mbed-os for GR-LYCHEE
Dependents: mbed-os-example-blinky-gr-lychee GR-Boads_Camera_sample GR-Boards_Audio_Recoder GR-Boads_Camera_DisplayApp ... more
cfstore_svm.cpp
00001 /* 00002 * mbed Microcontroller Library 00003 * Copyright (c) 2006-2016 ARM Limited 00004 * 00005 * Licensed under the Apache License, Version 2.0 (the "License"); 00006 * you may not use this file except in compliance with the License. 00007 * You may obtain a copy of the License at 00008 * 00009 * http://www.apache.org/licenses/LICENSE-2.0 00010 * 00011 * Unless required by applicable law or agreed to in writing, software 00012 * distributed under the License is distributed on an "AS IS" BASIS, 00013 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00014 * See the License for the specific language governing permissions and 00015 * limitations under the License. 00016 * 00017 */ 00018 00019 #include <stdint.h> 00020 #include <Driver_Common.h> 00021 #include "storage_volume_manager.h" 00022 #include "cfstore_config.h" 00023 #include "cfstore_debug.h" 00024 #include "cfstore_svm.h" 00025 00026 /** @file cfstore_svm.cpp 00027 * 00028 * This module is provides a C wrapper to the C++ storage-volume-manager.h API, 00029 * so it can be called by the C-HAL implementation configuration_store.c 00030 */ 00031 00032 #ifndef CFSTORE_SVM_VOL_01_START_OFFSET 00033 #define CFSTORE_SVM_VOL_01_START_OFFSET 0x80000UL 00034 #endif 00035 00036 #ifndef CFSTORE_SVM_VOL_01_SIZE 00037 #define CFSTORE_SVM_VOL_01_SIZE 0x80000UL 00038 #endif 00039 00040 #ifdef CFSTORE_CONFIG_BACKEND_FLASH_ENABLED 00041 extern ARM_DRIVER_STORAGE ARM_Driver_Storage_MTD_K64F; 00042 static ARM_DRIVER_STORAGE *cfstore_svm_storage_drv = &ARM_Driver_Storage_MTD_K64F; 00043 00044 /* the storage volume manager instance used to generate virtual mtd descriptors */ 00045 StorageVolumeManager volumeManager; 00046 00047 /* used only for the initialization of the volume-manager. */ 00048 static void cfstore_svm_volume_manager_initialize_callback(int32_t status) 00049 { 00050 CFSTORE_FENTRYLOG("%s: with status %d" , __func__, (int) status); 00051 } 00052 00053 static void cfstore_svm_journal_mtc_callback(int32_t status, ARM_STORAGE_OPERATION operation) 00054 { 00055 CFSTORE_FENTRYLOG("%s: operation %d with status %d" , __func__, (int) operation, (int) status); 00056 } 00057 00058 int32_t cfstore_svm_init(struct _ARM_DRIVER_STORAGE *storage_mtd) 00059 { 00060 int32_t ret = ARM_DRIVER_OK; 00061 00062 CFSTORE_FENTRYLOG("%s:entered\n", __func__); 00063 ret = volumeManager.initialize(cfstore_svm_storage_drv, cfstore_svm_volume_manager_initialize_callback); 00064 if(ret < ARM_DRIVER_OK) { 00065 CFSTORE_ERRLOG("%s:debug: volume-manager::initialize() failed for storage_mtd=%p (ret=%d)", __func__, storage_mtd, (int) ret); 00066 return ret; 00067 } 00068 ret = volumeManager.addVolume_C(CFSTORE_SVM_VOL_01_START_OFFSET, CFSTORE_SVM_VOL_01_SIZE, storage_mtd); 00069 if(ret < ARM_DRIVER_OK) { 00070 CFSTORE_ERRLOG("%s:debug: volume-manager::addVolume_C() failed for storage_mtd=%p (ret=%d)", __func__, storage_mtd, (int) ret); 00071 return ret; 00072 } 00073 ret = storage_mtd->Initialize(cfstore_svm_journal_mtc_callback); 00074 if(ret < ARM_DRIVER_OK) { 00075 CFSTORE_ERRLOG("%s:debug: storage_mtd->initialize() failed for storage_mtd=%p (ret=%d)", __func__, storage_mtd, (int) ret); 00076 return ret; 00077 } 00078 return ret; 00079 } 00080 00081 #endif /* CFSTORE_CONFIG_BACKEND_FLASH_ENABLED */ 00082
Generated on Tue Jul 12 2022 11:02:22 by
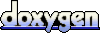