mbed-os for GR-LYCHEE
Dependents: mbed-os-example-blinky-gr-lychee GR-Boads_Camera_sample GR-Boards_Audio_Recoder GR-Boads_Camera_DisplayApp ... more
lwip_upap.c
00001 /* 00002 * upap.c - User/Password Authentication Protocol. 00003 * 00004 * Copyright (c) 1984-2000 Carnegie Mellon University. All rights reserved. 00005 * 00006 * Redistribution and use in source and binary forms, with or without 00007 * modification, are permitted provided that the following conditions 00008 * are met: 00009 * 00010 * 1. Redistributions of source code must retain the above copyright 00011 * notice, this list of conditions and the following disclaimer. 00012 * 00013 * 2. Redistributions in binary form must reproduce the above copyright 00014 * notice, this list of conditions and the following disclaimer in 00015 * the documentation and/or other materials provided with the 00016 * distribution. 00017 * 00018 * 3. The name "Carnegie Mellon University" must not be used to 00019 * endorse or promote products derived from this software without 00020 * prior written permission. For permission or any legal 00021 * details, please contact 00022 * Office of Technology Transfer 00023 * Carnegie Mellon University 00024 * 5000 Forbes Avenue 00025 * Pittsburgh, PA 15213-3890 00026 * (412) 268-4387, fax: (412) 268-7395 00027 * tech-transfer@andrew.cmu.edu 00028 * 00029 * 4. Redistributions of any form whatsoever must retain the following 00030 * acknowledgment: 00031 * "This product includes software developed by Computing Services 00032 * at Carnegie Mellon University (http://www.cmu.edu/computing/)." 00033 * 00034 * CARNEGIE MELLON UNIVERSITY DISCLAIMS ALL WARRANTIES WITH REGARD TO 00035 * THIS SOFTWARE, INCLUDING ALL IMPLIED WARRANTIES OF MERCHANTABILITY 00036 * AND FITNESS, IN NO EVENT SHALL CARNEGIE MELLON UNIVERSITY BE LIABLE 00037 * FOR ANY SPECIAL, INDIRECT OR CONSEQUENTIAL DAMAGES OR ANY DAMAGES 00038 * WHATSOEVER RESULTING FROM LOSS OF USE, DATA OR PROFITS, WHETHER IN 00039 * AN ACTION OF CONTRACT, NEGLIGENCE OR OTHER TORTIOUS ACTION, ARISING 00040 * OUT OF OR IN CONNECTION WITH THE USE OR PERFORMANCE OF THIS SOFTWARE. 00041 */ 00042 00043 #include "netif/ppp/ppp_opts.h" 00044 #if PPP_SUPPORT && PAP_SUPPORT /* don't build if not configured for use in lwipopts.h */ 00045 00046 /* 00047 * @todo: 00048 */ 00049 00050 #if 0 /* UNUSED */ 00051 #include <stdio.h> 00052 #include <string.h> 00053 #endif /* UNUSED */ 00054 00055 #include "netif/ppp/ppp_impl.h" 00056 00057 #include "netif/ppp/upap.h" 00058 00059 #if PPP_OPTIONS 00060 /* 00061 * Command-line options. 