall be included in 00013 all copies or substantial portions of the Software. 00014 00015 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00016 IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00017 FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00018 AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00019 LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00020 OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00021 THE SOFTWARE. 00022 */ 00023 00024 #ifndef SERIALBUF_H 00025 #define SERIALBUF_H 00026 00027 #include "mbed.h" 00028 #include "netCfg.h" 00029 #if NET_USB_SERIAL 00030 #include "drv/serial/usb/UsbSerial.h" 00031 #endif 00032 00033 class SerialCircularBuf 00034 { 00035 public: 00036 SerialCircularBuf(int len); 00037 ~SerialCircularBuf(); 00038 00039 int room(); 00040 int len(); 00041 00042 void write(char c); 00043 char read(); 00044 00045 void setReadMode(bool readMode); //If true, keeps chars in buf when read, false by default 00046 void flushRead(); //Delete chars that have been read & return chars len (only useful with readMode = true) 00047 void resetRead(); //Go back to initial read position & return chars len (only useful with readMode = true) 00048 00049 private: 00050 char* m_buf; 00051 int m_len; 00052 00053 volatile char* m_pReadStart; 00054 volatile char* m_pRead; 00055 volatile char* m_pWrite; 00056 volatile bool m_readMode; 00057 }; 00058 00059 class SerialBuf 00060 { 00061 public: 00062 SerialBuf(int len); //Buffer length 00063 virtual ~SerialBuf(); 00064 00065 void attach(Serial* pSerial); 00066 void detach(); 00067 00068 #if NET_USB_SERIAL 00069 void attach(UsbSerial* pUsbSerial); 00070 #endif 00071 00072 //Really useful for debugging 00073 char getc(); 00074 void putc(char c); 00075 bool readable(); 00076 bool writeable(); 00077 /*protected:*/ 00078 void setReadMode(bool readMode); //If true, keeps chars in buf when read, false by default 00079 void flushRead(); //Delete chars that have been read & return chars len (only useful with readMode = true) 00080 void resetRead(); //Go back to initial read position & return chars len (only useful with readMode = true) 00081 00082 private: 00083 void onRxInterrupt(); //Callback from m_pSerial 00084 void onTxInterrupt(); //Callback from m_pSerial 00085 00086 SerialCircularBuf m_rxBuf; 00087 SerialCircularBuf m_txBuf; 00088 00089 Serial* m_pSerial; //Not owned 00090 00091 #if NET_USB_SERIAL 00092 //USB Serial Impl 00093 UsbSerial* m_pUsbSerial; //Not owned 00094 //Ticker m_usbTick; 00095 #endif 00096 }; 00097 00098 00099 #endif
Generated on Tue Jul 12 2022 11:52:58 by
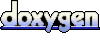