Debug library
Dependents: NetworkingCoreLib LwIPNetworking yeswecancoap lwip
dbg.cpp
00001 /* dbg.cpp */ 00002 /* Copyright (C) 2012 mbed.org, MIT License 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00005 * and associated documentation files (the "Software"), to deal in the Software without restriction, 00006 * including without limitation the rights to use, copy, modify, merge, publish, distribute, 00007 * sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is 00008 * furnished to do so, subject to the following conditions: 00009 * 00010 * The above copyright notice and this permission notice shall be included in all copies or 00011 * substantial portions of the Software. 00012 * 00013 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00014 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00015 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00016 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00017 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00018 */ 00019 00020 #include "dbg.h" 00021 00022 #include "mbed.h" 00023 #include "rtos.h" 00024 00025 #include <cstdio> 00026 #include <cstdarg> 00027 00028 using namespace std; 00029 00030 static Serial debug_pc(USBTX, USBRX); 00031 00032 Mutex* debug_printf_mutex; //Singleton runtime initialisation to avoid static initialisation chaos problems 00033 00034 static char debug_newline[3]; 00035 00036 void debug_init() 00037 { 00038 debug_set_newline("\n"); 00039 debug_pc.baud(115200); 00040 debug_printf_mutex = new Mutex(); 00041 printf("[START]\n"); 00042 fflush(stdout); 00043 } 00044 00045 void debug_set_newline(const char* newline) 00046 { 00047 strncpy( debug_newline, newline, 2 ); 00048 debug_newline[2] = '\0'; 00049 } 00050 00051 void debug(int level, const char* module, int line, const char* fmt, ...) 00052 { 00053 osStatus ret = debug_printf_mutex->lock(); 00054 if(ret != osOK) 00055 { 00056 /* 00057 printf("[FATAL] DBG Mutex failed!! (ret code = %02x - Mutex is @ %p)\n", ret, &debug_printf_mutex); 00058 error(""); 00059 while(1); //FATAL 00060 */ 00061 } 00062 switch(level) 00063 { 00064 default: 00065 case 1: 00066 printf("[ERROR]"); 00067 break; 00068 case 2: 00069 printf("[WARN]"); 00070 break; 00071 case 3: 00072 printf("[INFO]"); 00073 break; 00074 case 4: 00075 printf("[DBG]"); 00076 break; 00077 } 00078 00079 printf(" Module %s - Line %d: ", module, line); 00080 00081 va_list argp; 00082 00083 va_start(argp, fmt); 00084 vprintf(fmt, argp); 00085 va_end(argp); 00086 00087 printf(debug_newline); 00088 00089 fflush(stdout); 00090 00091 if(ret == osOK) 00092 { 00093 debug_printf_mutex->unlock(); 00094 } 00095 00096 } 00097 00098 void debug_error(const char* module, int line, int ret) 00099 { 00100 debug_printf_mutex->lock(); 00101 printf("[RC] Module %s - Line %d : Error %d\n", module, line, ret); 00102 fflush(stdout); 00103 debug_printf_mutex->unlock(); 00104 } 00105 00106 void debug_exact(const char* fmt, ...) 00107 { 00108 osStatus ret = debug_printf_mutex->lock(); 00109 if(ret != osOK) 00110 { 00111 /* 00112 printf("[FATAL] DBG Mutex failed!! (ret code = %02x - Mutex is @ %p)\n", ret, &debug_printf_mutex); 00113 error(""); 00114 while(1); //FATAL 00115 */ 00116 } 00117 00118 va_list argp; 00119 00120 va_start(argp, fmt); 00121 vprintf(fmt, argp); 00122 va_end(argp); 00123 00124 if(ret == osOK) 00125 { 00126 debug_printf_mutex->unlock(); 00127 } 00128 }
Generated on Thu Jul 14 2022 07:10:37 by
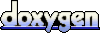