00062 */ 00063 static option_t pap_option_list[] = { 00064 { "hide-password", o_bool, &hide_password, 00065 "Don't output passwords to log", OPT_PRIO | 1 }, 00066 { "show-password", o_bool, &hide_password, 00067 "Show password string in debug log messages", OPT_PRIOSUB | 0 }, 00068 00069 { "pap-restart", o_int, &upap[0].us_timeouttime, 00070 "Set retransmit timeout for PAP", OPT_PRIO }, 00071 { "pap-max-authreq", o_int, &upap[0].us_maxtransmits, 00072 "Set max number of transmissions for auth-reqs", OPT_PRIO }, 00073 { "pap-timeout", o_int, &upap[0].us_reqtimeout, 00074 "Set time limit for peer PAP authentication", OPT_PRIO }, 00075 00076 { NULL } 00077 }; 00078 #endif /* PPP_OPTIONS */ 00079 00080 /* 00081 * Protocol entry points. 00082 */ 00083 static void upap_init(ppp_pcb *pcb); 00084 static void upap_lowerup(ppp_pcb *pcb); 00085 static void upap_lowerdown(ppp_pcb *pcb); 00086 static void upap_input(ppp_pcb *pcb, u_char *inpacket, int l); 00087 static void upap_protrej(ppp_pcb *pcb); 00088 #if PRINTPKT_SUPPORT 00089 static int upap_printpkt(const u_char *p, int plen, void (*printer) (void *, const char *, ...), void *arg); 00090 #endif /* PRINTPKT_SUPPORT */ 00091 00092 const struct protent pap_protent = { 00093 PPP_PAP, 00094 upap_init, 00095 upap_input, 00096 upap_protrej, 00097 upap_lowerup, 00098 upap_lowerdown, 00099 NULL, 00100 NULL, 00101 #if PRINTPKT_SUPPORT 00102 upap_printpkt, 00103 #endif /* PRINTPKT_SUPPORT */ 00104 #if PPP_DATAINPUT 00105 NULL, 00106 #endif /* PPP_DATAINPUT */ 00107 #if PRINTPKT_SUPPORT 00108 "PAP", 00109 NULL, 00110 #endif /* PRINTPKT_SUPPORT */ 00111 #if PPP_OPTIONS 00112 pap_option_list, 00113 NULL, 00114 #endif /* PPP_OPTIONS */ 00115 #if DEMAND_SUPPORT 00116 NULL, 00117 NULL 00118 #endif /* DEMAND_SUPPORT */ 00119 }; 00120 00121 static void upap_timeout(void *arg); 00122 #if PPP_SERVER 00123 static void upap_reqtimeout(void *arg); 00124 static void upap_rauthreq(ppp_pcb *pcb, u_char *inp, int id, int len); 00125 #endif /* PPP_SERVER */ 00126 static void upap_rauthack(ppp_pcb *pcb, u_char *inp, int id, int len); 00127 static void upap_rauthnak(ppp_pcb *pcb, u_char *inp, int id, int len); 00128 static void upap_sauthreq(ppp_pcb *pcb); 00129 #if PPP_SERVER 00130 static void upap_sresp(ppp_pcb *pcb, u_char code, u_char id, const char *msg, int msglen); 00131 #endif /* PPP_SERVER */ 00132 00133 00134 /* 00135 * upap_init - Initialize a UPAP unit. 00136 */ 00137 static void upap_init(ppp_pcb *pcb) { 00138 pcb->upap.us_user = NULL; 00139 pcb->upap.us_userlen = 0; 00140 pcb->upap.us_passwd = NULL; 00141 pcb->upap.us_passwdlen = 0; 00142 pcb->upap.us_clientstate = UPAPCS_INITIAL; 00143 #if PPP_SERVER 00144 pcb->upap.us_serverstate = UPAPSS_INITIAL; 00145 #endif /* PPP_SERVER */ 00146 pcb->upap.us_id = 0; 00147 } 00148 00149 00150 /* 00151 * upap_authwithpeer - Authenticate us with our peer (start client). 