mbed-os for GR-LYCHEE
Dependents: mbed-os-example-blinky-gr-lychee GR-Boads_Camera_sample GR-Boards_Audio_Recoder GR-Boads_Camera_DisplayApp ... more
test_mem.c
00001 #include "test_mem.h" 00002 00003 #include "lwip/mem.h" 00004 #include "lwip/stats.h" 00005 00006 #if !LWIP_STATS || !MEM_STATS 00007 #error "This tests needs MEM-statistics enabled" 00008 #endif 00009 #if LWIP_DNS 00010 #error "This test needs DNS turned off (as it mallocs on init)" 00011 #endif 00012 00013 /* Setups/teardown functions */ 00014 00015 static void 00016 mem_setup(void) 00017 { 00018 } 00019 00020 static void 00021 mem_teardown(void) 00022 { 00023 } 00024 00025 00026 /* Test functions */ 00027 00028 /** Call mem_malloc, mem_free and mem_trim and check stats */ 00029 START_TEST(test_mem_one) 00030 { 00031 #define SIZE1 16 00032 #define SIZE1_2 12 00033 #define SIZE2 16 00034 void *p1, *p2; 00035 mem_size_t s1, s2; 00036 LWIP_UNUSED_ARG(_i); 00037 00038 fail_unless(lwip_stats.mem.used == 0); 00039 00040 p1 = mem_malloc(SIZE1); 00041 fail_unless(p1 != NULL); 00042 fail_unless(lwip_stats.mem.used >= SIZE1); 00043 s1 = lwip_stats.mem.used; 00044 00045 p2 = mem_malloc(SIZE2); 00046 fail_unless(p2 != NULL); 00047 fail_unless(lwip_stats.mem.used >= SIZE2 + s1); 00048 s2 = lwip_stats.mem.used; 00049 00050 mem_trim(p1, SIZE1_2); 00051 00052 mem_free(p2); 00053 fail_unless(lwip_stats.mem.used <= s2 - SIZE2); 00054 00055 mem_free(p1); 00056 fail_unless(lwip_stats.mem.used == 0); 00057 } 00058 END_TEST 00059 00060 static void malloc_keep_x(int x, int num, int size, int freestep) 00061 { 00062 int i; 00063 void* p[16]; 00064 LWIP_ASSERT("invalid size", size >= 0 && size < (mem_size_t)-1); 00065 memset(p, 0, sizeof(p)); 00066 for(i = 0; i < num && i < 16; i++) { 00067 p[i] = mem_malloc((mem_size_t)size); 00068 fail_unless(p[i] != NULL); 00069 } 00070 for(i = 0; i < num && i < 16; i += freestep) { 00071 if (i == x) { 00072 continue; 00073 } 00074 mem_free(p[i]); 00075 p[i] = NULL; 00076 } 00077 for(i = 0; i < num && i < 16; i++) { 00078 if (i == x) { 00079 continue; 00080 } 00081 if (p[i] != NULL) { 00082 mem_free(p[i]); 00083 p[i] = NULL; 00084 } 00085 } 00086 fail_unless(p[x] != NULL); 00087 mem_free(p[x]); 00088 } 00089 00090 START_TEST(test_mem_random) 00091 { 00092 const int num = 16; 00093 int x; 00094 int size; 00095 int freestep; 00096 LWIP_UNUSED_ARG(_i); 00097 00098 fail_unless(lwip_stats.mem.used == 0); 00099 00100 for (x = 0; x < num; x++) { 00101 for (size = 1; size < 32; size++) { 00102 for (freestep = 1; freestep <= 3; freestep++) { 00103 fail_unless(lwip_stats.mem.used == 0); 00104 malloc_keep_x(x, num, size, freestep); 00105 fail_unless(lwip_stats.mem.used == 0); 00106 } 00107 } 00108 } 00109 } 00110 END_TEST 00111 00112 /** Create the suite including all tests for this module */ 00113 Suite * 00114 mem_suite(void) 00115 { 00116 testfunc tests[] = { 00117 TESTFUNC(test_mem_one), 00118 TESTFUNC(test_mem_random) 00119 }; 00120 return create_suite("MEM", tests, sizeof(tests)/sizeof(testfunc), mem_setup, mem_teardown); 00121 }
Generated on Tue Jul 12 2022 11:02:33 by
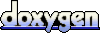