mbed-os for GR-LYCHEE
Dependents: mbed-os-example-blinky-gr-lychee GR-Boads_Camera_sample GR-Boards_Audio_Recoder GR-Boads_Camera_DisplayApp ... more
__init__.py
00001 """ 00002 mbed SDK 00003 Copyright (c) 2014-2016 ARM Limited 00004 00005 Licensed under the Apache License, Version 2.0 (the "License"); 00006 you may not use this file except in compliance with the License. 00007 You may obtain a copy of the License at 00008 00009 http://www.apache.org/licenses/LICENSE-2.0 00010 00011 Unless required by applicable law or agreed to in writing, software 00012 distributed under the License is distributed on an "AS IS" BASIS, 00013 WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00014 See the License for the specific language governing permissions and 00015 limitations under the License. 00016 """ 00017 from os.path import split,splitext, basename 00018 00019 from tools.export.exporters import Exporter 00020 00021 class Folder: 00022 def __init__(self, name): 00023 self.name = name 00024 self.children = [] 00025 00026 def contains(self, folderName): 00027 for child in self.children: 00028 if child.name == folderName: 00029 return True 00030 return False 00031 00032 def __str__(self): 00033 retval = self.name + " " 00034 if len(self.children) > 0: 00035 retval += "[ " 00036 for child in self.children: 00037 retval += child.__str__() 00038 retval += " ]" 00039 00040 return retval 00041 00042 def findChild(self, folderName): 00043 for child in self.children: 00044 if child.name == folderName: 00045 return child 00046 return None 00047 00048 def addChild(self, folderName): 00049 if folderName == '': 00050 return None 00051 00052 if not self.contains(folderName): 00053 self.children.append(Folder(folderName)) 00054 00055 return self.findChild(folderName) 00056 00057 class SimplicityV3(Exporter ): 00058 NAME = 'SimplicityV3' 00059 TOOLCHAIN = 'GCC_ARM' 00060 00061 TARGETS = [ 00062 'EFM32GG_STK3700', 00063 'EFM32ZG_STK3200', 00064 'EFM32LG_STK3600', 00065 'EFM32WG_STK3800', 00066 'EFM32HG_STK3400', 00067 'EFM32PG_STK3401' 00068 ] 00069 00070 PARTS = { 00071 'EFM32GG_STK3700': 'com.silabs.mcu.si32.efm32.efm32gg.efm32gg990f1024', 00072 'EFM32ZG_STK3200': 'com.silabs.mcu.si32.efm32.efm32zg.efm32zg222f32', 00073 'EFM32LG_STK3600': 'com.silabs.mcu.si32.efm32.efm32lg.efm32lg990f256', 00074 'EFM32WG_STK3800': 'com.silabs.mcu.si32.efm32.efm32wg.efm32wg990f256', 00075 'EFM32HG_STK3400': 'com.silabs.mcu.si32.efm32.efm32hg.efm32hg322f64', 00076 'EFM32PG_STK3401': 'com.silabs.mcu.si32.efm32.efm32pg1b.efm32pg1b200f256gm48' 00077 } 00078 00079 KITS = { 00080 'EFM32GG_STK3700': 'com.silabs.kit.si32.efm32.efm32gg.stk3700', 00081 'EFM32ZG_STK3200': 'com.silabs.kit.si32.efm32.efm32zg.stk3200', 00082 'EFM32LG_STK3600': 'com.silabs.kit.si32.efm32.efm32lg.stk3600', 00083 'EFM32WG_STK3800': 'com.silabs.kit.si32.efm32.efm32wg.stk3800', 00084 'EFM32HG_STK3400': 'com.silabs.kit.si32.efm32.efm32hg.slstk3400a', 00085 'EFM32PG_STK3401': 'com.silabs.kit.si32.efm32.efm32pg.slstk3401a' 00086 } 00087 00088 FILE_TYPES = { 00089 'c_sources':'1', 00090 'cpp_sources':'1', 00091 's_sources':'1' 00092 } 00093 00094 EXCLUDED_LIBS = [ 00095 'm', 00096 'c', 00097 'gcc', 00098 'nosys', 00099 'supc++', 00100 'stdc++' 00101 ] 00102 00103 DOT_IN_RELATIVE_PATH = False 00104 00105 MBED_CONFIG_HEADER_SUPPORTED = True 00106 00107 orderedPaths = Folder("Root") 00108 00109 def check_and_add_path(self, path): 00110 levels = path.split('/') 00111 base = self.orderedPaths 00112 for level in levels: 00113 if base.contains(level): 00114 base = base.findChild(level) 00115 else: 00116 base.addChild(level) 00117 base = base.findChild(level) 00118 00119 00120 def generate(self): 00121 # "make" wants Unix paths 00122 self.resources.win_to_unix() 00123 00124 main_files = [] 00125 00126 EXCLUDED_LIBS = [ 00127 'm', 00128 'c', 00129 'gcc', 00130 'nosys', 00131 'supc++', 00132 'stdc++' 00133 ] 00134 00135 for r_type in ['s_sources', 'c_sources', 'cpp_sources']: 00136 r = getattr(self.resources, r_type) 00137 if r: 00138 for source in r: 00139 self.check_and_add_path(split(source)[0]) 00140 00141 if not ('/' in source): 00142 main_files.append(source) 00143 00144 libraries = [] 00145 for lib in self.resources.libraries: 00146 l, _ = splitext(basename(lib)) 00147 if l[3:] not in EXCLUDED_LIBS: 00148 libraries.append(l[3:]) 00149 00150 defines = [] 00151 for define in self.toolchain.get_symbols(): 00152 if '=' in define: 00153 keyval = define.split('=') 00154 defines.append( (keyval[0], keyval[1]) ) 00155 else: 00156 defines.append( (define, '') ) 00157 00158 self.check_and_add_path(split(self.resources.linker_script)[0]) 00159 00160 ctx = { 00161 'name': self.project_name, 00162 'main_files': main_files, 00163 'recursiveFolders': self.orderedPaths, 00164 'object_files': self.resources.objects, 00165 'include_paths': self.resources.inc_dirs, 00166 'library_paths': self.resources.lib_dirs, 00167 'linker_script': self.resources.linker_script, 00168 'libraries': libraries, 00169 'defines': defines, 00170 'part': self.PARTS[self.target], 00171 'kit': self.KITS[self.target], 00172 'loopcount': 0 00173 } 00174 ctx.update(self.flags) 00175 00176 ## Strip main folder from include paths because ssproj is not capable of handling it 00177 if '.' in ctx['include_paths']: 00178 ctx['include_paths'].remove('.') 00179 ctx['include_root'] = True 00180 00181 ''' 00182 Suppress print statements 00183 print('\n') 00184 print(self.target) 00185 print('\n') 00186 print(ctx) 00187 print('\n') 00188 print(self.orderedPaths) 00189 for path in self.orderedPaths.children: 00190 print(path.name + "\n") 00191 for bpath in path.children: 00192 print("\t" + bpath.name + "\n") 00193 ''' 00194 00195 self.gen_file('simplicity/slsproj.tmpl', ctx, '%s.slsproj' % self.project_name)
Generated on Tue Jul 12 2022 11:02:21 by
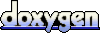