00152 * 00153 * Set new state and send authenticate's. 00154 */ 00155 void upap_authwithpeer(ppp_pcb *pcb, const char *user, const char *password) { 00156 00157 if(!user || !password) 00158 return; 00159 00160 /* Save the username and password we're given */ 00161 pcb->upap.us_user = user; 00162 pcb->upap.us_userlen = LWIP_MIN(strlen(user), 0xff); 00163 pcb->upap.us_passwd = password; 00164 pcb->upap.us_passwdlen = LWIP_MIN(strlen(password), 0xff); 00165 pcb->upap.us_transmits = 0; 00166 00167 /* Lower layer up yet? */ 00168 if (pcb->upap.us_clientstate == UPAPCS_INITIAL || 00169 pcb->upap.us_clientstate == UPAPCS_PENDING) { 00170 pcb->upap.us_clientstate = UPAPCS_PENDING; 00171 return; 00172 } 00173 00174 upap_sauthreq(pcb); /* Start protocol */ 00175 } 00176 00177 #if PPP_SERVER 00178 /* 00179 * upap_authpeer - Authenticate our peer (start server). 00180 * 00181 * Set new state. 00182 */ 00183 void upap_authpeer(ppp_pcb *pcb) { 00184 00185 /* Lower layer up yet? */ 00186 if (pcb->upap.us_serverstate == UPAPSS_INITIAL || 00187 pcb->upap.us_serverstate == UPAPSS_PENDING) { 00188 pcb->upap.us_serverstate = UPAPSS_PENDING; 00189 return; 00190 } 00191 00192 pcb->upap.us_serverstate = UPAPSS_LISTEN; 00193 if (pcb->settings.pap_req_timeout > 0) 00194 TIMEOUT(upap_reqtimeout, pcb, pcb->settings.pap_req_timeout); 00195 } 00196 #endif /* PPP_SERVER */ 00197 00198 /* 00199 * upap_timeout - Retransmission timer for sending auth-reqs expired. 00200 */ 00201 static void upap_timeout(void *arg) { 00202 ppp_pcb *pcb = (ppp_pcb*)arg; 00203 00204 if (pcb->upap.us_clientstate != UPAPCS_AUTHREQ) 00205 return; 00206 00207 if (pcb->upap.us_transmits >= pcb->settings.pap_max_transmits) { 00208 /* give up in disgust */ 00209 ppp_error("No response to PAP authenticate-requests"); 00210 pcb->upap.us_clientstate = UPAPCS_BADAUTH; 00211 auth_withpeer_fail(pcb, PPP_PAP); 00212 return; 00213 } 00214 00215 upap_sauthreq(pcb); /* Send Authenticate-Request */ 00216 } 00217 00218 00219 #if PPP_SERVER 00220 /* 00221 * upap_reqtimeout - Give up waiting for the peer to send an auth-req. 00222 */ 00223 static void upap_reqtimeout(void *arg) { 00224 ppp_pcb *pcb = (ppp_pcb*)arg; 00225 00226 if (pcb->upap.us_serverstate != UPAPSS_LISTEN) 00227 return; /* huh?? */ 00228 00229 auth_peer_fail(pcb, PPP_PAP); 00230 pcb->upap.us_serverstate = UPAPSS_BADAUTH; 00231 } 00232 #endif /* PPP_SERVER */ 00233 00234 00235 /* 00236 * upap_lowerup - The lower layer is up. 00237 * 00238 * Start authenticating if pending. 00239 */ 00240 static void upap_lowerup(ppp_pcb *pcb) { 00241 00242 if (pcb->upap.us_clientstate == UPAPCS_INITIAL) 00243 pcb->upap.us_clientstate = UPAPCS_CLOSED; 00244 else if (pcb->upap.us_clientstate == UPAPCS_PENDING) { 00245 upap_sauthreq(pcb); /* send an auth-request */ 00246 } 00247 00248 #if PPP_SERVER 00249 if (pcb->upap.us_serverstate == UPAPSS_INITIAL) 00250 pcb->upap.us_serverstate = UPAPSS_CLOSED; 00251 else if (pcb->upap.us_serverstate == UPAPSS_PENDING) { 00252 pcb->upap.us_serverstate = UPAPSS_LISTEN; 00253 if (pcb->settings.pap_req_timeout > 0) 00254 TIMEOUT(upap_reqtimeout, pcb, pcb->settings.pap_req_timeout); 00255 } 00256 #endif /* PPP_SERVER */ 00257 } 00258 00259 00260 /* 00261 * upap_lowerdown - The lower layer is down. 00262 * 00263 * Cancel all timeouts. 