Library for controlling an MCP4725 "12-Bit Digital-to-Analog Converter with EEPROM Memory". There is an accompanying test suite program "MCP_4725_Library_Test" that can be used to test this library.
Dependents: IGGE_Power MCP4725_Library_Test MCP4725 SinWave ... more
MCP4725 Class Reference
#include <mcp4725.h>
Public Types | |
enum | PowerMode { Normal = 0, PowerDown1k = 1, PowerDown100k = 2, PowerDown500k = 3 } |
The device supports two types of power modes: normal and power-down. More... | |
enum | BusFrequency { Standard100kHz, Fast400kHz, HighSpeed3_4Mhz } |
The device supports 3 different I2C bus frequencies. More... | |
Public Member Functions | |
MCP4725 (PinName sda, PinName scl, BusFrequency bus_frequency, int device_address_bits) | |
Create an mcp4725 I2C interface. | |
int | read (enum PowerMode *mode, enum PowerMode *mode_eeprom, int *dac_value, int *dac_value_eeprom, bool *eeprom_write_in_progress) |
Read the contents of the dac register, the contents of eeprom, and if an eeprom write is currently active. | |
Protected Attributes | |
I2C | _i2c_interface |
mbed I2C interface driver. | |
int | _device_address |
The full i2c device address. |
Detailed Description
MCP4725 class.
Used for interfacing with a mcp4725 12-Bit Digital-to-Analog Converter. To convert between the 12bit dac_value and Vout, use the following formula: dac_value = (Vout*4096/Vref), where Vout is the desired output analog voltage, Vref is the voltage connected to the Vdd pin of the device. Typically Vdd will be 3.3volts.
Note: There is an accompanying test suite program "MCP_4725_Library_Test" that can be used to test this library.
Definition at line 17 of file mcp4725.h.
Member Enumeration Documentation
enum BusFrequency |
enum PowerMode |
The device supports two types of power modes: normal and power-down.
In normal mode the device operates a normal digital to analog conversion. In power-down mode all digitial to analog conversion is stopped, resulting in the device using less power (typically 60nA). Also, in power down mode Vout will be pulled to ground using either a 1k, 100k or 500k ohm internal resistors.
- Enumerator:
Constructor & Destructor Documentation
MCP4725 | ( | PinName | sda, |
PinName | scl, | ||
BusFrequency | bus_frequency, | ||
int | device_address_bits | ||
) |
Create an mcp4725 I2C interface.
- Parameters:
-
sda I2C data line pin scl I2C clock line pin bus_frequency the frequency at which the I2C bus is running. device_address_bits The 3bit address bits of the device.
Definition at line 4 of file mcp4725.cpp.
Member Function Documentation
int read | ( | enum PowerMode * | mode, |
enum PowerMode * | mode_eeprom, | ||
int * | dac_value, | ||
int * | dac_value_eeprom, | ||
bool * | eeprom_write_in_progress | ||
) |
Read the contents of the dac register, the contents of eeprom, and if an eeprom write is currently active.
- Parameters:
-
mode Pointer to variable to store the current power mode. mode_eeprom Pointer to variable to store the power mode as is stored in eeprom. dac_value Pointer to variable to store the current dac value. dac_value_eeprom Pointer to variable to store the dac value as is stored in eeprom. eeprom_write_in_progress Pointer to variable to store the current eeprom write status.
- Returns:
- 0 on success, non-0 on failure
Definition at line 27 of file mcp4725.cpp.
Field Documentation
int _device_address [protected] |
I2C _i2c_interface [protected] |
Generated on Thu Jul 14 2022 00:26:39 by
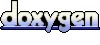