
Dependencies: EthernetNetIf mbed
HTTPClientStreamingExample.cpp
00001 #include "mbed.h" 00002 #include "EthernetNetIf.h" 00003 #include "HTTPClient.h" 00004 00005 EthernetNetIf eth; 00006 HTTPClient http; 00007 00008 HTTPResult result; 00009 bool completed = false; 00010 void request_callback(HTTPResult r) 00011 { 00012 result = r; 00013 completed = true; 00014 } 00015 00016 int main() { 00017 00018 printf("Start\n"); 00019 00020 printf("Setting up...\n"); 00021 EthernetErr ethErr = eth.setup(); 00022 if(ethErr) 00023 { 00024 printf("Error %d in setup.\n", ethErr); 00025 return -1; 00026 } 00027 printf("Setup OK\n"); 00028 00029 HTTPStream stream; 00030 00031 char BigBuf[512 + 1] = {0}; 00032 stream.readNext((byte*)BigBuf, 512); //Point to buffer for the first read 00033 //HTTPResult r = http.get("http://newsrss.bbc.co.uk/rss/newsonline_uk_edition/front_page/rss.xml", &stream, request_callback); //Load a very large page, such as the hackaday RSS feed 00034 //HTTPResult r = http.get("http://hackaday.com/feed/", &stream, request_callback); //Load a very large page, such as the hackaday RSS feed 00035 HTTPResult r = http.get("http://mbed.org/blog/feeds/entries/", &stream, request_callback); //Load a very large page 00036 00037 while(!completed) 00038 { 00039 Net::poll(); //Polls the Networking stack 00040 if(stream.readable()) 00041 { 00042 BigBuf[stream.readLen()] = 0; //Transform this buffer in a zero-terminated char* string 00043 printf("%s",BigBuf); //Display it while loading 00044 //Note: some servers do not like if you throttle them too much, so printf'ing during a request is generally bad practice 00045 stream.readNext((byte*)BigBuf, 512); //Buffer has been read, now we can put more data in it 00046 } 00047 } 00048 printf("\n--------------\n"); 00049 if(result == HTTP_OK) 00050 { 00051 printf("Read completely\n"); 00052 } 00053 else 00054 { 00055 printf("Error %d\n", result); 00056 } 00057 00058 while(1) 00059 { 00060 00061 } 00062 00063 return 0; 00064 00065 }
Generated on Sat Jul 16 2022 08:45:20 by
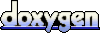