00264 */ 00265 static void upap_lowerdown(ppp_pcb *pcb) { 00266 00267 if (pcb->upap.us_clientstate == UPAPCS_AUTHREQ) /* Timeout pending? */ 00268 UNTIMEOUT(upap_timeout, pcb); /* Cancel timeout */ 00269 #if PPP_SERVER 00270 if (pcb->upap.us_serverstate == UPAPSS_LISTEN && pcb->settings.pap_req_timeout > 0) 00271 UNTIMEOUT(upap_reqtimeout, pcb); 00272 #endif /* PPP_SERVER */ 00273 00274 pcb->upap.us_clientstate = UPAPCS_INITIAL; 00275 #if PPP_SERVER 00276 pcb->upap.us_serverstate = UPAPSS_INITIAL; 00277 #endif /* PPP_SERVER */ 00278 } 00279 00280 00281 /* 00282 * upap_protrej - Peer doesn't speak this protocol. 00283 * 00284 * This shouldn't happen. In any case, pretend lower layer went down. 00285 */ 00286 static void upap_protrej(ppp_pcb *pcb) { 00287 00288 if (pcb->upap.us_clientstate == UPAPCS_AUTHREQ) { 00289 ppp_error("PAP authentication failed due to protocol-reject"); 00290 auth_withpeer_fail(pcb, PPP_PAP); 00291 } 00292 #if PPP_SERVER 00293 if (pcb->upap.us_serverstate == UPAPSS_LISTEN) { 00294 ppp_error("PAP authentication of peer failed (protocol-reject)"); 00295 auth_peer_fail(pcb, PPP_PAP); 00296 } 00297 #endif /* PPP_SERVER */ 00298 upap_lowerdown(pcb); 00299 } 00300 00301 00302 /* 00303 * upap_input - Input UPAP packet. 00304 */ 00305 static void upap_input(ppp_pcb *pcb, u_char *inpacket, int l) { 00306 u_char *inp; 00307 u_char code, id; 00308 int len; 00309 00310 /* 00311 * Parse header (code, id and length). 00312 * If packet too short, drop it. 00313 */ 00314 inp = inpacket; 00315 if (l < UPAP_HEADERLEN) { 00316 UPAPDEBUG(("pap_input: rcvd short header.")); 00317 return; 00318 } 00319 GETCHAR(code, inp); 00320 GETCHAR(id, inp); 00321 GETSHORT(len, inp); 00322 if (len < UPAP_HEADERLEN) { 00323 UPAPDEBUG(("pap_input: rcvd illegal length.")); 00324 return; 00325 } 00326 if (len > l) { 00327 UPAPDEBUG(("pap_input: rcvd short packet.")); 00328 return; 00329 } 00330 len -= UPAP_HEADERLEN; 00331 00332 /* 00333 * Action depends on code. 00334 */ 00335 switch (code) { 00336 case UPAP_AUTHREQ: 00337 #if PPP_SERVER 00338 upap_rauthreq(pcb, inp, id, len); 00339 #endif /* PPP_SERVER */ 00340 break; 00341 00342 case UPAP_AUTHACK: 00343 upap_rauthack(pcb, inp, id, len); 00344 break; 00345 00346 case UPAP_AUTHNAK: 00347 upap_rauthnak(pcb, inp, id, len); 00348 break; 00349 00350 default: /* XXX Need code reject */ 00351 break; 00352 } 00353 } 00354 00355 #if PPP_SERVER 00356 /* 00357 * upap_rauth - Receive Authenticate. 00358 */ 00359 static void upap_rauthreq(ppp_pcb *pcb, u_char *inp, int id, int len) { 00360 u_char ruserlen, rpasswdlen; 00361 char *ruser; 00362 char *rpasswd; 00363 char rhostname[256]; 00364 int retcode; 00365 const char *msg; 00366 int msglen; 00367 00368 if (pcb->upap.us_serverstate < UPAPSS_LISTEN) 00369 return; 00370 00371 /* 00372 * If we receive a duplicate authenticate-request, we are 00373 * supposed to return the same status as for the first request. 00374 */ 00375 if (pcb->upap.us_serverstate == UPAPSS_OPEN) { 00376 upap_sresp(pcb, UPAP_AUTHACK, id, "", 0); /* return auth-ack */ 00377 return; 00378 } 00379 if (pcb->upap.us_serverstate == UPAPSS_BADAUTH) { 00380 upap_sresp(pcb, UPAP_AUTHNAK, id, "", 0); /* return auth-nak */ 00381 return; 00382 } 00383 00384 /* 00385 * Parse user/passwd. 00386 */ 00387 if (len < 1) { 00388 UPAPDEBUG(("pap_rauth: rcvd short packet.")); 00389 return; 00390 } 00391 GETCHAR(ruserlen, inp); 00392 len -= sizeof (u_char) + ruserlen + sizeof (u_char); 00393 if (len < 0) { 00394 UPAPDEBUG(("pap_rauth: rcvd short packet.")); 00395 return; 00396 } 00397 ruser = (char *) inp; 00398 INCPTR(ruserlen, inp); 00399 GETCHAR(rpasswdlen, inp); 00400 if (len < rpasswdlen) { 00401 UPAPDEBUG(("pap_rauth: rcvd short packet.")); 00402 return; 00403 } 00404 00405 rpasswd = (char *) inp; 00406 00407 /* 00408 * Check the username and password given. 00409 */ 00410 retcode = UPAP_AUTHNAK; 00411 if (auth_check_passwd(pcb, ruser, ruserlen, rpasswd, rpasswdlen, &msg, &msglen)) { 00412 retcode = UPAP_AUTHACK; 00413 } 00414 BZERO(rpasswd, rpasswdlen); 00415 00416 #if 0 /* UNUSED */ 00417 /* 00418 * Check remote number authorization. A plugin may have filled in 00419 * the remote number or added an allowed number, and rather than 00420 * return an authenticate failure, is leaving it for us to verify. 00421 */ 00422 if (retcode == UPAP_AUTHACK) { 00423 if (!auth_number()) { 00424 /* We do not want to leak info about the pap result. */ 00425 retcode = UPAP_AUTHNAK; /* XXX exit value will be "wrong" */ 00426 warn("calling number %q is not authorized", remote_number); 00427 } 00428 } 00429 00430 msglen = strlen(msg); 00431 if (msglen > 255) 00432 msglen = 255; 00433 #endif /* UNUSED */ 00434 00435 upap_sresp(pcb, retcode, id, msg, msglen); 00436 00437 /* Null terminate and clean remote name. */ 00438 ppp_slprintf(rhostname, sizeof(rhostname), "%.*v", ruserlen, ruser); 00439 00440 if (retcode == UPAP_AUTHACK) { 00441 pcb->upap.us_serverstate = UPAPSS_OPEN; 00442 ppp_notice("PAP peer authentication succeeded for %q", rhostname); 00443 auth_peer_success(pcb, PPP_PAP, 0, ruser, ruserlen); 00444 } else { 00445 pcb->upap.us_serverstate = UPAPSS_BADAUTH; 00446 ppp_warn("PAP peer authentication failed for %q", rhostname); 00447 auth_peer_fail(pcb, PPP_PAP); 00448 } 00449 00450 if (pcb->settings.pap_req_timeout > 0) 00451 UNTIMEOUT(upap_reqtimeout, pcb); 00452 } 00453 #endif /* PPP_SERVER */ 00454 00455 /* 00456 * upap_rauthack - Receive Authenticate-Ack. 00457 */ 00458 static void upap_rauthack(ppp_pcb *pcb, u_char *inp, int id, int len) { 00459 u_char msglen; 00460 char *msg; 00461 LWIP_UNUSED_ARG(id); 00462 00463 if (pcb->upap.us_clientstate != UPAPCS_AUTHREQ) /* XXX */ 00464 return; 00465 00466 /* 00467 * Parse message. 00468 */ 00469 if (len < 1) { 00470 UPAPDEBUG(("pap_rauthack: ignoring missing msg-length.")); 00471 } else { 00472 GETCHAR(msglen, inp); 00473 if (msglen > 0) { 00474 len -= sizeof (u_char); 00475 if (len < msglen) { 00476 UPAPDEBUG(("pap_rauthack: rcvd short packet.")); 00477 return; 00478 } 00479 msg = (char *) inp; 00480 PRINTMSG(msg, msglen); 00481 } 00482 } 00483 00484 pcb->upap.us_clientstate = UPAPCS_OPEN; 00485 00486 auth_withpeer_success(pcb, PPP_PAP, 0); 00487 } 00488 00489 00490 /* 00491 * upap_rauthnak - Receive Authenticate-Nak. 00492 */ 00493 static void upap_rauthnak(ppp_pcb *pcb, u_char *inp, int id, int len) { 00494 u_char msglen; 00495 char *msg; 00496 LWIP_UNUSED_ARG(id); 00497 00498 if (pcb->upap.us_clientstate != UPAPCS_AUTHREQ) /* XXX */ 00499 return; 00500 00501 /* 00502 * Parse message. 00503 */ 00504 if (len < 1) { 00505 UPAPDEBUG(("pap_rauthnak: ignoring missing msg-length.")); 00506 } else { 00507 GETCHAR(msglen, inp); 00508 if (msglen > 0) { 00509 len -= sizeof (u_char); 00510 if (len < msglen) { 00511 UPAPDEBUG(("pap_rauthnak: rcvd short packet.")); 00512 return; 00513 } 00514 msg = (char *) inp; 00515 PRINTMSG(msg, msglen); 00516 } 00517 } 00518 00519 pcb->upap.us_clientstate = UPAPCS_BADAUTH; 00520 00521 ppp_error("PAP authentication failed"); 00522 auth_withpeer_fail(pcb, PPP_PAP); 00523 } 00524 00525 00526 /* 00527 * upap_sauthreq - Send an Authenticate-Request. 00528 */ 00529 static void upap_sauthreq(ppp_pcb *pcb) { 00530 struct pbuf *p; 00531 u_char *outp; 00532 int outlen; 00533 00534 outlen = UPAP_HEADERLEN + 2 * sizeof (u_char) + 00535 pcb->upap.us_userlen + pcb->upap.us_passwdlen; 00536 p = pbuf_alloc(PBUF_RAW, (u16_t)(PPP_HDRLEN +outlen), PPP_CTRL_PBUF_TYPE); 00537 if(NULL == p) 00538 return; 00539 if(p->tot_len != p->len) { 00540 pbuf_free(p); 00541 return; 00542 } 00543 00544 outp = (u_char*)p->payload; 00545 MAKEHEADER(outp, PPP_PAP); 00546 00547 PUTCHAR(UPAP_AUTHREQ, outp); 00548 PUTCHAR(++pcb->upap.us_id, outp); 00549 PUTSHORT(outlen, outp); 00550 PUTCHAR(pcb->upap.us_userlen, outp); 00551 MEMCPY(outp, pcb->upap.us_user, pcb->upap.us_userlen); 00552 INCPTR(pcb->upap.us_userlen, outp); 00553 PUTCHAR(pcb->upap.us_passwdlen, outp); 00554 MEMCPY(outp, pcb->upap.us_passwd, pcb->upap.us_passwdlen); 00555 00556 ppp_write(pcb, p); 00557 00558 TIMEOUT(upap_timeout, pcb, pcb->settings.pap_timeout_time); 00559 ++pcb->upap.us_transmits; 00560 pcb->upap.us_clientstate = UPAPCS_AUTHREQ; 00561 } 00562 00563 #if PPP_SERVER 00564 /* 00565 * upap_sresp - Send a response (ack or nak). 00566 */ 00567 static void upap_sresp(ppp_pcb *pcb, u_char code, u_char id, const char *msg, int msglen) { 00568 struct pbuf *p; 00569 u_char *outp; 00570 int outlen; 00571 00572 outlen = UPAP_HEADERLEN + sizeof (u_char) + msglen; 00573 p = pbuf_alloc(PBUF_RAW, (u16_t)(PPP_HDRLEN +outlen), PPP_CTRL_PBUF_TYPE); 00574 if(NULL == p) 00575 return; 00576 if(p->tot_len != p->len) { 00577 pbuf_free(p); 00578 return; 00579 } 00580 00581 outp = (u_char*)p->payload; 00582 MAKEHEADER(outp, PPP_PAP); 00583 00584 PUTCHAR(code, outp); 00585 PUTCHAR(id, outp); 00586 PUTSHORT(outlen, outp); 00587 PUTCHAR(msglen, outp); 00588 MEMCPY(outp, msg, msglen); 00589 00590 ppp_write(pcb, p); 00591 } 00592 #endif /* PPP_SERVER */ 00593 00594 #if PRINTPKT_SUPPORT 00595 /* 00596 * upap_printpkt - print the contents of a PAP packet. 00597 */ 00598 static const char* const upap_codenames[] = { 00599 "AuthReq", "AuthAck", "AuthNak" 00600 }; 00601 00602 static int upap_printpkt(const u_char *p, int plen, void (*printer) (void *, const char *, ...), void *arg) { 00603 int code, id, len; 00604 int mlen, ulen, wlen; 00605 const u_char *user, *pwd, *msg; 00606 const u_char *pstart; 00607 00608 if (plen < UPAP_HEADERLEN) 00609 return 0; 00610 pstart = p; 00611 GETCHAR(code, p); 00612 GETCHAR(id, p); 00613 GETSHORT(len, p); 00614 if (len < UPAP_HEADERLEN || len > plen) 00615 return 0; 00616 00617 if (code >= 1 && code <= (int)LWIP_ARRAYSIZE(upap_codenames)) 00618 printer(arg, " %s", upap_codenames[code-1]); 00619 else 00620 printer(arg, " code=0x%x", code); 00621 printer(arg, " id=0x%x", id); 00622 len -= UPAP_HEADERLEN; 00623 switch (code) { 00624 case UPAP_AUTHREQ: 00625 if (len < 1) 00626 break; 00627 ulen = p[0]; 00628 if (len < ulen + 2) 00629 break; 00630 wlen = p[ulen + 1]; 00631 if (len < ulen + wlen + 2) 00632 break; 00633 user = (const u_char *) (p + 1); 00634 pwd = (const u_char *) (p + ulen + 2); 00635 p += ulen + wlen + 2; 00636 len -= ulen + wlen + 2; 00637 printer(arg, " user="); 00638 ppp_print_string(user, ulen, printer, arg); 00639 printer(arg, " password="); 00640 /* FIXME: require ppp_pcb struct as printpkt() argument */ 00641 #if 0 00642 if (!pcb->settings.hide_password) 00643 #endif 00644 ppp_print_string(pwd, wlen, printer, arg); 00645 #if 0 00646 else 00647 printer(arg, "<hidden>"); 00648 #endif 00649 break; 00650 case UPAP_AUTHACK: 00651 case UPAP_AUTHNAK: 00652 if (len < 1) 00653 break; 00654 mlen = p[0]; 00655 if (len < mlen + 1) 00656 break; 00657 msg = (const u_char *) (p + 1); 00658 p += mlen + 1; 00659 len -= mlen + 1; 00660 printer(arg, " "); 00661 ppp_print_string(msg, mlen, printer, arg); 00662 break; 00663 default: 00664 break; 00665 } 00666 00667 /* print the rest of the bytes in the packet */ 00668 for (; len > 0; --len) { 00669 GETCHAR(code, p); 00670 printer(arg, " %.2x", code); 00671 } 00672 00673 return p - pstart; 00674 } 00675 #endif /* PRINTPKT_SUPPORT */ 00676 00677 #endif /* PPP_SUPPORT && PAP_SUPPORT */
Generated on Tue Jul 12 2022 11:02:28 by
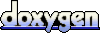